


Dimensional journey of PHP multi-dimensional array sorting: from one dimension to multi-dimensional
One-dimensional arrays use the sort() function to sort, two-dimensional arrays use the usort() function to sort by internal elements, high-dimensional arrays use the multi-layer nested usort() function to sort by hierarchical elements, and the decomposition problem is solved layer by layer. is the key.
The dimensional journey of PHP multi-dimensional array sorting: from one dimension to multi-dimensional
In PHP, array is a powerful data structure that can Stores various types of data, including multidimensional arrays. Multidimensional arrays are arrays that contain other arrays, which allow us to create complex data structures.
Sorting multidimensional arrays can be a challenging task, but understanding the concepts behind it is crucial. In this article, we will take a journey into dimensions and learn how to sort multi-dimensional arrays of one, two and higher dimensions using PHP built-in functions.
One-dimensional array sorting
One-dimensional array sorting is the simplest form. Using the sort()
function, we can sort the elements in the array in ascending order:
<?php $arr = [5, 2, 8, 3, 1]; sort($arr); print_r($arr); // 输出:[1, 2, 3, 5, 8] ?>
Two-dimensional array sorting
The sorting of two-dimensional arrays is slightly more complicated. We can sort based on the elements in its internal array. For example, suppose we have a two-dimensional array containing student grades:
<?php $students = [ ['name' => 'Alice', 'score' => 90], ['name' => 'Bob', 'score' => 80], ['name' => 'Carol', 'score' => 70] ]; ?>
To sort students in descending order based on their scores, we can use the usort()
function:
<?php usort($students, function($a, $b) { return $b['score'] <=> $a['score']; }); print_r($students); // 输出:[ // ['name' => 'Alice', 'score' => 90], // ['name' => 'Bob', 'score' => 80], // ['name' => 'Carol', 'score' => 70] // ] ?>
High Dimension Array Sorting
The concept of sorting is the same for higher dimensional arrays. For example, let us consider a three-dimensional array containing information about three students' classes:
<?php $classes = [ [ ['name' => 'Alice', 'grade' => 'A'], ['name' => 'Bob', 'grade' => 'B'], ['name' => 'Carol', 'grade' => 'C'] ], [ ['name' => 'Dave', 'grade' => 'A'], ['name' => 'Eve', 'grade' => 'B'], ['name' => 'Frank', 'grade' => 'C'] ], [ ['name' => 'George', 'grade' => 'A'], ['name' => 'Helen', 'grade' => 'B'], ['name' => 'Ian', 'grade' => 'C'] ] ]; ?>
To sort all three classes in descending order based on the students' grades, we can use a multilevel usort( )
Function nesting:
<?php usort($classes, function($a, $b) { usort($a, function($c, $d) { return $d['grade'] <=> $c['grade']; }); usort($b, function($c, $d) { return $d['grade'] <=> $c['grade']; }); return $b[0]['grade'] <=> $a[0]['grade']; }); print_r($classes); // 输出:[ // [ // ['name' => 'Alice', 'grade' => 'A'], // ['name' => 'Bob', 'grade' => 'B'], // ['name' => 'Carol', 'grade' => 'C'] // ], // [ // ['name' => 'Dave', 'grade' => 'A'], // ['name' => 'Eve', 'grade' => 'B'], // ['name' => 'Frank', 'grade' => 'C'] // ], // [ // ['name' => 'George', 'grade' => 'A'], // ['name' => 'Helen', 'grade' => 'B'], // ['name' => 'Ian', 'grade' => 'C'] // ] // ] ?>
The key to understanding multi-dimensional array sorting is to decompose the problem and use nested usort()
functions to solve it layer by layer. This way we can easily sort complex data structures with arbitrary dimensions.
The above is the detailed content of Dimensional journey of PHP multi-dimensional array sorting: from one dimension to multi-dimensional. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
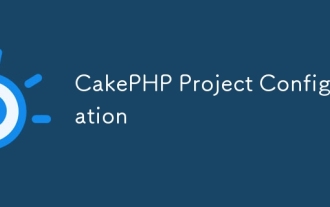
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
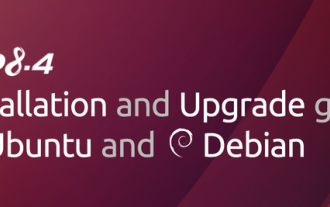
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
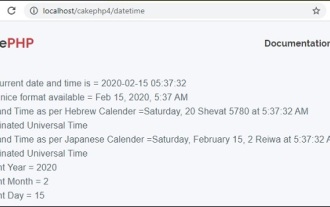
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
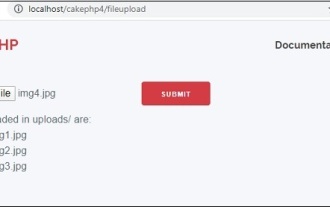
To work on file upload we are going to use the form helper. Here, is an example for file upload.
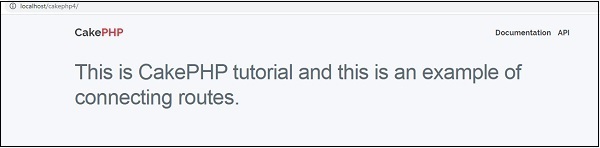
In this chapter, we are going to learn the following topics related to routing ?
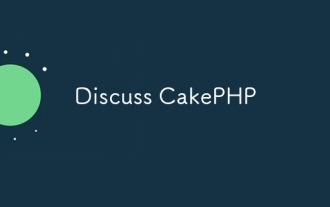
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
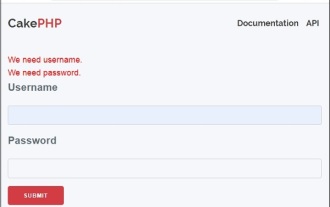
Validator can be created by adding the following two lines in the controller.
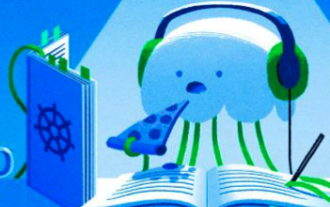
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
