What are the advantages of recursive calls in Java functions?
Answer: The advantages of using recursive calls to Java functions include: clarity and conciseness, efficiency, maintainability, simple modeling and practical cases. Clear and concise: Recursive code is simpler and easier to understand than iterative methods, reducing the level of code nesting. Efficient: In some cases, recursion is more efficient than iteration because the overhead of creating and destroying new function calls is eliminated. Maintainability: Recursive code is easier to maintain than code using loops because recursive methods have clear termination conditions. Simple modeling: Recursion provides a natural way to model problems with a recursive structure. Practical case: The factorial evaluation function demonstrates the implementation and advantages of recursion.
Advantages of recursive calls in Java functions
Recursion is a programming technique that allows functions to call themselves to solve problems . It is particularly useful when solving problems with nested structures or self-similar properties. In Java, recursion can be achieved by overloading a function and passing decreasing parameter values.
Advantages:
- Clear and concise:Recursive code is usually more concise and easier to understand than iterative methods. This is particularly useful for solving complex problems because they can reduce the nesting level of your code.
- Efficient: In some cases, recursion can be more efficient than iteration. This is because the recursion calls itself directly, eliminating the overhead of creating and destroying new function calls.
- Maintainability: Recursive code is generally easier to maintain than code that uses loops or other iterative methods. This is because recursive methods have a clear termination condition, making the code easier to understand and debug.
- Simple problem modeling: Recursion provides a way to model problems with a recursive structure in a natural way. This is because recursive functions can be broken down into smaller sub-problems like the problem itself.
Practical case:
The following is a recursive function implemented in Java for calculating factorial:
public class Factorial { public static int calculateFactorial(int n) { if (n == 0) { return 1; } else { return n * calculateFactorial(n - 1); } } public static void main(String[] args) { int result = calculateFactorial(5); System.out.println("5 factorial is: " + result); // 输出: 5 factorial is: 120 } }
In this example , calculateFactorial()
The function takes a non-negative integer as a parameter and returns its factorial. The function solves the problem by calling itself, decrementing the parameter value n
with each recursive call until the termination condition is reached (n == 0
).
The above is the detailed content of What are the advantages of recursive calls in Java functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


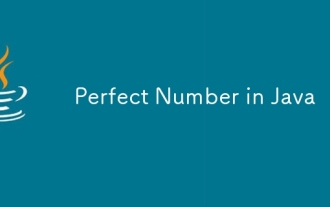
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
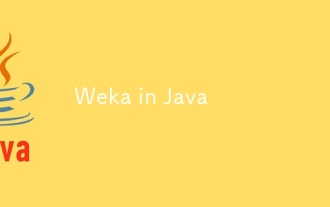
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
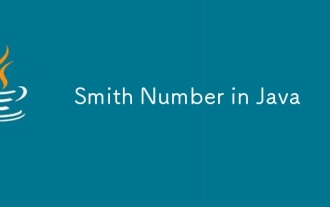
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
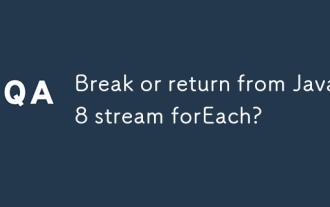
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
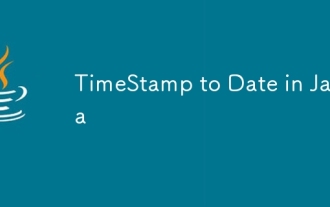
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
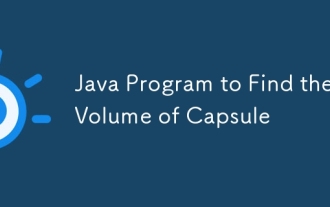
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
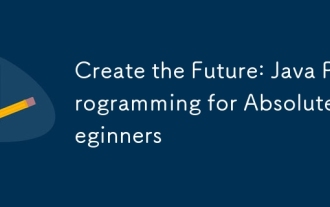
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
