Examples of flag usage in C language
Usage of flag in C language: Program control: represents true/false status, used to control program flow based on conditions. Status indicator: Indicates a specific status, such as whether it is registered. Error handling: Used to handle errors, such as returning an error code when a file fails to open. Parameter passing: Indicates optional parameters, used to modify function behavior.
Usage examples of flag in C language
flag is a widely used variable type in C language, representing A Boolean value (true or false). It is often used to control program flow or indicate a specific status. Here are some common usage examples of flag:
As a program control
int main() { int flag = 0; // 初始化为假 // 当满足某个条件时,将 flag 设置为真 if (condition) { flag = 1; } // 根据 flag 的值执行不同的操作 if (flag) { // 条件为真时执行的代码 } else { // 条件为假时执行的代码 } return 0; }
As a status indicator
struct student { int id; char name[50]; int enrolled; // 0: 未注册,1: 已注册 }; void register_student(struct student *student) { student->enrolled = 1; } int is_student_enrolled(struct student *student) { return student->enrolled; }
Handling as error
int open_file(const char *filename) { FILE *file; file = fopen(filename, "r"); if (!file) { return -1; // 返回错误代码 } return file; } int main() { FILE *file = open_file("myfile.txt"); if (file == -1) { // 处理文件打开错误 } // 使用 file 指针读取文件内容 fclose(file); return 0; }
Passed as parameter
void print_array(int arr[], int size, int reverse) { if (reverse) { for (int i = size - 1; i >= 0; i--) { printf("%d ", arr[i]); } } else { for (int i = 0; i < size; i++) { printf("%d ", arr[i]); } } } int main() { int arr[] = {1, 2, 3, 4, 5}; int size = sizeof(arr) / sizeof(arr[0]); print_array(arr, size, 0); // 正序打印数组 print_array(arr, size, 1); // 倒序打印数组 return 0; }
The above is the detailed content of Examples of flag usage in C language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


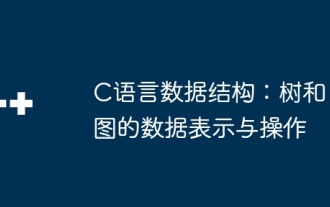
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
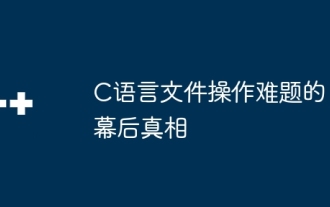
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
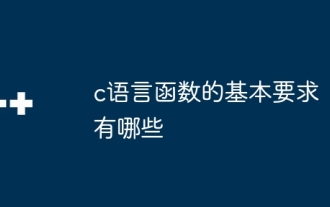
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
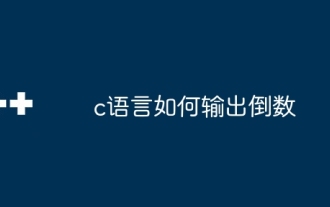
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
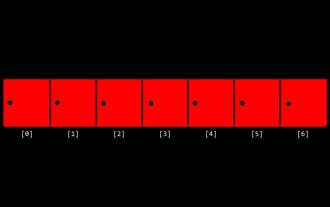
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
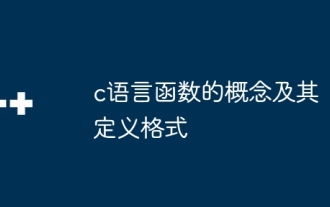
C language functions are reusable code blocks, receive parameters for processing, and return results. It is similar to the Swiss Army Knife, powerful and requires careful use. Functions include elements such as defining formats, parameters, return values, and function bodies. Advanced usage includes function pointers, recursive functions, and callback functions. Common errors are type mismatch and forgetting to declare prototypes. Debugging skills include printing variables and using a debugger. Performance optimization uses inline functions. Function design should follow the principle of single responsibility. Proficiency in C language functions can significantly improve programming efficiency and code quality.
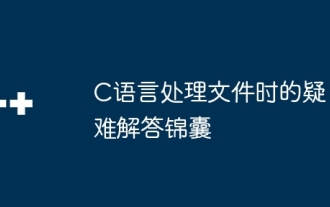
Troubleshooting Tips for C language processing files When processing files in C language, you may encounter various problems. The following are common problems and corresponding solutions: Problem 1: Cannot open the file code: FILE*fp=fopen("myfile.txt","r");if(fp==NULL){//File opening failed} Reason: File path error File does not exist without file read permission Solution: Check the file path to ensure that the file has check file permission problem 2: File reading failed code: charbuffer[100];size_tread_bytes=fread(buffer,1,siz
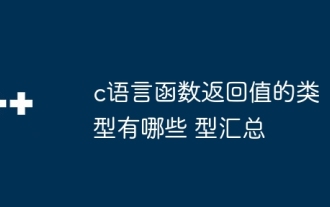
The return value types of C language function include int, float, double, char, void and pointer types. int is used to return integers, float and double are used to return floats, and char returns characters. void means that the function does not return any value. The pointer type returns the memory address, be careful to avoid memory leakage.结构体或联合体可返回多个相关数据。
