


How to use performance analysis tools to analyze and optimize Java functions?
Java performance analysis tools can be used to analyze and optimize the performance of Java functions. Choose a profiling tool: JVisualVM, VisualVM, Java Flight Recorder (JFR), etc. Configure performance analysis tools: set sampling rate, enable events. Execute the function and collect data: Execute the function after enabling the profiling tool. Analyze performance data: Identify bottleneck indicators such as CPU usage, memory usage, execution time, hotspots, and more. Optimize functions: Use optimization algorithms, refactor code, use caching and other technologies to improve efficiency.
Use performance analysis tools to analyze and optimize Java functions
Java performance analysis tools are valuable tools for diagnosing and optimizing Java code performance . This article guides you through using performance analysis tools to analyze and optimize Java functions.
Choose a profiling tool
There are many profiling tools for Java, including:
- JVisualVM
- VisualVM
- Java Flight Recorder (JFR)
- YourKit
Choose a tool based on your specific needs and preferences.
Configuring the Performance Analysis Tool
Install and configure your performance analysis tool to monitor Java functions. This may include setting the sample rate, enabling specific events, or loading an agent. Follow the instructions in the tool documentation.
Execute the function and collect data
After enabling the performance analysis tool, execute the Java function. The tool collects data about the function's runtime behavior.
Analyze performance data
After collecting the data, analyze it using performance analysis tools to identify performance bottlenecks. Check the following metrics:
- CPU Usage: The amount of CPU resources consumed by the function.
- Memory usage: The amount of memory used by the function.
- Execution time: The time required for the function to complete execution.
- Hot spots: The line or method in the function that consumes the most resources.
Optimize function
Based on the performance analysis results, optimize the function to improve its efficiency. Try the following techniques:
- Optimize algorithms: Use more efficient algorithms or data structures.
- Refactor code: Remove unnecessary code or reorganize code to improve readability and maintainability.
- Use caching: Cache frequently accessed data to reduce access to underlying resources.
Practical Case
Suppose we have a Java function that calculates the nth term of the Fibonacci sequence. Let's use JVisualVM to analyze and optimize it:
public class Fibonacci { public static int fib(int n) { if (n <= 1) { return 1; } return fib(n - 1) + fib(n - 2); } }
Let's use JVisualVM to analyze this function. We see CPU usage is high because the function is recursive, resulting in a large number of stack calls.
In order to optimize the function, we use Memoization to store the results of previous calculations in the cache. The modified code is as follows:
import java.util.HashMap; import java.util.Map; public class Fibonacci { private static Map<Integer, Integer> cache = new HashMap<>(); public static int fib(int n) { if (n <= 1) { return 1; } if (cache.containsKey(n)) { return cache.get(n); } int result = fib(n - 1) + fib(n - 2); cache.put(n, result); return result; } }
This optimization significantly reduces CPU usage and improves the efficiency of the function.
The above is the detailed content of How to use performance analysis tools to analyze and optimize Java functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
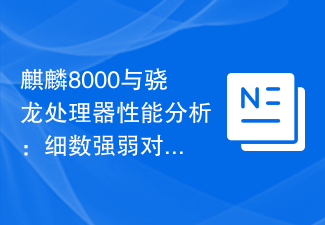
Kirin 8000 and Snapdragon processor performance analysis: detailed comparison of strengths and weaknesses. With the popularity of smartphones and their increasing functionality, processors, as the core components of mobile phones, have also attracted much attention. One of the most common and excellent processor brands currently on the market is Huawei's Kirin series and Qualcomm's Snapdragon series. This article will focus on the performance analysis of Kirin 8000 and Snapdragon processors, and explore the comparison of the strengths and weaknesses of the two in various aspects. First, let’s take a look at the Kirin 8000 processor. As Huawei’s latest flagship processor, Kirin 8000
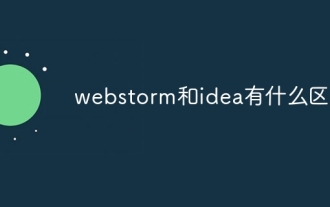
WebStorm is tailor-made for web development and provides powerful features for web development languages, while IntelliJ IDEA is a versatile IDE that supports multiple languages. Their differences mainly lie in language support, web development features, code navigation, debugging and testing capabilities, and additional features. The final choice depends on language preference and project needs.
