A guide to unit testing Go concurrent functions
Unit testing concurrent functions is critical as this helps ensure their correct behavior in a concurrent environment. Fundamental principles such as mutual exclusion, synchronization, and isolation must be considered when testing concurrent functions. Concurrent functions can be unit tested by simulating, testing race conditions, and verifying results.
Unit Testing Guide for Go Concurrent Functions
In the Go language, writing concurrent code usually involves multiple goroutines and shared state. Unit testing concurrent functions is critical as this helps ensure correct behavior in a concurrent environment.
Basic principles of concurrent mode
When testing concurrent functions, you need to consider the following basic principles:
- Mutual exclusion:Ensure Access to shared state is protected against race conditions.
- Synchronization: Coordinate the interaction between goroutines to ensure data integrity and consistency.
- Isolation: When testing concurrent functions, you need to ensure that they are isolated from each other and do not affect each other.
Unit testing concurrent functions
You can use the following methods to unit test concurrent functions:
- Simulation: Simulate other goroutines behavior that allows unit testing of concurrent interactions.
- Test for race conditions: Use stress testing or concurrent runs to test how your code behaves under race conditions.
- Verify results: Check the results of concurrent functions to ensure that their expected behavior is consistent with the actual behavior.
Practical Case
The following code example shows how to use the Go test package to unit test concurrent functions:
import ( "testing" "time" ) func TestConcurrentFunction(t *testing.T) { // 模拟其他 goroutine 的行为 done := make(chan bool) go func() { time.Sleep(100 * time.Millisecond) done <- true }() // 并发调用待测函数 result, err := concurrentFunction() // 等待模拟 goroutine 完成 <-done // 验证结果 if err != nil { t.Errorf("concurrentFunction() returned an error: %v", err) } if result != "success" { t.Errorf("concurrentFunction() did not return the expected result") } } func concurrentFunction() (string, error) { // 模拟并发访问共享状态 lock.Lock() defer lock.Unlock() value := 100 value++ return "success", nil }
The above is the detailed content of A guide to unit testing Go concurrent functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
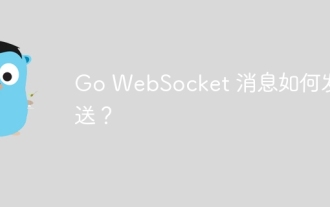
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
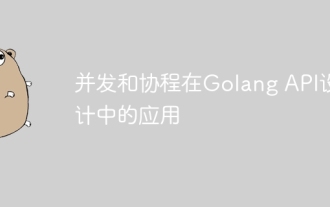
Concurrency and coroutines are used in GoAPI design for: High-performance processing: Processing multiple requests simultaneously to improve performance. Asynchronous processing: Use coroutines to process tasks (such as sending emails) asynchronously, releasing the main thread. Stream processing: Use coroutines to efficiently process data streams (such as database reads).
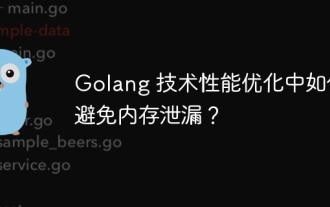
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
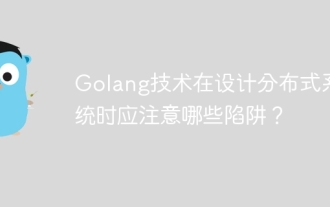
Pitfalls in Go Language When Designing Distributed Systems Go is a popular language used for developing distributed systems. However, there are some pitfalls to be aware of when using Go, which can undermine the robustness, performance, and correctness of your system. This article will explore some common pitfalls and provide practical examples on how to avoid them. 1. Overuse of concurrency Go is a concurrency language that encourages developers to use goroutines to increase parallelism. However, excessive use of concurrency can lead to system instability because too many goroutines compete for resources and cause context switching overhead. Practical case: Excessive use of concurrency leads to service response delays and resource competition, which manifests as high CPU utilization and high garbage collection overhead.
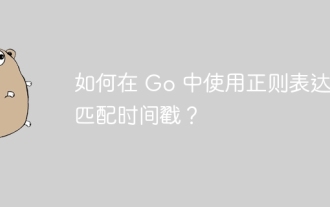
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
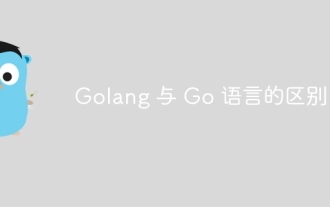
Go and the Go language are different entities with different characteristics. Go (also known as Golang) is known for its concurrency, fast compilation speed, memory management, and cross-platform advantages. Disadvantages of the Go language include a less rich ecosystem than other languages, a stricter syntax, and a lack of dynamic typing.
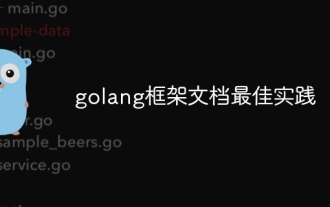
Writing clear and comprehensive documentation is crucial for the Golang framework. Best practices include following an established documentation style, such as Google's Go Coding Style Guide. Use a clear organizational structure, including headings, subheadings, and lists, and provide navigation. Provides comprehensive and accurate information, including getting started guides, API references, and concepts. Use code examples to illustrate concepts and usage. Keep documentation updated, track changes and document new features. Provide support and community resources such as GitHub issues and forums. Create practical examples, such as API documentation.
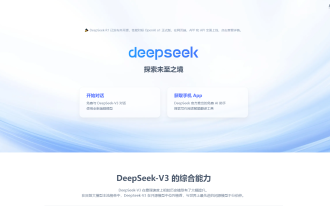
DeepSeek: How to deal with the popular AI that is congested with servers? As a hot AI in 2025, DeepSeek is free and open source and has a performance comparable to the official version of OpenAIo1, which shows its popularity. However, high concurrency also brings the problem of server busyness. This article will analyze the reasons and provide coping strategies. DeepSeek web version entrance: https://www.deepseek.com/DeepSeek server busy reason: High concurrent access: DeepSeek's free and powerful features attract a large number of users to use at the same time, resulting in excessive server load. Cyber Attack: It is reported that DeepSeek has an impact on the US financial industry.
