The difference between % and / in C language
May 02, 2024 pm 05:24 PMIn C language, % is the modulo operator, which returns the remainder of the division of two integer values; / is the division operator, which returns the quotient between two values, even if the operand is an integer. Floating point value. The key differences are the result type (% is integer, / is floating point), operand type (% is limited to integer, / can be integer or floating point), and purpose (% calculates remainder, / calculates quotient).
The difference between % and / in C language
In C language,%
and /
are two different operators with different functions and uses.
%
Modulo operator
%
The operator is called the modulo operator. It calculates the remainder of two integer values divided by another integer value. For example:
int a = 7; int b = 3; int remainder = a % b; // remainder = 1
/
Division operator
##- operator is called the division operator. It calculates the quotient between two values. In C, the division operator always returns a floating-point value, even if the operands are both integers. For example:
int a = 7; int b = 3; float quotient = a / b; // quotient = 2.333333
Key Differences
The following are the key differences between the% and
/ operators:
- Result type: %
returns an integer value, while
/returns a floating point value.
- Operand type: %
can only be used for integer values, while
/can be used for integer and floating point values.
- Uses: %
Used to calculate remainders, such as when calculating array element indexes or checking parity.
/Used to calculate quotients, such as when calculating averages or proportions.
Example
Calculate the remainder:
int sum = 10; int numElements = 3; int remainder = sum % numElements; // remainder = 1
Calculate the quotient:
float area = 12.5; float length = 5.0; float width = area / length; // width = 2.5
The above is the detailed content of The difference between % and / in C language. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
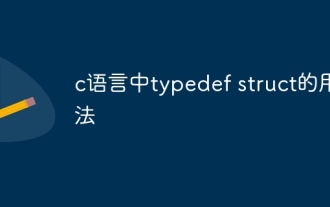
Usage of typedef struct in c language
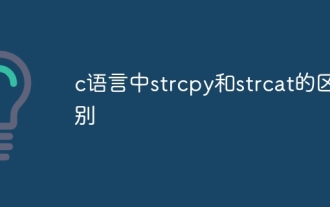
The difference between strcpy and strcat in c language
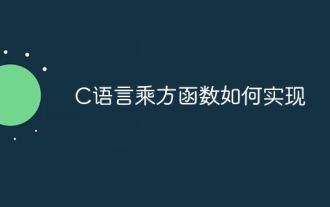
How to implement the power function in C language
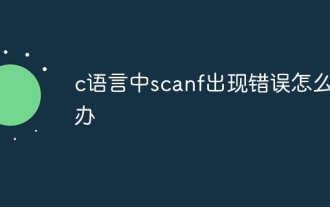
What to do if there is an error in scanf in C language
