


Detailed explanation of C++ function inheritance: How to use inheritance to implement pluggable architecture?
Function inheritance allows derived classes to override base class functions to avoid code duplication. Implementation method: Use the override keyword before derived class functions. Practical case: In the plug-in architecture, the plug-in class serves as the base class, and the derived class provides plug-in implementation. The plug-in is dynamically loaded and run through the PluginManager class.
C Detailed explanation of function inheritance: a powerful tool for pluggable architecture
Overview of function inheritance
Function inheritance allows you to inherit in derived classes Base class functions to avoid duplication of code. The functions of the derived class will override the functions of the base class, which means that the functions of the derived class will be called at runtime instead of the functions of the base class.
How to use function inheritance
To implement function inheritance, you need to use the override
keyword in the class definition of the derived class to override the functions of the base class. For example:
class Base { public: virtual void print() { std::cout << "Base class print" << std::endl; } }; class Derived : public Base { public: virtual void print() override { std::cout << "Derived class print" << std::endl; } };
Practical case: pluggable architecture
Function inheritance is very useful when creating a pluggable architecture. In a pluggable architecture, you can load and unload different modules or components at runtime. This is useful when you need to dynamically change application functionality or provide customizable extensions.
The following is an example of using function inheritance to implement a pluggable architecture:
class Plugin { public: virtual void init() = 0; virtual void run() = 0; virtual void terminate() = 0; }; class PluginA : public Plugin { public: void init() override {} void run() override { std::cout << "Plugin A is running" << std::endl; } void terminate() override {} }; class PluginB : public Plugin { public: void init() override {} void run() override { std::cout << "Plugin B is running" << std::endl; } void terminate() override {} }; class PluginManager { public: std::vector<std::unique_ptr<Plugin>> plugins; void loadPlugin(std::unique_ptr<Plugin> plugin) { plugins.push_back(std::move(plugin)); } void runPlugins() { for (auto& plugin : plugins) { plugin->run(); } } };
In this example, the Plugin
class acts as a base class and defines the interface of the plug-in ( init()
, run()
, terminate()
). PluginA
and PluginB
are derived classes that provide the actual plug-in implementation. PluginManager
The class is responsible for managing plug-ins and allows dynamic loading and running of plug-ins.
The above is the detailed content of Detailed explanation of C++ function inheritance: How to use inheritance to implement pluggable architecture?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
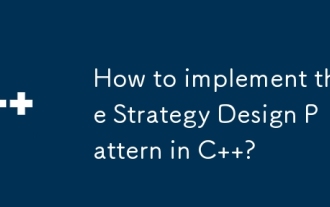
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
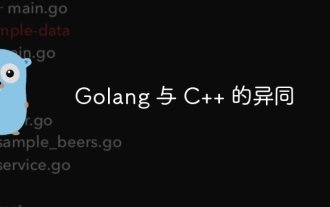
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
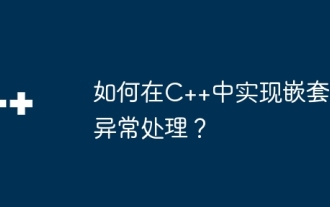
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
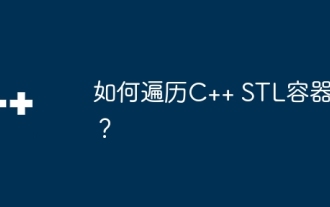
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
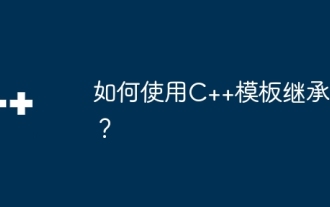
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
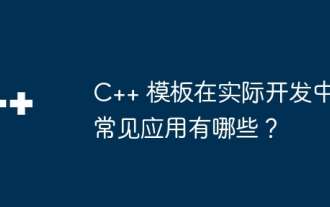
C++ templates are widely used in actual development, including container class templates, algorithm templates, generic function templates and metaprogramming templates. For example, a generic sorting algorithm can sort arrays of different types of data.
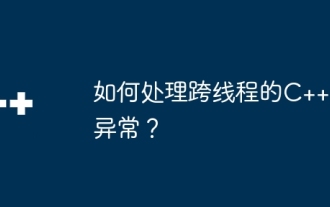
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
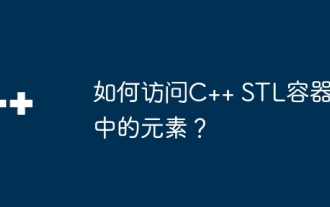
How to access elements in C++ STL container? There are several ways to do this: Traverse a container: Use an iterator Range-based for loop to access specific elements: Use an index (subscript operator []) Use a key (std::map or std::unordered_map)
