


What are the reusability and extensibility advantages of C++ functions in large GUI projects?
C functions provide reusability and extensibility benefits in large GUI projects by encapsulating code and allowing code reuse: Reusability: Functions that encapsulate common tasks can eliminate redundancy and reduce maintenance effort. Extensibility: Supports adding new features or modifying existing functions to extend the project GUI as needed. Practical example: Using C functions in large GUI projects brings significant reusability, scalability, and maintainability benefits.
The advantages of reusability and scalability of C functions in large GUI projects
In the development of large GUI projects, Reusability and extensibility are critical. C functions play a vital role in achieving these goals by encapsulating code and allowing code reuse.
Code Reuse
C functions allow code reuse, thereby eliminating redundancy and reducing maintenance effort. By encapsulating common tasks in functions, you can easily use these features throughout your project without having to rewrite your code.
// 定义一个绘制按钮的函数 void drawButton(const sf::Vector2f& position, const sf::Color& color) { sf::RectangleShape button(sf::Vector2f(100.f, 50.f)); button.setPosition(position); button.setFillColor(color); window.draw(button); } // 不同的类可以使用 drawButton 函数来创建按钮 class MyClass1 { public: void createButton() { drawButton({100.f, 100.f}, sf::Color::Red); } }; class MyClass2 { public: void createButton() { drawButton({200.f, 200.f}, sf::Color::Green); } };
Extensibility
C functions support extensibility, allowing you to easily add new functionality to your project. You can extend your project's GUI as needed by creating new functions or modifying existing ones.
// 修改 drawButton 函数以绘制带文本的按钮 void drawButton(const sf::Vector2f& position, const sf::Color& color, const std::string& text) { // 绘制按钮 drawButton(position, color); // 添加文本 sf::Text label; label.setString(text); label.setPosition(position + sf::Vector2f(5.f, 5.f)); window.draw(label); } // 现在可以创建带文本的按钮了 class MyClass3 { public: void createButton() { drawButton({300.f, 300.f}, sf::Color::Blue, "Click Me"); } };
Practical case
In a large graphical user interface project, the use of C functions can bring significant benefits:
- Reusability: Reusable functions for creating common GUI elements such as buttons, menus, and windows. This saves development time and reduces code duplication.
- Extensibility: Easily add new functionality to your project or modify existing functionality by creating new functions or modifying existing ones. This enables projects to quickly adapt to changing needs.
- Maintainability:Encapsulating code in functions makes maintenance easier, as individual functions can be easily modified or replaced without affecting other parts of the code.
Overall, C functions provide the powerful advantages of reusability and extensibility in large GUI projects, thereby simplifying the development, maintenance, and extension process.
The above is the detailed content of What are the reusability and extensibility advantages of C++ functions in large GUI projects?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
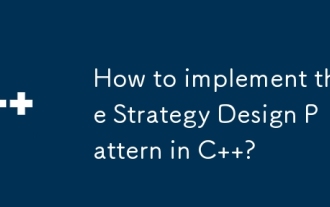
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
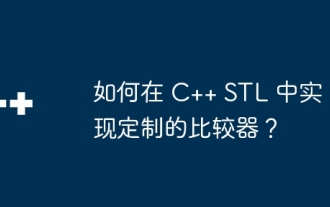
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
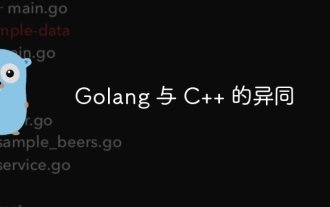
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
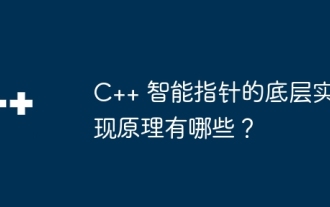
C++ smart pointers implement automatic memory management through pointer counting, destructors, and virtual function tables. The pointer count keeps track of the number of references, and when the number of references drops to 0, the destructor releases the original pointer. Virtual function tables enable polymorphism, allowing specific behaviors to be implemented for different types of smart pointers.
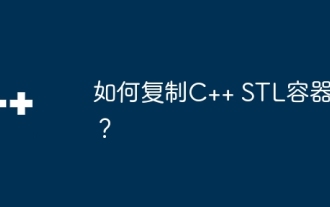
There are three ways to copy a C++ STL container: Use the copy constructor to copy the contents of the container to a new container. Use the assignment operator to copy the contents of the container to the target container. Use the std::copy algorithm to copy the elements in the container.
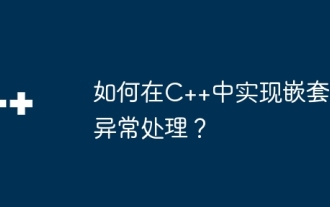
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
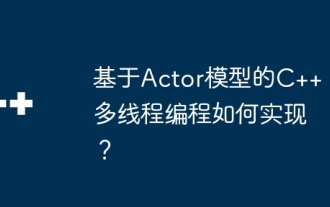
C++ multi-threaded programming implementation based on the Actor model: Create an Actor class that represents an independent entity. Set the message queue where messages are stored. Defines the method for an Actor to receive and process messages from the queue. Create Actor objects and start threads to run them. Send messages to Actors via the message queue. This approach provides high concurrency, scalability, and isolation, making it ideal for applications that need to handle large numbers of parallel tasks.
