


C++ function pointers and Qt framework: building flexible GUI applications
Application of function pointers in C and Qt frameworks: Function pointers allow functions to be passed as variables. The Qt framework uses a signals and slots mechanism that allows function pointers to be assigned to event handlers. Slot functions can be assigned to signals via the connect() function. Practical examples show how to use function pointers and the Qt framework to build GUI applications, including creating buttons, assigning slot functions, and running event loops.
C Function Pointers and Qt Framework: Building Flexible GUI Applications
Overview
Function pointers are powerful tools in C. They Allows you to pass functions as variables. This is very useful in GUI programming because you have the flexibility to assign different functions to event handlers. The Qt framework makes extensive use of function pointers, providing great flexibility for building dynamic and customizable GUIs.
Basic knowledge of function pointers
Function pointers are pointers to functions. The syntax is as follows:
returnType (*function_pointer_name)(param_list);
where:
returnType
is the type of value returned by the function.function_pointer_name
is the name of the function pointer.param_list
is the type list of function parameters.
To get a pointer to a function, you can use the type conversion operator&
:
returnType (*function_pointer_name)(param_list) = &function_name;
Using function pointers in Qt
The Qt framework provides a convenient way to use function pointers: signals and slots. Signals are events that are triggered in an object, and slots are functions that respond to signals. You can assign a function pointer to a slot to specify a function to execute when a signal fires.
For example, the following is a slot function that will print text when a button is clicked:
void printText() { qDebug() << "Button clicked!"; }
To assign a slot function to a button's clicked signal, use connect()
Function:
QObject::connect(button, SIGNAL(clicked()), &printText, SLOT());
Practical case
The following is a practical case of using function pointers and Qt framework to build GUI applications:
#include <QtWidgets> int main(int argc, char **argv) { QApplication a(argc, argv); // 创建一个按钮 QPushButton button("Print Text"); // 将函数指针分配给 clicked 信号 QObject::connect(&button, SIGNAL(clicked()), &printText, SLOT()); // 显示按钮 button.show(); // 运行事件循环 return a.exec(); }
Description:
- This program creates a button and will print text when the button is clicked. The
-
QObject::connect()
function assigns theprintText
function pointer to the button'sclicked
signal. - The application event loop will handle the click event and trigger the
printText
function.
Conclusion
By combining function pointers and the Qt framework, you can build flexible and customizable GUI applications. Using function pointers, you can dynamically assign different functions to event handlers as needed. This is essential for creating interactive and responsive interfaces.
The above is the detailed content of C++ function pointers and Qt framework: building flexible GUI applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
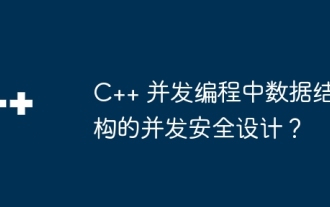
Concurrency-safe design of data structures in C++ concurrent programming?

C++ object layout is aligned with memory to optimize memory usage efficiency
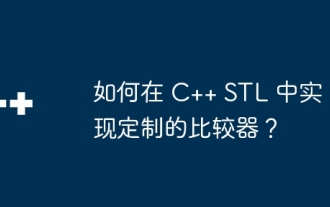
How to implement a custom comparator in C++ STL?
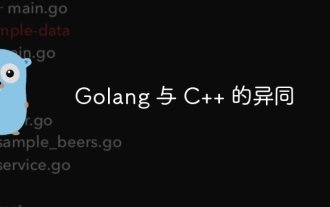
Similarities and Differences between Golang and C++
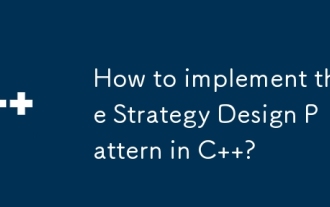
How to implement the Strategy Design Pattern in C++?
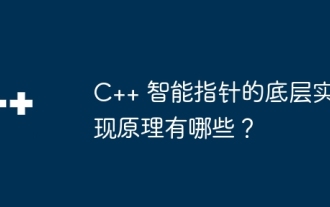
What are the underlying implementation principles of C++ smart pointers?
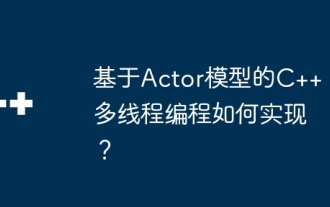
How to implement C++ multi-thread programming based on the Actor model?
