The role of iterators in java
Iterator is a Java design pattern used to traverse collection elements. It allows accessing and moving to elements, performing operations such as deletion and reset. There are three main iterator types in Java: Iterator, ListIterator and Enumeration. Using an iterator requires getting its instance, then iterating over the elements one by one, getting the value, deleting the element and resetting the pointer, which can be used to traverse arrays and collections.
The role of iterators in Java
Overview
Iteration Converter is a design pattern in Java that allows traversing collections and arrays in a structured manner. It is essentially a pointer that points to the next element in the collection and provides methods to access and move to that element.
Function
Iterators are mainly used for the following purposes in Java:
- Traverse a collection: Use iteration The operator can iterate through all elements in the collection one by one.
-
Get the element value: Use the
next()
method of the iterator to get the element value pointed to by the current pointer. - Deleting elements: Some iterators allow deletion of the element pointed to by the current pointer.
-
Reset iteration: Use the iterator's
reset()
method to reset the iterator pointer to the beginning of the collection.
Types
Java provides three main iterator types:
- Iterator: A general-purpose iterator that traverses all elements in a collection or array.
- ListIterator: Extends Iterator to allow bidirectional traversal and insertion of elements.
- Enumeration: An obsolete iterator type used for traversing enumeration or legacy collection classes.
Using
When using iterators, you usually follow these steps:
- Get an iterator for a collection or array.
- Use the
hasNext()
method to check if there are more elements. - Use the
next()
method to get the value of the current element. - Use the
remove()
method to remove elements as needed. - Use the
reset()
method to reset the iterator pointer.
Example
Consider the following example of traversing an array:
// 创建一个数组 int[] numbers = {1, 2, 3, 4, 5}; // 获取数组的迭代器 Iterator<Integer> iterator = Arrays.stream(numbers).iterator(); // 遍历数组并打印元素 while (iterator.hasNext()) { System.out.println(iterator.next()); }
Output:
<code>1 2 3 4 5</code>
The above is the detailed content of The role of iterators in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
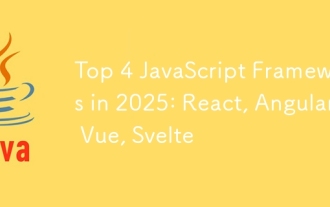
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
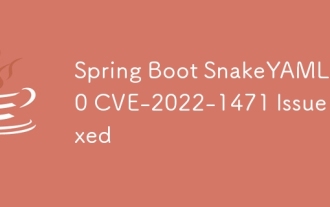
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
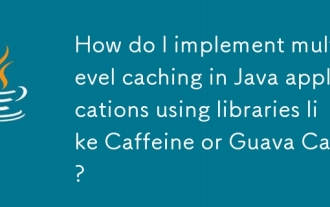
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
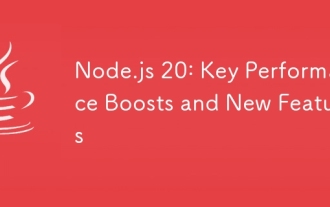
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
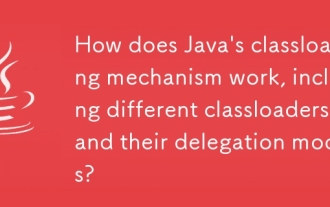
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
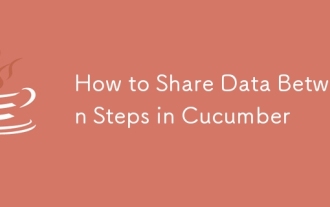
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
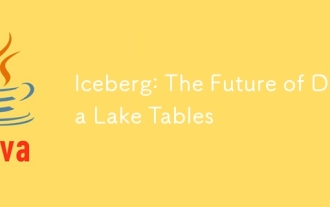
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
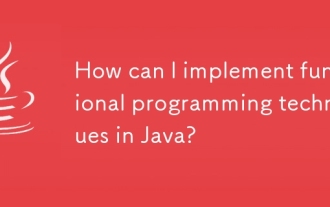
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
