


Detailed explanation of C++ function recursion: Recursion in dynamic programming
Abstract: Recursive calls are implemented in C by calling its own function. The recursive solution of the Fibonacci sequence requires three components: basic conditions (n is less than or equal to 1), recursive calls (solving F(n-1) and F(n-2) by itself), increment/decrement (n every recursion Decrease 1) at a time. The advantage is that the code is concise, but the disadvantage is that the space complexity is high and stack overflow may occur. For large data sets, it is recommended to use dynamic programming to optimize space complexity.
#C Detailed explanation of function recursion: Recursion in dynamic programming
Recursion is the process of a function calling itself. In C, a recursive function needs to have the following components:
- Basic conditions: when the recursion ends
- Recursive call: the function calls itself
- Increment/decrement: The calculation or modification used each time the function is called recursively
Practical case: Fibonacci sequence
The Fibonacci sequence is a sequence of numbers. Each number is the sum of the previous two numbers. It can be expressed as:
F(n) = F(n-1) F(n-2)
The following is a function that uses C to recursively solve the Fibonacci sequence:
int fibonacci(int n) { if (n <= 1) { return n; } return fibonacci(n-1) + fibonacci(n-2); }
How to understand recursive solution of Fibonacci sequence
- ##Basic conditions: When n is less than or equal to 1, the recursion ends and n is returned .
- Recursive call: Otherwise, the function calls itself to solve for F(n-1) and F(n-2).
- Increment/decrement: n decreases by 1 for each recursion.
Advantages and Disadvantages
Advantages:
- The code is concise and clear
- Easy to understand
Disadvantages:
- High space complexity (save each recursive call in the stack)
- may occur Stack overflow (when the recursion depth is too large)
Tips:
- For large data sets, it is recommended to use dynamic programming methods instead of recursion, to optimize space complexity.
- It is important to understand the recursion termination conditions to avoid infinite recursion.
The above is the detailed content of Detailed explanation of C++ function recursion: Recursion in dynamic programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


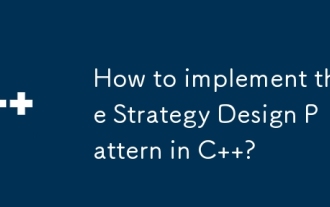
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
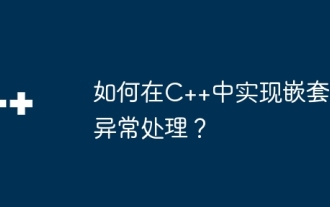
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
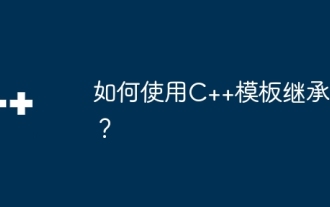
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
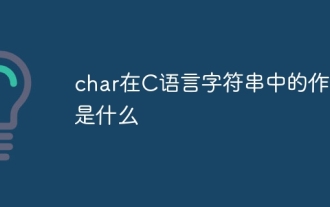
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
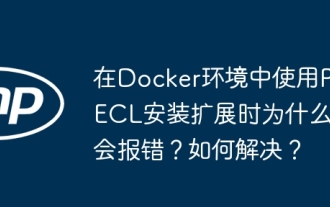
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
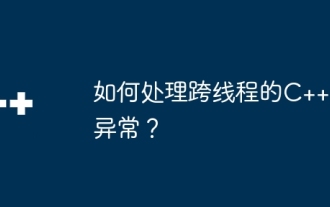
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
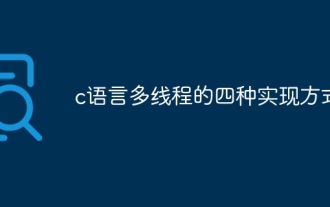
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
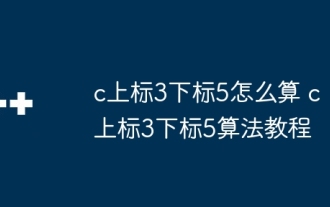
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
