


Detailed explanation of C++ function debugging: How to debug problems in functions that contain exception handling?
C Debugging functions that contain exception handling uses exception point breakpoints to identify exception locations. Use the catch command in gdb to print exception information and stack traces. Use the exception logger to capture and analyze exceptions, including messages, stack traces, and variable values.
#Detailed explanation of C function debugging: debugging functions containing exception handling
Debugging functions containing exception handling in C needs to be done with caution , because exceptions can change the flow of function execution and can lead to errors that are difficult to track. Here are some effective ways to debug such functions:
Using exception point breakpoints
Exception point breakpoints can pause execution at a specific point where an exception is thrown or caught . This helps to find the source line of the exception and check the state of the variables at that time.
Using the catch command in gdb
The catch command in gdb allows catching and checking exception information when an exception occurs. It can be used to print exception messages, stack traces, and variable values.
Using the Exception Logger
The Exception Logger is a tool that captures and records exception information, including messages, stack traces, and variable values. This helps analyze the cause of an exception after it occurs.
Practical case: Debugging a function that throws std::out_of_range
exception
Suppose we have a function named get_element
A function that throws std::out_of_range
exception if the array index is exceeded:
int get_element(const int* arr, int size, int index) { if (index < 0 || index >= size) { throw std::out_of_range("Index out of range"); } return arr[index]; }
We can use exception point breakpoints to debug this function. Set a breakpoint where the exception occurs, such as in an if
statement. Run the program and set the index to a value beyond the row range. The breakpoint will trigger and we can inspect the variable value in the debugger to find out what caused the exception.
Also, we can also use the catch command in gdb:
(gdb) catch throw (gdb) r (gdb) catch throw (gdb) info locals
This will pause execution and print the exception message and variable value.
The above method helps to effectively debug C functions containing exception handling and find out the root cause of the error.
The above is the detailed content of Detailed explanation of C++ function debugging: How to debug problems in functions that contain exception handling?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


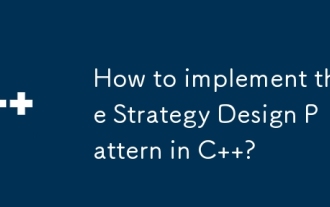
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
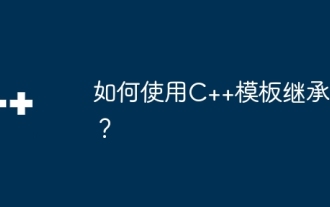
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
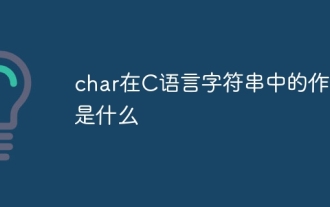
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
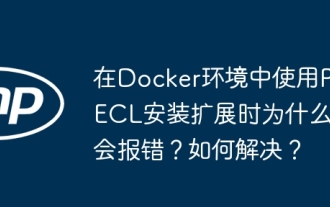
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
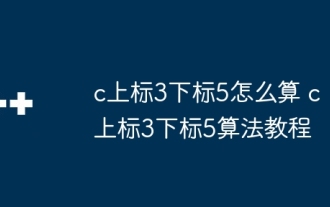
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
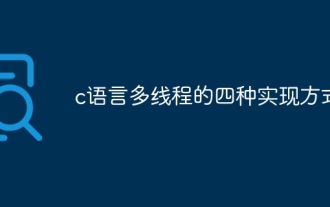
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
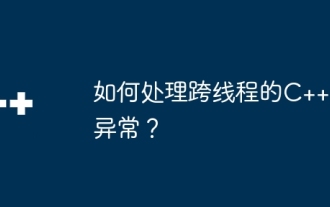
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
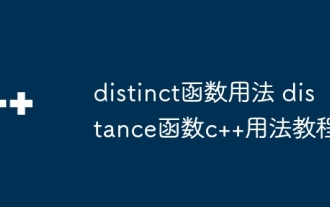
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
