


Detailed explanations and solutions to common problems with PHP functions
Common PHP function problems and solutions: Function is not defined: Check the existence of the function to ensure it is correctly defined or imported. Missing parameters: Supplement required parameters according to function declaration. Parameter type error: Confirm that the parameter type matches the declaration, and use conversion or validation to resolve the mismatch. Return type mismatch: Make sure the function return matches the declared type, modify the logic or return type. Function recursion infinite loop: Add explicit termination condition to prevent infinite loop.
Detailed explanations and solutions to common problems with PHP functions
As a widely used programming language, PHP provides a series of practical functions to handle various tasks. During use, you may encounter some common problems. Listed below are some common problems and their corresponding solutions, with practical cases to help you solve the problem easily.
1. Function is not defined
// 例子:使用未定义的函数 myFunction() myFunction(); // 触发错误
Solution:
Check whether the function exists in the code or in an external library . Make sure the function is correctly defined or imported.
2. The function is missing a required parameter
// 例子:函数需要一个参数,但调用时未提供 sendEmail(); // 触发错误
Solution:
Check the required parameters against the function declaration. If parameters are missing, provide the required parameters when calling.
3. Function parameter type error
// 例子:函数期望一个整数,但传递了一个字符串 average($value); // 触发错误
Solution:
Confirm the parameters passed to the function and the declared type match. Use casts or perform data validation to resolve mismatches.
4. Function return type mismatch
// 例子:函数声明为返回一个整数,但实际上返回一个字符串 function sum($a, $b): int { return "PHP"; // 触发错误 }
Solution:
Make sure the function return matches the declared type . Modify the function logic or return type to correct the mismatch.
5. Infinite loop occurs when function is called recursively
// 例子:函数递归调用自己,但没有明确的终止条件 function factorial($n) { return factorial($n - 1) * $n; }
Solution:
Add an explicit termination condition to prevent infinite loops. For example, the factorial function returns 1 when n equals 1.
Practical example:
Consider a function that counts the number of vowels in a text string:
function countVowels($text) { $vowels = ['a', 'e', 'i', 'o', 'u']; $count = 0; $text = strtolower($text); for ($i = 0; $i < strlen($text); $i++) { if (in_array($text[$i], $vowels)) { $count++; } } return $count; } echo countVowels("Hello, world!"); // 输出:3
The above is the detailed content of Detailed explanations and solutions to common problems with PHP functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


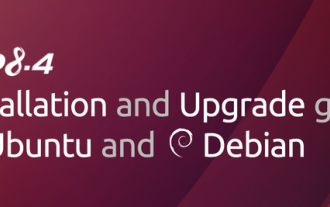
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
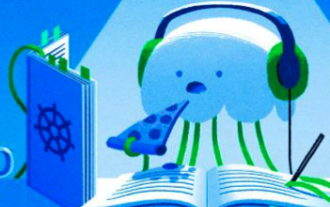
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
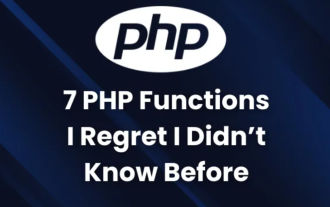
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
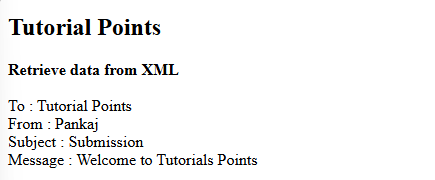
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
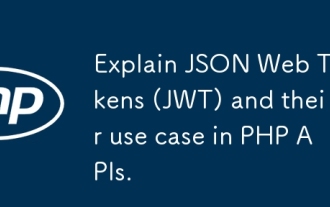
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
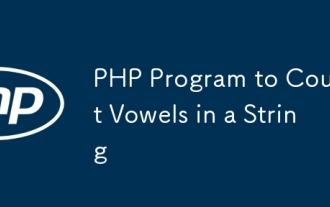
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
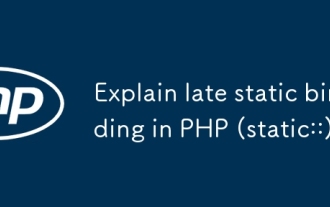
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
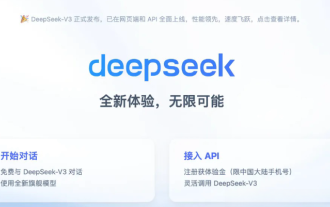
DeepSeekAI Tool User Guide and FAQ DeepSeek is a powerful AI intelligent tool. This article will answer some common usage questions to help you get started quickly. FAQ: The difference between different access methods: There is no difference in function between web version, App version and API calls, and App is just a wrapper for web version. The local deployment uses a distillation model, which is slightly inferior to the full version of DeepSeek-R1, but the 32-bit model theoretically has 90% full version capability. What is a tavern? SillyTavern is a front-end interface that requires calling the AI model through API or Ollama. What is breaking limit
