


Detailed explanation of C++ function templates: a powerful tool for concept-oriented programming
Function templates provide powerful tools for C through concept-oriented programming to achieve universal functions and type safety. Syntax: template <typename T> T foo(T a, T b) Practical combat: Generic maximum function, supporting different types of parameters. Concept Programming Constraints: Imposing type constraints, such as Comparable or Arithmetic, that restrict parameter types. Advantages: code reusability, type safety, scalability.
Detailed explanation of C function templates: a powerful tool for concept-oriented programming
Function templates are a powerful tool in C that allow the creation of functions that can be parameterized by different types. function. Through concept-oriented programming, we can impose constraints on the parameter types of function templates, thereby achieving type safety and code reusability.
Function template syntax
The syntax of function template is as follows:
template <typename T> T foo(T a, T b) { // ... }
Where:
template <typename T>
means this is a function template,T
is a type parameter.T foo(T a, T b)
is the function prototype, whereT
represents the function’s parameter and return value types and type parametersT
same.
Practical case: Maximum function
Consider a function that obtains the maximum of two values:
int max(int a, int b) { return a > b ? a : b; } double max(double a, double b) { return a > b ? a : b; }
We can use function templates to generalize this function Typing:
template <typename T> T max(T a, T b) { return a > b ? a : b; }
Now, we can use the same max
function to find the maximum value of any type, including integers, floating point numbers, and even custom types:
int x = max(2, 5); // x == 5 double y = max(3.14, 9.81); // y == 9.81
Conceptual programming constraints
Concept-oriented programming allows us to apply constraints on function templates, thereby limiting the possibility of parameter types. The C standard library provides many concepts, such as:
- ##Comparable
: type has
<,
>,
<=,
>=operator.
- Arithmetic
: Type has arithmetic operators (
,
-,
*,
/).
- Integral
: The type is an integer type.
template <typename T> requires Comparable<T> T max(T a, T b) { // ... }
max function parameters.
- Code reusability:One-time functions can be created for different types of parameters .
- Type safety: Concept-oriented programming allows the imposition of type constraints to prevent accidental use of incompatible types.
- Extensibility: New function templates can be easily created to support new types or concepts.
The above is the detailed content of Detailed explanation of C++ function templates: a powerful tool for concept-oriented programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


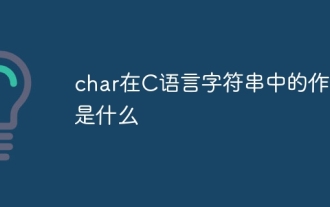
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
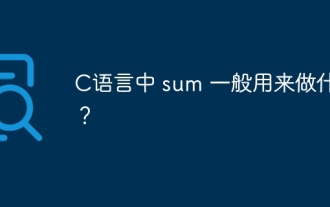
There is no function named "sum" in the C language standard library. "sum" is usually defined by programmers or provided in specific libraries, and its functionality depends on the specific implementation. Common scenarios are summing for arrays, and can also be used in other data structures, such as linked lists. In addition, "sum" is also used in fields such as image processing and statistical analysis. An excellent "sum" function should have good readability, robustness and efficiency.
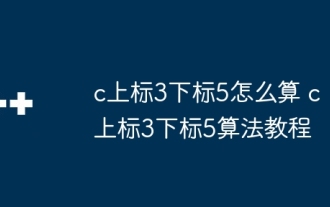
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
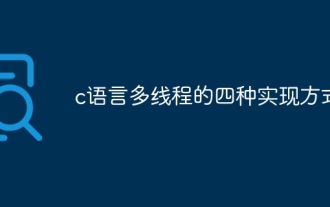
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
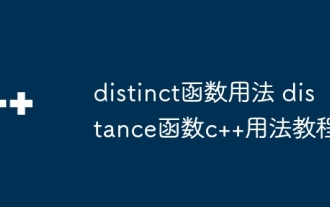
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
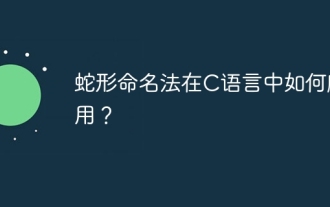
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
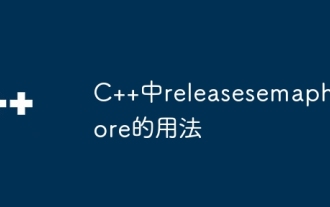
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
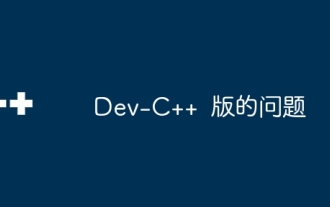
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
