Atomic operations in C++ memory management
Atomic operations are crucial in managing shared memory in a multi-threaded environment, ensuring that accesses to memory are independent of each other. The C standard library provides atomic types, such as std::atomic_int, and member functions such as load() and store() for performing atomic operations. These operations are either performed in full or not at all, preventing data corruption caused by concurrent access. Practical cases such as lock-free queues demonstrate the practical application of atomic operations. Use fetch_add() to atomically update the head and tail pointers of the queue to ensure the atomicity and consistency of queue operations.
Atomic operations in C memory management
Atomic operations are performed within a single atomic operation Instruction sequence, between system schedules. This means that the operation will either be executed in full or not at all, and it will not be interrupted midway. This is crucial for managing memory in a multi-threaded environment, as we can ensure that accesses to shared memory are independent of each other.
C Atomic types in the standard library
C The standard library provides a collection of atomic types, including:
-
std ::atomic_int
: Atomic integer -
std::atomic_bool
: Atomic Boolean value -
std::atomic_size_t
: Atomicsize_t
Type
Atomic operations
In order to perform atomic operations on atomic variables, you can use the std::atomic
class provided Member functions of:
-
load()
: Load the current value of the atomic variable -
store()
: Store the value to the atom In a variable -
fetch_add()
: Atomicly add a value to an atomic variable -
compare_exchange_strong()
: Compare the current value and only match Time exchange
Practical case: lock-free queue
Let us create a lock-free queue to demonstrate the practical application of atomic operations:
#include <deque> #include <atomic> template<typename T> class ConcurrentQueue { private: std::deque<T> data; std::atomic<size_t> head; std::atomic<size_t> tail; public: ConcurrentQueue() { head.store(0); tail.store(0); } void push(T item) { data[tail.fetch_add(1)] = item; } T pop() { if (head == tail) { return T{}; } return data[head.fetch_add(1)]; } size_t size() { return tail - head; } };
This queue uses atomic operations to ensure that operations on the queue are atomic and consistent. The push()
method uses fetch_add()
to atomically add tail
and store the new element. The pop()
method uses fetch_add()
to atomically add head
and retrieve elements.
Conclusion
Atomic operations are very useful in multi-threaded programming, they can ensure that concurrent access to shared memory is consistent and predictable. The C standard library provides collections of atomic types and related operations, allowing us to easily implement lock-free data structures, thereby improving the performance and reliability of concurrent code.
The above is the detailed content of Atomic operations in C++ memory management. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
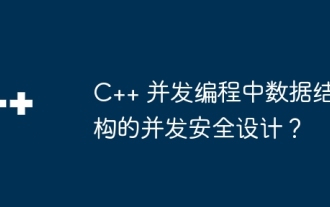
In C++ concurrent programming, the concurrency-safe design of data structures is crucial: Critical section: Use a mutex lock to create a code block that allows only one thread to execute at the same time. Read-write lock: allows multiple threads to read at the same time, but only one thread to write at the same time. Lock-free data structures: Use atomic operations to achieve concurrency safety without locks. Practical case: Thread-safe queue: Use critical sections to protect queue operations and achieve thread safety.

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
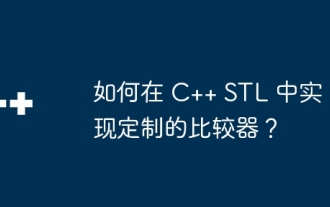
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
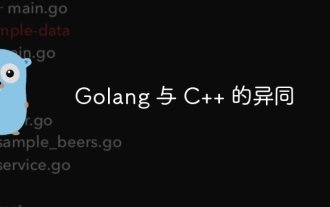
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
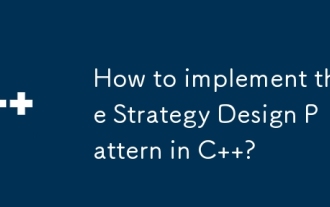
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
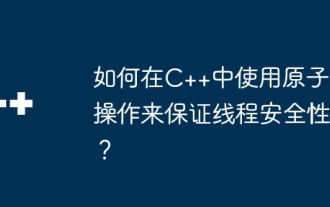
Thread safety can be guaranteed by using atomic operations in C++, using std::atomic template class and std::atomic_flag class to represent atomic types and Boolean types respectively. Atomic operations are performed through functions such as std::atomic_init(), std::atomic_load(), and std::atomic_store(). In the actual case, atomic operations are used to implement thread-safe counters to ensure thread safety when multiple threads access concurrently, and finally output the correct counter value.
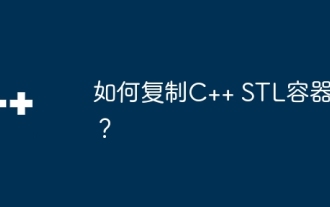
There are three ways to copy a C++ STL container: Use the copy constructor to copy the contents of the container to a new container. Use the assignment operator to copy the contents of the container to the target container. Use the std::copy algorithm to copy the elements in the container.
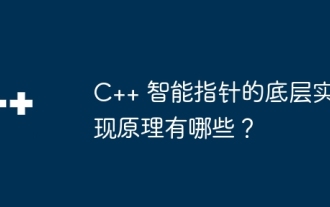
C++ smart pointers implement automatic memory management through pointer counting, destructors, and virtual function tables. The pointer count keeps track of the number of references, and when the number of references drops to 0, the destructor releases the original pointer. Virtual function tables enable polymorphism, allowing specific behaviors to be implemented for different types of smart pointers.
