Integration of PHP functions and big data analysis
Use PHP functions and extensions to efficiently process and analyze big data. Integrate common PHP functions: perform tasks such as data transformation, aggregation, and grouping. Use extension libraries: Enhance PHP's big data processing capabilities, such as MongoDB PHP library and Elasticsearch PHP library. Practical case: By connecting to the database, retrieving data, using built-in functions to calculate the number of visits, and analyzing website traffic data.
Integration of PHP functions and big data analysis
With the continuous growth of big data, data analysis has become crucial . PHP's breadth makes it ideal for big data analysis, and it provides a variety of functions to simplify the process of working with large data sets.
Integration of commonly used PHP functions
PHP provides many out-of-the-box functions that can be used for tasks such as data transformation, aggregation, and grouping. Here are a few common examples:
// 将字符串转换为整数 $int = intval("123"); // 计算数组元素的和 $sum = array_sum([1, 2, 3]); // 根据字段分组数组 $grouped = array_column_multidimensional($array, 'column');
Using extension libraries
In addition to built-in functions, PHP has many libraries that can enhance its big data processing capabilities. Some popular choices include:
- MongoDB PHP Library: For interacting with the MongoDB database
- Elasticsearch PHP Library: For interacting with Elasticsearch search engine interaction
- Apache Hadoop PHP library:Used to interact with the Apache Hadoop distributed computing framework
Practical case: analyzing website traffic data
Let us consider a practical case where we will use PHP functions and extensions to analyze website traffic data.
<?php // 连接到数据库 $mongo = new MongoDB\Client(); $collection = $mongo->database->collection; // 检索流量数据 $cursor = $collection->find([], ['limit' => 10000]); // 转换数据格式 $rows = []; foreach ($cursor as $row) { $rows[] = [$row['timestamp'], $row['url'], $row['user_agent'], $row['ip_address']]; } // 计算每个 URL 的访问次数 $counts = array_count_values(array_column($rows, 1)); // 打印结果 print_r($counts); ?>
This script uses the MongoDB PHP library to connect to a MongoDB database, retrieve traffic data and convert it into an array. It then counts the number of visits to each URL using the built-in array_count_values()
function.
Conclusion
By integrating PHP functions and big data analytics, you can create powerful solutions to efficiently process and analyze large data sets. With extended libraries and practical examples, you can easily build applications to gain valuable insights and make informed decisions.
The above is the detailed content of Integration of PHP functions and big data analysis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
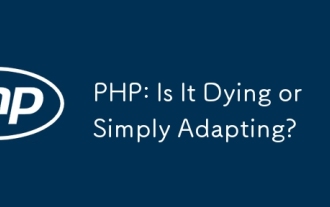
PHP is not dying, but constantly adapting and evolving. 1) PHP has undergone multiple version iterations since 1994 to adapt to new technology trends. 2) It is currently widely used in e-commerce, content management systems and other fields. 3) PHP8 introduces JIT compiler and other functions to improve performance and modernization. 4) Use OPcache and follow PSR-12 standards to optimize performance and code quality.
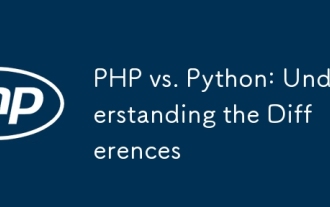
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
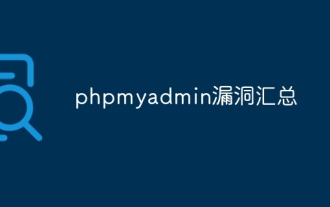
The key to PHPMyAdmin security defense strategy is: 1. Use the latest version of PHPMyAdmin and regularly update PHP and MySQL; 2. Strictly control access rights, use .htaccess or web server access control; 3. Enable strong password and two-factor authentication; 4. Back up the database regularly; 5. Carefully check the configuration files to avoid exposing sensitive information; 6. Use Web Application Firewall (WAF); 7. Carry out security audits. These measures can effectively reduce the security risks caused by PHPMyAdmin due to improper configuration, over-old version or environmental security risks, and ensure the security of the database.

The tools and frameworks that need to be mastered in H5 development include Vue.js, React and Webpack. 1.Vue.js is suitable for building user interfaces and supports component development. 2.React optimizes page rendering through virtual DOM, suitable for complex applications. 3.Webpack is used for module packaging and optimize resource loading.

To become proficient when using Composer, you need to master the following skills: 1. Proficient in using composer.json and composer.lock files, 2. Understand how Composer works, 3. Master Composer's command line tools, 4. Understand basic and advanced usage, 5. Familiar with common errors and debugging techniques, 6. Optimize usage and follow best practices.

The PHP community provides rich resources and support to help developers grow. 1) Resources include official documentation, tutorials, blogs and open source projects such as Laravel and Symfony. 2) Support can be obtained through StackOverflow, Reddit and Slack channels. 3) Development trends can be learned by following RFC. 4) Integration into the community can be achieved through active participation, contribution to code and learning sharing.

Apache is the basis of many websites because of its stability, reliability and highly configurable. 1.Apache is developed by the Apache Software Foundation, supports a variety of operating systems and provides static and dynamic content services. 2. Its core functions include handling HTTP requests, virtual hosting and modular design. 3. Configuration examples from basic settings to advanced virtual hosts and URL rewrites. 4. Common errors such as permissions, syntax and module loading problems can be solved through corresponding debugging techniques. 5. Performance optimization includes tuning parameters, using cache and load balancing, and following best practices can improve server efficiency and security.
