


How does a Java function use NIO technology to handle high concurrent requests?
Java NIO is an efficient technology for handling high concurrent requests. It is implemented using non-blocking I/O and polling mechanisms: Create NIO Selector to listen for events; register Channel to Selector and listen for ACCEPT events; wait for events in a loop to process ACCEPT, READ and WRITE events; ACCEPT event handles client connection and creates SocketChannel; READ event reads data, and WRITE event writes data back.
Java function uses NIO to handle high concurrent requests
Introduction
Non-blocking I/O (NIO) is an efficient technology in Java for handling large numbers of concurrent requests. It uses asynchronous operations and polling mechanisms to effectively utilize system resources and improve system throughput.
Steps
1. Create NIO Selector
NIO Selector is used to listen to events on the registered Channel.
Selector selector = Selector.open();
2. Register Channel
Register ServerSocketChannel to Selector and listen to ACCEPT event.
ServerSocketChannel serverChannel = ServerSocketChannel.open(); serverChannel.configureBlocking(false); serverChannel.register(selector, SelectionKey.OP_ACCEPT);
3. Loop waiting for events
Listen to events through the Selector.select() method.
while (true) { selector.select(); Set<SelectionKey> keys = selector.selectedKeys(); // 处理事件... }
4. Handle the ACCEPT event
When the ACCEPT event occurs, accept the connection and create a SocketChannel.
if (key.isAcceptable()) { ServerSocketChannel channel = (ServerSocketChannel) key.channel(); SocketChannel clientChannel = channel.accept(); clientChannel.configureBlocking(false); clientChannel.register(selector, SelectionKey.OP_READ); }
Practical case
The following is a simple Java NIO Echo server example. It listens for client connections and echoes received messages.
EchoServer.java
import java.io.IOException; import java.net.InetSocketAddress; import java.nio.ByteBuffer; import java.nio.channels.SelectionKey; import java.nio.channels.Selector; import java.nio.channels.ServerSocketChannel; import java.nio.channels.SocketChannel; public class EchoServer { private Selector selector; private ServerSocketChannel serverChannel; private int port; public EchoServer(int port) { this.port = port; } public void start() throws IOException { // 创建 Selector selector = Selector.open(); // 创建 ServerSocketChannel serverChannel = ServerSocketChannel.open(); serverChannel.configureBlocking(false); serverChannel.bind(new InetSocketAddress(port)); serverChannel.register(selector, SelectionKey.OP_ACCEPT); // 不断循环等待事件 while (true) { int keysCount = selector.select(); if (keysCount == 0) { continue; } Set<SelectionKey> keys = selector.selectedKeys(); for (SelectionKey key : keys) { try { if (key.isAcceptable()) { handleAccept(key); } else if (key.isReadable()) { handleRead(key); } else if (key.isWritable()) { handleWrite(key); } } catch (IOException e) { e.printStackTrace(); key.cancel(); SocketChannel channel = (SocketChannel) key.channel(); channel.close(); } } keys.clear(); } } private void handleAccept(SelectionKey key) throws IOException { ServerSocketChannel channel = (ServerSocketChannel) key.channel(); SocketChannel clientChannel = channel.accept(); clientChannel.configureBlocking(false); clientChannel.register(selector, SelectionKey.OP_READ); } private void handleRead(SelectionKey key) throws IOException { SocketChannel channel = (SocketChannel) key.channel(); ByteBuffer buffer = ByteBuffer.allocate(1024); int readBytes = channel.read(buffer); if (readBytes == -1) { channel.close(); return; } buffer.flip(); channel.write(buffer); } private void handleWrite(SelectionKey key) throws IOException { SocketChannel channel = (SocketChannel) key.channel(); channel.write(ByteBuffer.allocate(1024)); } public static void main(String[] args) throws IOException { new EchoServer(9090).start(); } }
The above is the detailed content of How does a Java function use NIO technology to handle high concurrent requests?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