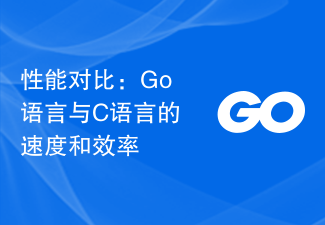
Performance comparison: speed and efficiency of Go language and C language In the field of computer programming, performance has always been an important indicator that developers pay attention to. When choosing a programming language, developers usually focus on its speed and efficiency. Go language and C language, as two popular programming languages, are widely used for system-level programming and high-performance applications. This article will compare the performance of Go language and C language in terms of speed and efficiency, and demonstrate the differences between them through specific code examples. First, let's take a look at the overview of Go language and C language. Go language is developed by G
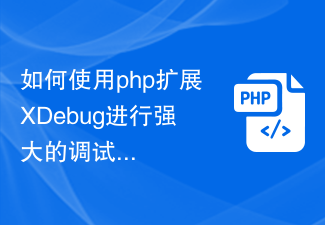
How to use the PHP extension Xdebug for powerful debugging and performance analysis Introduction: In the process of developing PHP applications, debugging and performance analysis are essential links. Xdebug is a powerful debugging tool commonly used by PHP developers. It provides a series of advanced functions, such as breakpoint debugging, variable tracking, performance analysis, etc. This article will introduce how to use Xdebug for powerful debugging and performance analysis, as well as some practical tips and precautions. 1. Install Xdebug and start using Xdebu
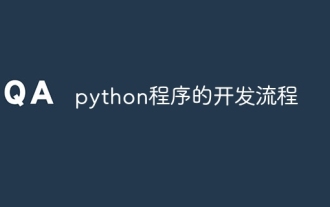
The Python program development process includes the following steps: Requirements analysis: clarify business needs and project goals. Design: Determine architecture and data structures, draw flowcharts or use design patterns. Writing code: Program in Python, following coding conventions and documentation comments. Testing: Writing unit and integration tests, conducting manual testing. Review and Refactor: Review code to find flaws and improve readability. Deploy: Deploy the code to the target environment. Maintenance: Fix bugs, improve functionality, and monitor updates.
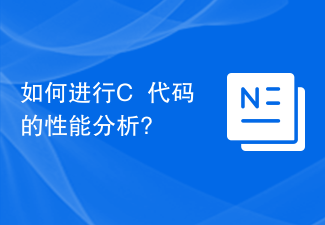
How to perform performance analysis of C++ code? Performance is an important consideration when developing C++ programs. Optimizing the performance of your code can improve the speed and efficiency of your program. However, to optimize your code, you first need to understand where its performance bottlenecks are. To find the performance bottleneck, you first need to perform code performance analysis. This article will introduce some commonly used C++ code performance analysis tools and techniques to help developers find performance bottlenecks in the code for optimization. Profiling tool using Profiling tool
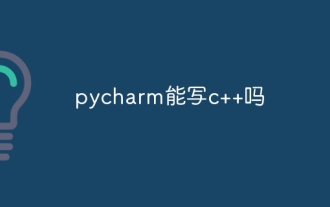
Yes, PyCharm can write C++ code. It is a cross-platform IDE that supports multiple languages, including C++. After installing the C++ plugin, you can use PyCharm's features such as code editor, compiler, debugger, and test runner to write and run C++ code.
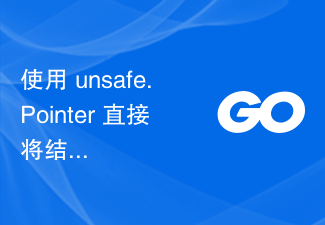
is it safe? (*teamdata)(unsafe.pointer(&team.id)) Sample code: functestTrans()[]*TeamData{teams:=createTeams()teamDatas:=make([]*TeamData,0,len(teams))for_, team:=rangeteams{//isthissafe?teamDatas=append(teamDatas,
