


The Art of Sorting Multidimensional Arrays in PHP: Optimizing Performance and Readability
When sorting a multidimensional array, you can use built-in functions such as sort() to sort the first subarray elements, and asort() to sort the specified elements in the subarray. Custom comparison functions allow sorting based on specific attributes. To optimize performance, consider using in-place sort functions, more efficient algorithms, and indexed arrays. Practical examples include multi-level sorting by student performance or product price and name.
The Art of Sorting Multidimensional Arrays in PHP: Optimizing Performance and Readability
Efficient sorting of multidimensional arrays is crucial when working with complex data sets important. PHP provides several methods for sorting multidimensional arrays, each with its own unique advantages and disadvantages. This article will explore various sequencing methods and provide practical examples for different use cases.
Using the sort()
function
sort()
function is the simplest sorting function built into PHP. It sorts the given array in-place, which means it modifies the original array. The following is an example of sorting a multi-dimensional array using the sort()
function:
$array = [ ['name' => 'John', 'age' => 30], ['name' => 'Jane', 'age' => 25], ['name' => 'Alice', 'age' => 28], ]; sort($array); print_r($array);
The above code will sort the array in ascending order based on the first element of the first sub-array (name) .
Use the asort()
function
asort()
The function is similar to the sort()
function, but it works on arrays Sort the values in ascending order. Here is an example of sorting a multidimensional array using the asort()
function:
$array = [ ['name' => 'John', 'age' => 30], ['name' => 'Jane', 'age' => 25], ['name' => 'Alice', 'age' => 28], ]; asort($array); print_r($array);
The above code sorts the array in ascending order based on the second element in each subarray, which is age.
Use user-defined comparison functions
For more complex multi-dimensional array sorting requirements, you can use custom comparison functions. Custom comparison functions allow you to sort based on specific properties of array elements. The following is an example of sorting a multidimensional array using a user-defined comparison function:
function compareByName($a, $b) { return strcmp($a['name'], $b['name']); } $array = [ ['name' => 'John', 'age' => 30], ['name' => 'Jane', 'age' => 25], ['name' => 'Alice', 'age' => 28], ]; usort($array, 'compareByName'); print_r($array);
The above code will sort the array in ascending order based on the name attribute.
Optimizing performance
When dealing with large data sets, it is very important to optimize sorting performance. Here are some optimization tips:
- Use in-place sort functions like
sort()
and `asort()) to avoid array copies. - Use a sorting algorithm with lower algorithmic complexity for large arrays, such as merge sort or heap sort.
- Use indexed arrays instead of associative arrays because indexed arrays are accessed faster than associative arrays.
Practical Case
Case 1: Sorting student data by student performance
$students = [ ['name' => 'John', 'score' => 90], ['name' => 'Jane', 'score' => 85], ['name' => 'Alice', 'score' => 95], ]; usort($students, function($a, $b) { return $a['score'] <=> $b['score']; });
Case 2: By product price Multi-level sorting of product array by name
$products = [ ['name' => 'Product A', 'price' => 100], ['name' => 'Product B', 'price' => 50], ['name' => 'Product A', 'price' => 120], ]; usort($products, function($a, $b) { if ($a['price'] == $b['price']) { return strcmp($a['name'], $b['name']); } return $a['price'] <=> $b['price']; });
The above is the detailed content of The Art of Sorting Multidimensional Arrays in PHP: Optimizing Performance and Readability. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


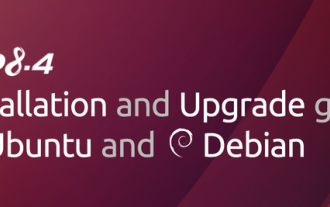
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
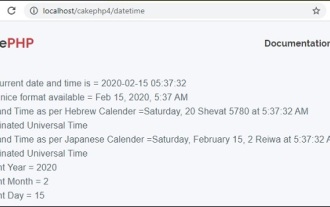
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
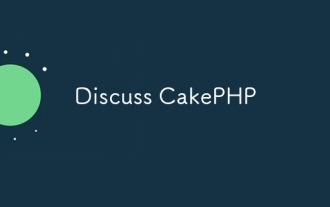
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
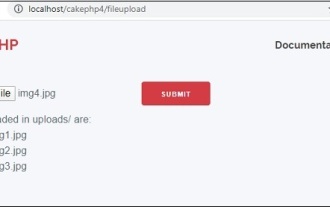
To work on file upload we are going to use the form helper. Here, is an example for file upload.
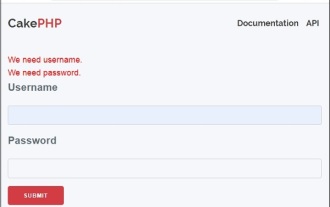
Validator can be created by adding the following two lines in the controller.
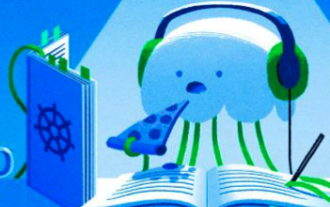
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
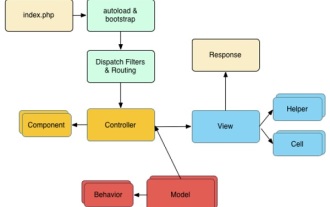
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
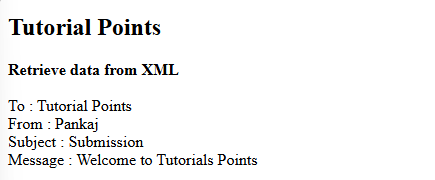
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
