


How to identify and improve Java function performance issues through code review?
Code review identifies performance issues with Java functions, including Big O complexity analysis, benchmarking, code coverage, and memory analysis. Through practical cases, it is demonstrated that optimizing linear search into binary search or hash table search can improve performance. Additionally, suggestions for improvements include avoiding unnecessary loops, using caches, parallelizing, choosing appropriate data structures, and using built-in methods.
Identify and improve performance issues of Java functions through code review
Code review is critical to ensuring software quality, and performance Optimization is a key aspect of this. By carefully examining the code of a Java function, you can identify potential performance issues and develop improvements.
Common Ways to Identify Performance Problems
- Big O Complexity Analysis: Determine the asymptotic growth rate of a function over input size , to evaluate its efficiency.
- Benchmarking: Use benchmarking tools to measure the execution time and resource usage of functions.
- Code Coverage: Identify code paths that are not executed, which may be signs of performance bottlenecks.
- Memory Analysis: Check memory allocation and deallocation to identify memory leaks or fragmentation.
Practical Case: Linear Search Optimization
Consider the following linear search function for finding a given element in an array:
public static int linearSearch(int[] arr, int target) { for (int i = 0; i < arr.length; i++) { if (arr[i] == target) { return i; } } return -1; }
Performance issues: For large arrays, the complexity of linear search is O(n), and as the array size increases, its search time will increase significantly.
Improvement measures:
- Use binary search: For sorted arrays, the binary search algorithm has a complexity of O(log n) , significantly improving search efficiency.
- Use a hash table: Storing array elements in a hash table can reduce the search complexity to O(1), which is a great improvement over linear search.
Other common improvement suggestions
- Avoid unnecessary loops: Traverse the data structure only when needed.
- Use cache: Store the results of repeated calculations to reduce overhead.
- Parallelization: Distribute computing tasks to multiple threads to improve efficiency.
- Consider the choice of data structure: Choose an appropriate collection class based on the type of data operation.
- Use built-in methods: Take advantage of the optimization methods provided by Java libraries instead of reinventing the wheel.
The above is the detailed content of How to identify and improve Java function performance issues through code review?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


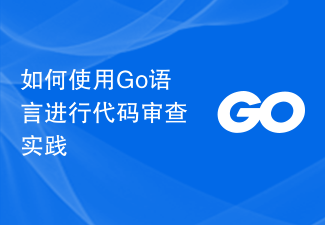
How to use Go language for code review practice Introduction: In the software development process, code review (CodeReview) is an important practice. By reviewing and analyzing each other's code, team members can identify potential problems, improve code quality, increase teamwork, and share knowledge. This article will introduce how to use Go language for code review practices, and attach code examples. 1. The importance of code review Code review is a best practice to promote code quality. It can find and correct potential errors in the code, improve the code
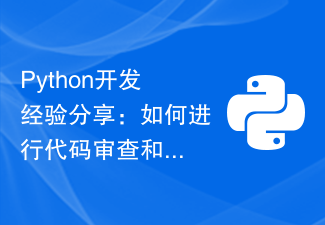
Python development experience sharing: How to conduct code review and quality assurance Introduction: In the software development process, code review and quality assurance are crucial links. Good code review can improve code quality, reduce errors and defects, and improve program maintainability and scalability. This article will share the experience of code review and quality assurance in Python development from the following aspects. 1. Develop code review specifications Code review is a systematic activity that requires a comprehensive inspection and evaluation of the code. In order to standardize code review
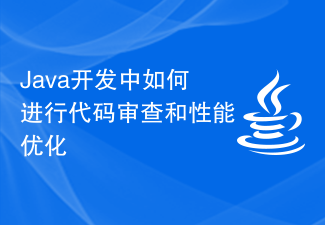
How to conduct code review and performance optimization in Java development requires specific code examples. In the daily Java development process, code review and performance optimization are very important links. Code review can ensure the quality and maintainability of the code, while performance optimization can improve the operating efficiency and response speed of the system. This article will introduce how to conduct Java code review and performance optimization, and give specific code examples. Code review Code review is the process of checking the code line by line as it is written and fixing potential problems and errors. the following
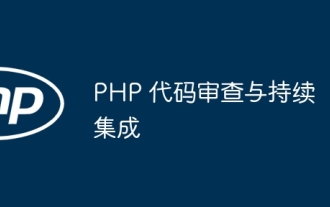
Yes, combining code reviews with continuous integration can improve code quality and delivery efficiency. Specific tools include: PHP_CodeSniffer: Check coding style and best practices. PHPStan: Detect errors and unused variables. Psalm: Provides type checking and advanced code analysis.
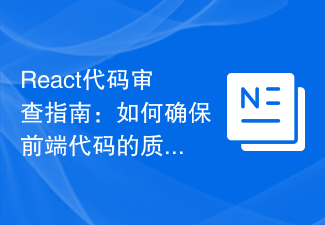
React Code Review Guide: How to Ensure the Quality and Maintainability of Front-End Code Introduction: In today’s software development, front-end code is increasingly important. As a popular front-end development framework, React is widely used in various types of applications. However, due to the flexibility and power of React, writing high-quality and maintainable code can become a challenge. To address this issue, this article will introduce some best practices for React code review and provide some concrete code examples. 1. Code style
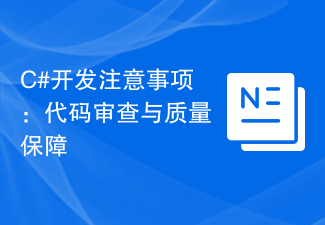
In the C# development process, code quality assurance is crucial. The quality of code directly affects the stability, maintainability and scalability of software. As an important quality assurance method, code review plays a role that cannot be ignored in software development. This article will focus on code review considerations in C# development to help developers improve code quality. 1. The purpose and significance of review Code review refers to the process of discovering and correcting existing problems and errors by carefully reading and inspecting the code. Its main purpose is to improve the
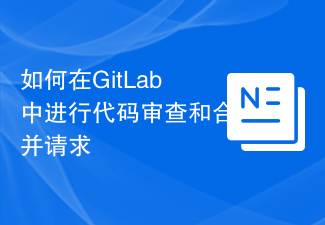
How to Conduct Code Reviews and Merge Requests in GitLab Code review is an important development practice that can help teams identify potential problems and improve code quality. In GitLab, through the merge request (MergeRequest) function, we can easily conduct code review and merge work. This article explains how to perform code reviews and merge requests in GitLab, while providing specific code examples. Preparation: Please make sure you have created a GitLab project and have the relevant
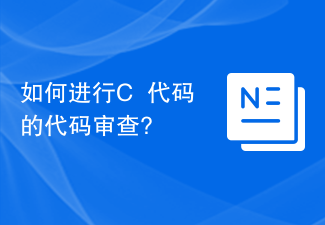
How to conduct code review of C++ code? Code review is a very important part of the software development process. It can help the development team identify and correct potential errors, improve code quality, and reduce the workload of subsequent maintenance and debugging. For strongly typed static languages like C++, code review is particularly important. Here are some key steps and considerations to help you conduct an effective C++ code review. Set code review standards: Before conducting a code review, the team should jointly develop a code review standard to agree on various errors and violations.
