FAQ about C++ function return values: types and meanings
C The function return value type can be any valid data type, including built-in types, user-defined types or void. If not explicitly specified, the return type is int. The void function does not return any value, but it can modify the program state. Local variables cannot be returned, but their data location can be returned using a pointer or reference. Standard C does not support returning multiple values directly, but it can be achieved through structure, reference or pointer parameters.
FAQ about C function return values: Types and meaning
In C, the function return value is when the function is defined The specified data type. It represents the value returned to the caller when the function is executed. Although this is a simple concept, there are several issues that often plague developers.
1. Can the return type be any data type?
Yes, the return type can be any valid C data type, including built-in types (such as int, float, bool, etc.), user-defined types (such as structures, classes), and even void.
2. What if the function does not explicitly specify the return value type?
You can explicitly return the return value of a function through the return statement. If not explicitly specified, the function's return type is int and returns the value assigned to it at the point of call.
3. What is a void function?
void function is a function that does not return any value. They are typically used to perform operations that modify the state of the program, such as printing output or updating variables.
4. Can local variables be returned?
No, functions cannot return local variables. Local variables will be destroyed after they go out of function scope. Instead, you can use a pointer or reference to return the data location pointed to by a local variable.
5. How to return multiple values?
Standard C does not provide a method to directly return multiple values. Common solutions are to use a structure or class to encapsulate multiple values, or to modify caller-supplied variables via reference or pointer parameters.
Practical case:
The following code shows how to define functions of various return types:
// 返回整型的函数 int add(int a, int b) { return a + b; } // 返回浮点型的函数 float divide(float a, float b) { return a / b; } // 返回结构的函数 struct Point { int x; int y; }; Point createPoint(int x, int y) { return Point{x, y}; } // void 函数 void printMessage(const char* message) { std::cout << message << std::endl; }
The above is the detailed content of FAQ about C++ function return values: types and meanings. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


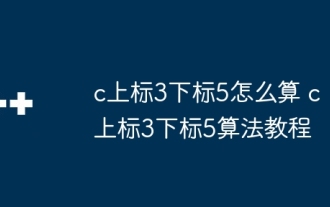
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
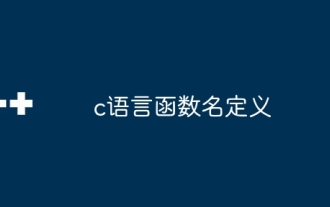
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
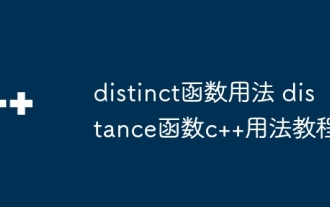
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
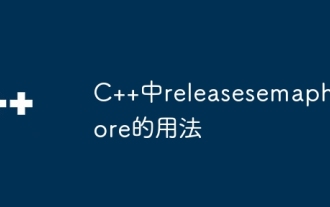
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
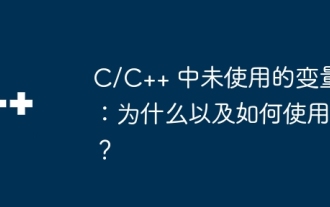
In C/C code review, there are often cases where variables are not used. This article will explore common reasons for unused variables and explain how to get the compiler to issue warnings and how to suppress specific warnings. Causes of unused variables There are many reasons for unused variables in the code: code flaws or errors: The most direct reason is that there are problems with the code itself, and the variables may not be needed at all, or they are needed but not used correctly. Code refactoring: During the software development process, the code will be continuously modified and refactored, and some once important variables may be left behind and unused. Reserved variables: Developers may predeclare some variables for future use, but they will not be used in the end. Conditional compilation: Some variables may only be under specific conditions (such as debug mode)
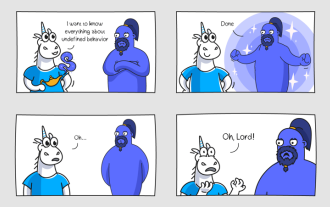
Exploring Undefined Behaviors in C Programming: A Detailed Guide This article introduces an e-book on Undefined Behaviors in C Programming, a total of 12 chapters covering some of the most difficult and lesser-known aspects of C Programming. This book is not an introductory textbook for C language, but is aimed at readers familiar with C language programming, and explores in-depth various situations and potential consequences of undefined behaviors. Author DmitrySviridkin, editor Andrey Karpov. After six months of careful preparation, this e-book finally met with readers. Printed versions will also be launched in the future. This book was originally planned to include 11 chapters, but during the creation process, the content was continuously enriched and finally expanded to 12 chapters - this itself is a classic array out-of-bounds case, and it can be said to be every C programmer
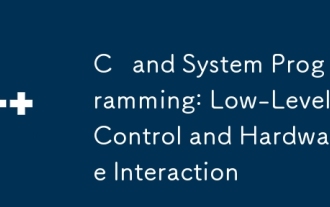
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
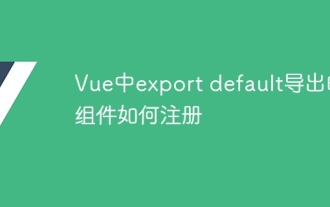
Question: How to register a Vue component exported through export default? Answer: There are three registration methods: Global registration: Use the Vue.component() method to register as a global component. Local Registration: Register in the components option, available only in the current component and its subcomponents. Dynamic registration: Use the Vue.component() method to register after the component is loaded.
