How to call classes in java
Calling class methods in Java: 1. Create an instance of the class; 2. Use the dot operator of the instance method; 3. Call the static method directly; 4. Pass the necessary parameters.
Methods called by classes in Java
Calling methods of classes in Java is very simple, just follow the following Steps:
-
Create an instance of the class: Use the
new
keyword to create an instance of the class, for example:
Person person = new Person();
- Calling instance methods: Use the dot operator (.`) to call instance methods of a class, for example:
person.setName("John Doe");
- Calling static methods: If the method is declared static, you can call it directly using the class name, for example:
Person.printStaticMessage();
- Pass parameters: If the method requires parameters, you can put them in parentheses Pass them, for example:
person.greet("Hello!");
Example:
Here is an example showing how to call the class:
class Person { private String name; public void setName(String name) { this.name = name; } public void greet(String message) { System.out.println(message + ", " + name + "!"); } public static void printStaticMessage() { System.out.println("This is a static message."); } } public class Main { public static void main(String[] args) { Person person = new Person(); person.setName("John Doe"); person.greet("Hello"); Person.printStaticMessage(); } }
Output:
<code>Hello, John Doe! This is a static message.</code>
The above is the detailed content of How to call classes in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
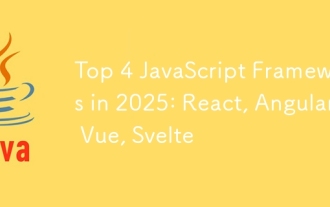
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
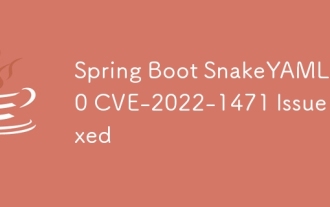
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
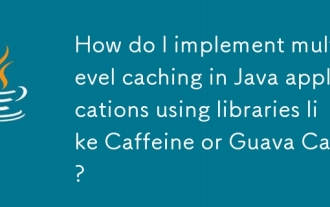
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
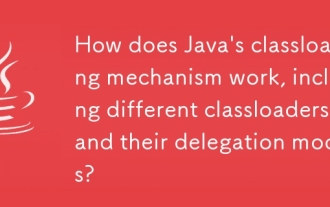
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
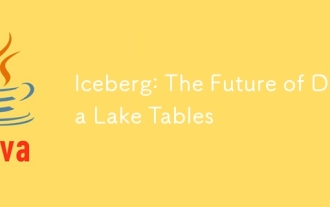
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
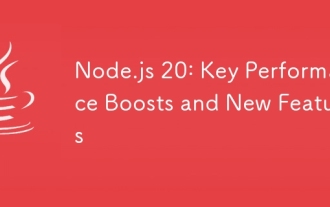
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
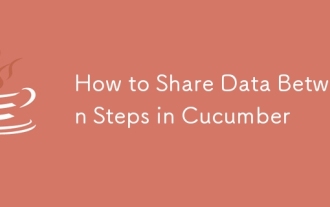
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
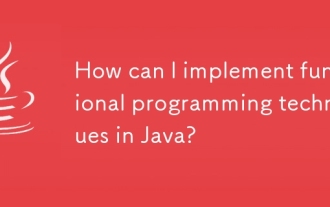
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
