


Detailed explanation of C++ function recursion: recursive optimization techniques
Function recursion is when a function calls itself, providing an effective way to solve complex problems by decomposing the problem into sub-problems. It is crucial to optimize recursion to avoid stack overflow. Common optimization techniques include: limiting recursion depth, using tail recursion optimization, using memos to avoid repeated calculations
C Detailed explanation of function recursion: recursion optimization techniques
What is function recursion?
Function recursion refers to the process of the function itself calling itself. Recursion provides an efficient way to solve complex problems by breaking a problem into smaller sub-problems.
Recursive Optimization Tips
When using recursion to solve problems, optimization is crucial to avoid stack overflows and other efficiency issues. Here are some common optimization tips:
- Limit recursion depth: In recursive functions, set the maximum recursion depth to prevent infinite recursion.
- Use tail recursion optimization: Tail recursion means that the function performs a recursive call on the last line. The compiler can optimize tail recursion and convert it into iteration, improving efficiency.
- Using memos: Memories are a data structure used to store the results of previous calculations. It allows recursive functions to avoid repeated calculations on repeated subproblems.
Practical case
Fibonacci sequence
The Fibonacci sequence is a sequence of integers. where each number is the sum of the previous two numbers. We can calculate the numbers in the Fibonacci sequence using a recursive function as follows:
int fibonacci(int n) { if (n <= 1) { return n; } else { return fibonacci(n - 1) + fibonacci(n - 2); } }
Optimized Fibonacci Sequence Function
Optimize using memo Fibonacci sequence function, we can significantly improve its efficiency:
int fibonacci(int n, vector<int>& memo) { if (n <= 1) { return n; } else if (memo[n] != -1) { return memo[n]; } else { memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo); return memo[n]; } }
Here, the memo memo is used to store the calculated values of the Fibonacci sequence. When the function is called again with the same parameters, it returns the stored value, avoiding double calculations.
Conclusion
Functional recursion is a powerful tool that can be used to solve a variety of problems. By understanding recursive optimization techniques and using them in real-world cases, you can significantly improve the efficiency and performance of your code.
The above is the detailed content of Detailed explanation of C++ function recursion: recursive optimization techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
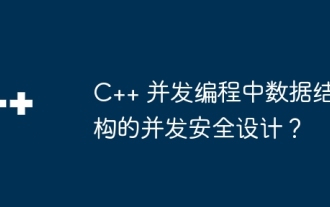
In C++ concurrent programming, the concurrency-safe design of data structures is crucial: Critical section: Use a mutex lock to create a code block that allows only one thread to execute at the same time. Read-write lock: allows multiple threads to read at the same time, but only one thread to write at the same time. Lock-free data structures: Use atomic operations to achieve concurrency safety without locks. Practical case: Thread-safe queue: Use critical sections to protect queue operations and achieve thread safety.

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
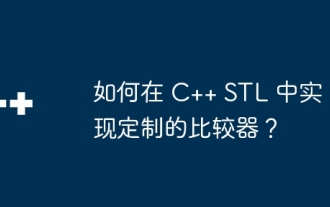
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
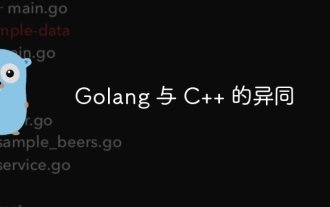
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
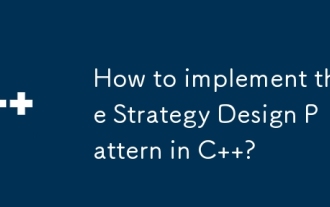
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
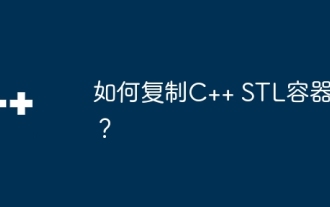
There are three ways to copy a C++ STL container: Use the copy constructor to copy the contents of the container to a new container. Use the assignment operator to copy the contents of the container to the target container. Use the std::copy algorithm to copy the elements in the container.
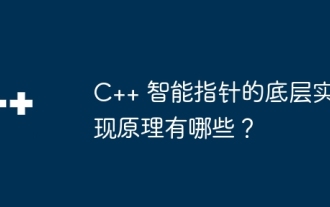
C++ smart pointers implement automatic memory management through pointer counting, destructors, and virtual function tables. The pointer count keeps track of the number of references, and when the number of references drops to 0, the destructor releases the original pointer. Virtual function tables enable polymorphism, allowing specific behaviors to be implemented for different types of smart pointers.
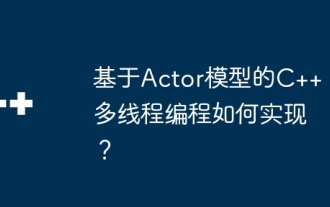
C++ multi-threaded programming implementation based on the Actor model: Create an Actor class that represents an independent entity. Set the message queue where messages are stored. Defines the method for an Actor to receive and process messages from the queue. Create Actor objects and start threads to run them. Send messages to Actors via the message queue. This approach provides high concurrency, scalability, and isolation, making it ideal for applications that need to handle large numbers of parallel tasks.
