


Design and implementation of function cache in golang concurrency mode
In order to implement function caching in Go's concurrent environment, you can follow the following steps: Define a Cache interface containing Get and Set methods. Use sync.Map to implement a syncMapCache structure, which implements the Cache interface and stores cache data. Register cache handling functions for different functions. Using sync.MapCache, you can cache function calculation results, such as the Fibonacci sequence, to effectively improve program performance.
Go function cache design and implementation in concurrent mode
In Go's concurrent programming environment, it is often necessary to cache some calculations results to improve performance. By saving the results of repeated calculations in the cache, unnecessary repeated calculations can be avoided, thereby significantly improving program running efficiency.
Goroutine Safe Function Cache
In order to implement safe function caching in concurrent mode, you can use the sync.Map
type. sync.Map
is a concurrency-safe key-value mapping, which can ensure that data competition problems will not occur during concurrent access.
Design
The design of function cache consists of the following steps:
- Define a
Cache
interface, which contains AGet
method and aSet
method of function type. - Implement a
sync.MapCache
structure, which implements theCache
interface and usessync.Map
to internally store cache data. - Register cache handling functions for different functions.
Implementation
The following is the implementation of sync.MapCache
:
import ( "sync" "github.com/golang/sync/syncmap" ) type Cache interface { Get(key interface{}) (interface{}, bool) Set(key, value interface{}) } type syncMapCache struct { syncmap.Map } func (c *syncMapCache) Get(key interface{}) (interface{}, bool) { return c.Load(key) } func (c *syncMapCache) Set(key, value interface{}) { c.Store(key, value) }
Practical case
The following is an example of using syncMapCache
to cache the results of a Fibonacci sequence calculation:
package main import ( "fmt" "math/big" "github.com/fatih/structs" ) type fibonacciKey struct { n int } func (k fibonacciKey) String() string { return structs.Name(k) } var fibCache = &syncMapCache{} func fibonacci(n int) *big.Int { if n <= 1 { return big.NewInt(int64(n)) } key := fibonacciKey{n} if fib, ok := fibCache.Get(key); ok { return fib.(*big.Int) } fib := fibonacci(n-1).Add(fibonacci(n-2), nil) fibCache.Set(key, fib) return fib } func main() { for i := 0; i < 10; i++ { fmt.Println(fibonacci(i)) } }
Summary
Passed Using the sync.Map
type of concurrency safety features, a reliable and efficient function cache can be implemented. This helps avoid unnecessary duplication of calculations, thereby improving program performance in concurrent mode.
The above is the detailed content of Design and implementation of function cache in golang concurrency mode. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


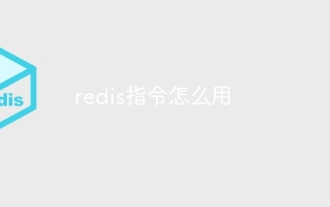
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
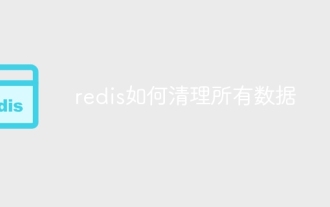
How to clean all Redis data: Redis 2.8 and later: The FLUSHALL command deletes all key-value pairs. Redis 2.6 and earlier: Use the DEL command to delete keys one by one or use the Redis client to delete methods. Alternative: Restart the Redis service (use with caution), or use the Redis client (such as flushall() or flushdb()).
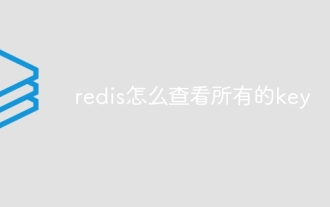
To view all keys in Redis, there are three ways: use the KEYS command to return all keys that match the specified pattern; use the SCAN command to iterate over the keys and return a set of keys; use the INFO command to get the total number of keys.

Redis uses hash tables to store data and supports data structures such as strings, lists, hash tables, collections and ordered collections. Redis persists data through snapshots (RDB) and append write-only (AOF) mechanisms. Redis uses master-slave replication to improve data availability. Redis uses a single-threaded event loop to handle connections and commands to ensure data atomicity and consistency. Redis sets the expiration time for the key and uses the lazy delete mechanism to delete the expiration key.
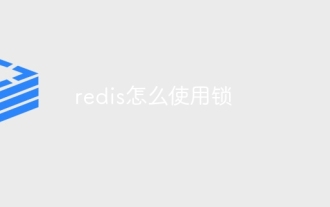
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
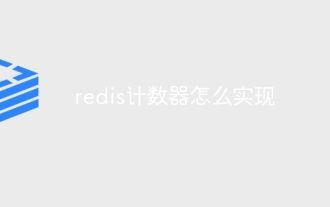
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.

Use of zset in Redis cluster: zset is an ordered collection that associates elements with scores. Sharding strategy: a. Hash sharding: Distribute the hash value according to the zset key. b. Range sharding: divide into ranges according to element scores, and assign each range to different nodes. Read and write operations: a. Read operations: If the zset key belongs to the shard of the current node, it will be processed locally; otherwise, it will be routed to the corresponding shard. b. Write operation: Always routed to shards holding the zset key.
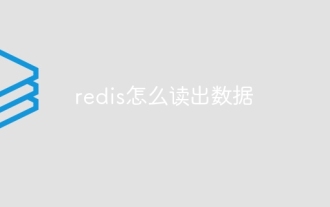
To read data from Redis, you can follow these steps: 1. Connect to the Redis server; 2. Use get(key) to get the value of the key; 3. If you need string values, decode the binary value; 4. Use exists(key) to check whether the key exists; 5. Use mget(keys) to get multiple values; 6. Use type(key) to get the data type; 7. Redis has other read commands, such as: getting all keys in a matching pattern, using cursors to iterate the keys, and sorting the key values.