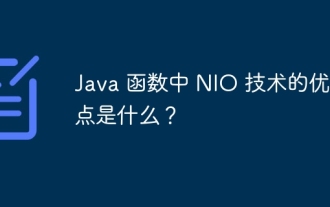
NIO (non-blocking IO) technology provides the advantages of high performance, scalability, low latency and low resource utilization in Java functions, but it also has higher complexity, the need for asynchronous programming, increased debugging difficulty, and system requirements. Higher disadvantages. In practice, NIO can optimize resource utilization and improve performance, such as when processing incoming HTTP requests.
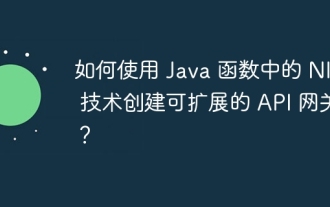
Answer: Using NIO technology you can create a scalable API gateway in Java functions to handle a large number of concurrent requests. Steps: Create NIOChannel, register event handler, accept connection, register data, read and write handler, process request, send response
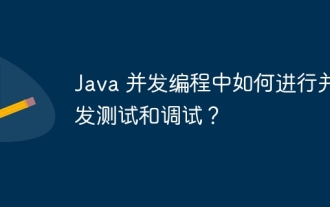
Concurrency testing and debugging Concurrency testing and debugging in Java concurrent programming are crucial and the following techniques are available: Concurrency testing: Unit testing: Isolate and test a single concurrent task. Integration testing: testing the interaction between multiple concurrent tasks. Load testing: Evaluate an application's performance and scalability under heavy load. Concurrency Debugging: Breakpoints: Pause thread execution and inspect variables or execute code. Logging: Record thread events and status. Stack trace: Identify the source of the exception. Visualization tools: Monitor thread activity and resource usage.
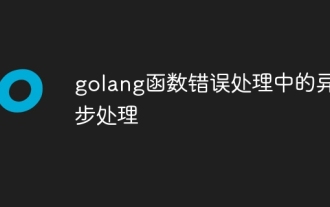
In Go functions, asynchronous error handling uses error channels to asynchronously pass errors from goroutines. The specific steps are as follows: Create an error channel. Start a goroutine to perform operations and send errors asynchronously. Use a select statement to receive errors from the channel. Handle errors asynchronously, such as printing or logging error messages. This approach improves the performance and scalability of concurrent code because error handling does not block the calling thread and execution can be canceled.
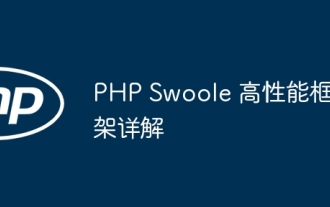
Swoole is a concurrency framework based on PHP coroutines, which has the advantages of high concurrency processing capabilities, low resource consumption, and simplified code development. Its main features include: coroutine concurrency, event-driven networks and concurrent data structures. By using the Swoole framework, developers can greatly improve the performance and throughput of web applications to meet the needs of high-concurrency scenarios.
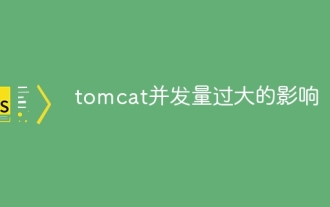
High concurrency in Tomcat leads to performance degradation and stability issues, including thread pool exhaustion, resource contention, deadlocks, and memory leaks. Mitigation measures include: adjusting thread pool settings, optimizing resource usage, monitoring server metrics, performing load testing, and using a load balancer.

Yes, nodejs can be used for large projects due to the following reasons: scalability, modularity, performance optimization, toolchain, and community support. Examples of large-scale projects using nodejs include PayPal, LinkedIn, Uber, Netflix, and Walmart.
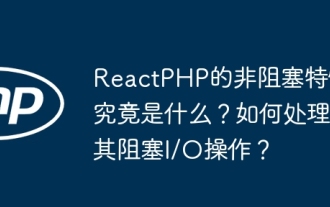
An official introduction to the non-blocking feature of ReactPHP in-depth interpretation of ReactPHP's non-blocking feature has aroused many developers' questions: "ReactPHPisnon-blockingbydefault...
