How to optimize data processing using NIO technology in Java functions?
Optimizing data processing using NIO involves the following steps: Create an NIO channel. Configure non-blocking mode. Create a selector. Register the channel to the selector. Select a ready channel. Channels ready for processing.
How to use NIO technology in Java functions to optimize data processing
Introduction
Non-blocking I/O (NIO) is a high-level I/O API used to enable efficient data processing in Java applications. Compared with traditional blocking I/O, NIO allows threads to perform other tasks while processing I/O operations, thereby improving concurrency and throughput.
Steps to use NIO
Using NIO to optimize data processing involves the following steps:
- Create an NIO channel: NioServerSocketChannel is used as the server endpoint, while NioSocketChannel is used as the client endpoint.
-
Configure non-blocking mode: Set the channel's
configureBlocking(false)
method tofalse
. -
Create Selector: The
Selector
object is used to monitor multiple channels and detect which channels are ready for read/write operations. - Register channels to selectors: Channels can be registered to selectors, specifying the read/write events they are interested in.
-
Select ready channels:
Selector.select()
The method blocks until one or more channels are ready for I/O operations. - Handling ready channels: For each ready channel, perform the appropriate read/write operations.
Practical Case
Consider a server-side application that needs to read data from the client and echo it back to the client. The following is a code snippet implemented using NIO:
public class NioServer { public static void main(String[] args) throws IOException { // 创建 NIO 通道 NioServerSocketChannel serverSocket = NioServerSocketChannel.open(); // 配置非阻塞模式 ServerSocketChannel.configureBlocking(false); // 绑定到端口 serverSocket.bind(new InetSocketAddress(8080)); // 创建选择器 Selector selector = Selector.open(); // 将服务器端点注册到选择器 serverSocket.register(selector, SelectionKey.OP_ACCEPT); while (true) { // 选择就绪的通道 selector.select(); // 处理就绪的通道 Iterator<SelectionKey> keys = selector.selectedKeys().iterator(); while (keys.hasNext()) { SelectionKey key = keys.next(); keys.remove(); if (key.isAcceptable()) { // 处理新连接 NioSocketChannel clientSocket = serverSocket.accept(); clientSocket.register(selector, SelectionKey.OP_READ); } else if (key.isReadable()) { // 读取数据 NioSocketChannel clientSocket = (NioSocketChannel) key.channel(); ByteBuffer buffer = ByteBuffer.allocate(1024); int bytesRead = clientSocket.read(buffer); if (bytesRead > 0) { // 回显数据 buffer.flip(); clientSocket.write(buffer); } else if (bytesRead == -1) { // 客户端已关闭连接 key.cancel(); clientSocket.close(); } } } } } }
By using NIO, this server application is able to handle multiple client connections simultaneously, improving concurrency and throughput.
The above is the detailed content of How to optimize data processing using NIO technology in Java functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


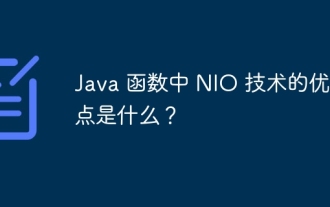
NIO (non-blocking IO) technology provides the advantages of high performance, scalability, low latency and low resource utilization in Java functions, but it also has higher complexity, the need for asynchronous programming, increased debugging difficulty, and system requirements. Higher disadvantages. In practice, NIO can optimize resource utilization and improve performance, such as when processing incoming HTTP requests.
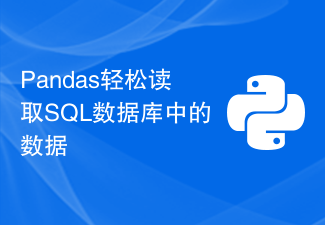
Data processing tool: Pandas reads data in SQL databases and requires specific code examples. As the amount of data continues to grow and its complexity increases, data processing has become an important part of modern society. In the data processing process, Pandas has become one of the preferred tools for many data analysts and scientists. This article will introduce how to use the Pandas library to read data from a SQL database and provide some specific code examples. Pandas is a powerful data processing and analysis tool based on Python
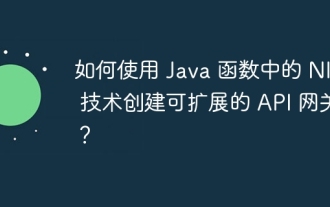
Answer: Using NIO technology you can create a scalable API gateway in Java functions to handle a large number of concurrent requests. Steps: Create NIOChannel, register event handler, accept connection, register data, read and write handler, process request, send response

JavaNIO API is an advanced API for handling I/O operations that provides better performance and scalability than traditional blocking I/O: Buffers: memory for transferring data between applications and the operating system area. Channels: Abstract concept that represents the connection between an application and an I/O device. Selectors: Used to poll multiple channels to determine which channels are ready for reading and writing.
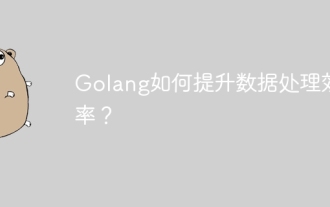
Golang improves data processing efficiency through concurrency, efficient memory management, native data structures and rich third-party libraries. Specific advantages include: Parallel processing: Coroutines support the execution of multiple tasks at the same time. Efficient memory management: The garbage collection mechanism automatically manages memory. Efficient data structures: Data structures such as slices, maps, and channels quickly access and process data. Third-party libraries: covering various data processing libraries such as fasthttp and x/text.
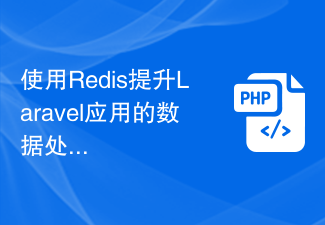
Use Redis to improve the data processing efficiency of Laravel applications. With the continuous development of Internet applications, data processing efficiency has become one of the focuses of developers. When developing applications based on the Laravel framework, we can use Redis to improve data processing efficiency and achieve fast access and caching of data. This article will introduce how to use Redis for data processing in Laravel applications and provide specific code examples. 1. Introduction to Redis Redis is a high-performance memory data
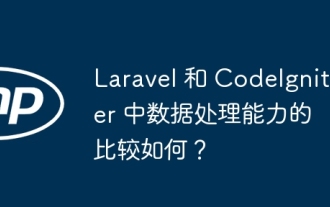
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
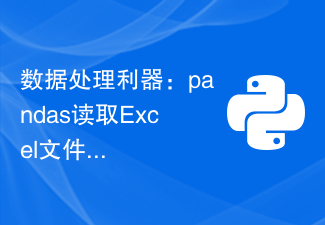
With the increasing popularity of data processing, more and more people are paying attention to how to use data efficiently and make the data work for themselves. In daily data processing, Excel tables are undoubtedly the most common data format. However, when a large amount of data needs to be processed, manually operating Excel will obviously become very time-consuming and laborious. Therefore, this article will introduce an efficient data processing tool - pandas, and how to use this tool to quickly read Excel files and perform data processing. 1. Introduction to pandas pandas
