Why do you need to reverse a PHP array?
PHP Array reversal has the following uses: getting the last element, traversing in reverse order, and creating a descending array. There are two methods of reversal: 1. array_reverse() function; 2. Use for loop. For example, to print a student resume in reverse order: first use array_reverse() to reverse the array, then traverse the reversed array in reverse order, outputting the name, age, and skills of each student.
#Why do you need to reverse a PHP array?
In PHP, array reversal is very useful in the following situations:
- Get the last element: The last element of the array can be reversed by Convert an array to get it easily.
- Traverse in reverse order: Reversing the array can conveniently traverse the array elements in reverse order.
- Creating a descending array: Reversing an array creates a descending version of a sorted array.
Two methods to reverse PHP array
1. array_reverse() function
<?php $arr = [1, 2, 3, 4, 5]; // 反转数组 $reversed_arr = array_reverse($arr); // 打印反转数组 print_r($reversed_arr); ?>
2. Using a For loop
<?php $arr = [1, 2, 3, 4, 5]; // 创建一个新数组来存储反转后的元素 $reversed_arr = []; // 使用 for 循环倒序遍历 original 数组 for ($i = count($arr) - 1; $i >= 0; $i--) { // 将元素添加到 reversed 数组的开头 array_unshift($reversed_arr, $arr[$i]); } // 打印反转数组 print_r($reversed_arr); ?>
Practical case
Print students’ resumes in reverse order
// 模拟学生履历表数组 $students = [ ['name' => 'John', 'age' => 21, 'skills' => ['PHP', 'JavaScript']], ['name' => 'Jane', 'age' => 22, 'skills' => ['HTML', 'CSS']], ['name' => 'Bob', 'age' => 23, 'skills' => ['Java', 'Python']], ]; // 使用 array_reverse() 反转数组 $reversed_students = array_reverse($students); // 倒序遍历反转的数组 foreach ($reversed_students as $student) { echo "Name: {$student['name']}, Age: {$student['age']}, Skills: "; foreach ($student['skills'] as $skill) { echo "$skill "; } echo "<br>"; }
This code will print the student's resume in reverse order:
Name: Bob, Age: 23, Skills: Java Python Name: Jane, Age: 22, Skills: HTML CSS Name: John, Age: 21, Skills: PHP JavaScript
The above is the detailed content of Why do you need to reverse a PHP array?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


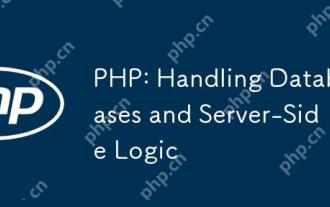
PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
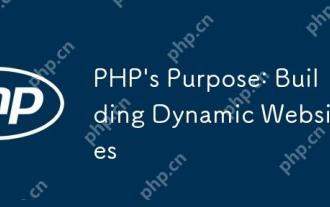
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.
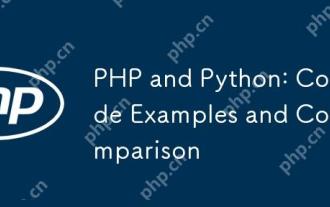
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
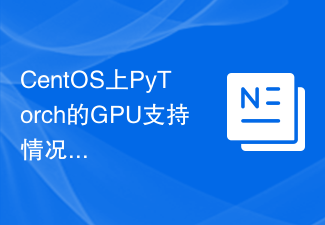
Enable PyTorch GPU acceleration on CentOS system requires the installation of CUDA, cuDNN and GPU versions of PyTorch. The following steps will guide you through the process: CUDA and cuDNN installation determine CUDA version compatibility: Use the nvidia-smi command to view the CUDA version supported by your NVIDIA graphics card. For example, your MX450 graphics card may support CUDA11.1 or higher. Download and install CUDAToolkit: Visit the official website of NVIDIACUDAToolkit and download and install the corresponding version according to the highest CUDA version supported by your graphics card. Install cuDNN library:
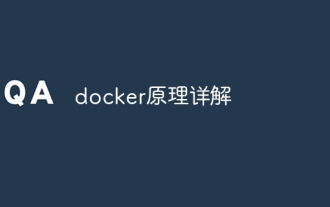
Docker uses Linux kernel features to provide an efficient and isolated application running environment. Its working principle is as follows: 1. The mirror is used as a read-only template, which contains everything you need to run the application; 2. The Union File System (UnionFS) stacks multiple file systems, only storing the differences, saving space and speeding up; 3. The daemon manages the mirrors and containers, and the client uses them for interaction; 4. Namespaces and cgroups implement container isolation and resource limitations; 5. Multiple network modes support container interconnection. Only by understanding these core concepts can you better utilize Docker.
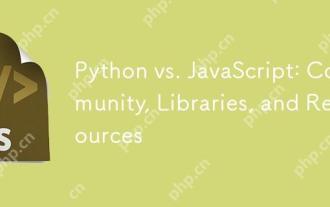
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.

PyTorch distributed training on CentOS system requires the following steps: PyTorch installation: The premise is that Python and pip are installed in CentOS system. Depending on your CUDA version, get the appropriate installation command from the PyTorch official website. For CPU-only training, you can use the following command: pipinstalltorchtorchvisiontorchaudio If you need GPU support, make sure that the corresponding version of CUDA and cuDNN are installed and use the corresponding PyTorch version for installation. Distributed environment configuration: Distributed training usually requires multiple machines or single-machine multiple GPUs. Place
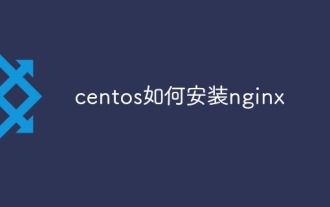
CentOS Installing Nginx requires following the following steps: Installing dependencies such as development tools, pcre-devel, and openssl-devel. Download the Nginx source code package, unzip it and compile and install it, and specify the installation path as /usr/local/nginx. Create Nginx users and user groups and set permissions. Modify the configuration file nginx.conf, and configure the listening port and domain name/IP address. Start the Nginx service. Common errors need to be paid attention to, such as dependency issues, port conflicts, and configuration file errors. Performance optimization needs to be adjusted according to the specific situation, such as turning on cache and adjusting the number of worker processes.
