Best practices for error handling in golang functions
GoLang function error handling best practices include: using the error variable to receive errors; checking nil values to determine whether there is an error; using the errors package to create custom error messages; using the defer recovery mechanism to handle panics; practical cases demonstrate these best practices Practice the application of CSV conversion JSON function.
GoLang Function Error Handling Best Practices
When handling errors in GoLang, there are several best practices to ensure that your code is robust and easy to maintain. Let's explore some of the most common techniques:
Using error
Variables
The error
type in GoLang represents any error that may occur during function operation . These errors should be received using the error
variable and handled carefully. The following example demonstrates this:
func divide(a, b int) (float64, error) { if b == 0 { return 0, errors.New("divisor cannot be zero") } return float64(a) / float64(b), nil }
Check nil
error
The value can be nil
, indicating no error. Therefore, when using the error
variable, it is important to check nil
to determine if an error exists. For example:
if err != nil { // 处理错误(打印、记录等) }
Using the errors
package
GoLang’s errors
package provides useful functions for creating and handling errors. Particularly useful for creating custom error messages:
import "errors" // 创建自定义错误类型 var MyError = errors.New("my custom error")
defer
The recovery mechanism
defer
statement allows you to delay the execution of an operation until the function returns. It is often used in conjunction with the recover()
built-in function in order to recover from panics and handle errors:
func recoverExample() { defer func() { if err := recover(); err != nil { // 处理从恐慌中恢复的错误 } }() // 代码可能导致恐慌 }
Practical Case
Let us consider a CSV file that converts A function for a JSON object that uses the above best practices:
import ( "encoding/csv" "errors" "io" "strconv" ) // 将 CSV 文件转换成 JSON 对象 func CSVtoJSON(csvReader io.Reader) (map[string]interface{}, error) { csvData, err := csv.NewReader(csvReader).ReadAll() if err != nil { return nil, err } data := make(map[string]interface{}) for _, row := range csvData { name := row[0] value := row[1] if _, ok := data[name]; ok { return nil, errors.New("duplicate key: " + name) } // 将 string 转换为 float64 floatValue, err := strconv.ParseFloat(value, 64) if err != nil { return nil, err } data[name] = floatValue } return data, nil }
By following these best practices, you can ensure that errors are handled in a robust and efficient manner in your GoLang functions.
The above is the detailed content of Best practices for error handling in golang functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


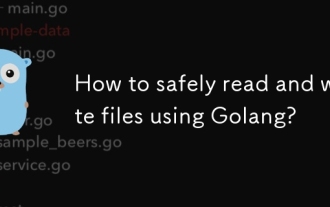
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
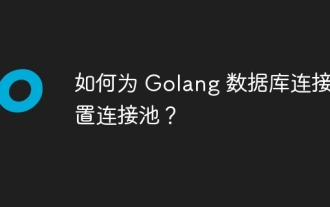
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
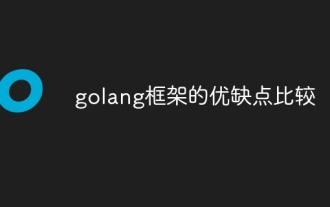
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
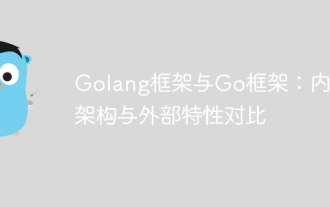
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
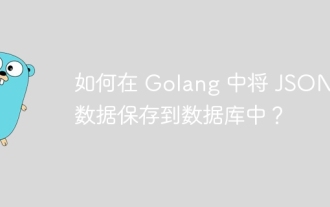
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
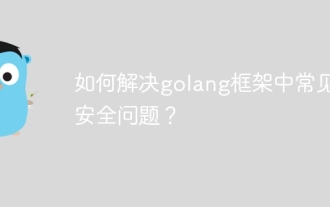
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
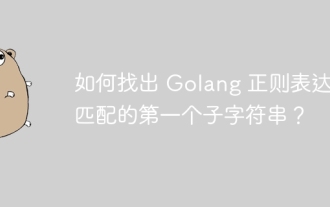
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
