Can golang variadic parameters be used in unit testing?
Variadic parameters in the Go language allow functions to receive a variable number of parameters, using the ... syntax. However, unit tests cannot pass variadic parameters directly. You can use reflection to create a test helper function and call the function dynamically using the reflect.ValueOf(sumNumbers).CallSlice(reflect.ValueOf(tt.args)) method.
Variadic parameters in the Go language
Variadic parameters allow a function to receive a variable number of parameters. In Go, variadic arguments use the ...
syntax.
Practical combat
The following code demonstrates the use of variable parameters:
func sumNumbers(args ...int) int { sum := 0 for _, v := range args { sum += v } return sum } func main() { // 传递一个参数 result := sumNumbers(10) fmt.Println(result) // 输出:10 // 传递多个参数 result = sumNumbers(10, 20, 30) fmt.Println(result) // 输出:60 }
Variable parameters in unit tests
Variable parameters cannot be passed directly in unit tests. This is because the Go language testing framework does not support them.
However, we can use reflection to dynamically call functions with variable parameters. The following code demonstrates how to create a test helper function for this:
func TestSumNumbers(t *testing.T) { tests := []struct { args []int want int }{ {[]int{10}, 10}, {[]int{10, 20}, 30}, } for _, tt := range tests { t.Run(fmt.Sprintf("%v", tt.args), func(t *testing.T) { got := reflect.ValueOf(sumNumbers).CallSlice(reflect.ValueOf(tt.args)) if got.Int() != tt.want { t.Errorf("sumNumbers(%v) = %v, want %v", tt.args, got.Int(), tt.want) } }) } }
This helper function dynamically calls the sumNumbers
function using the CallSlice
method, passing a []int
slice as parameter.
The above is the detailed content of Can golang variadic parameters be used in unit testing?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
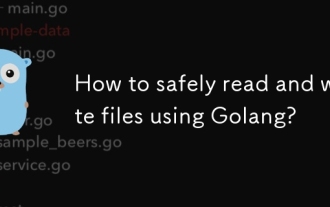
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
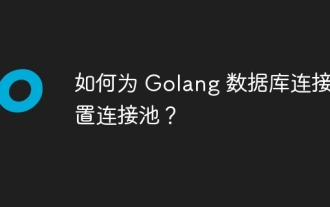
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
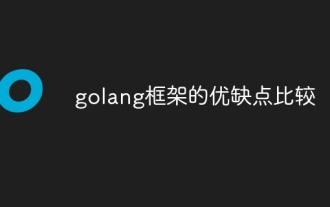
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
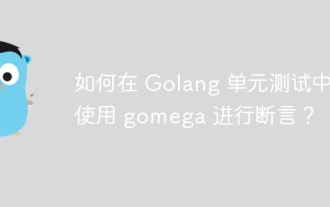
How to use Gomega for assertions in Golang unit testing In Golang unit testing, Gomega is a popular and powerful assertion library that provides rich assertion methods so that developers can easily verify test results. Install Gomegagoget-ugithub.com/onsi/gomega Using Gomega for assertions Here are some common examples of using Gomega for assertions: 1. Equality assertion import "github.com/onsi/gomega" funcTest_MyFunction(t*testing.T){
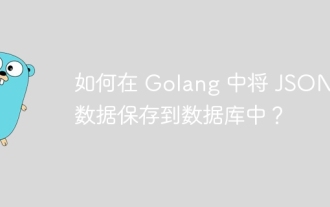
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
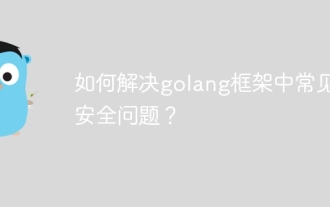
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
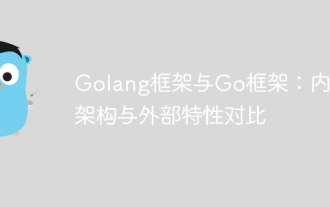
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
