Unit testing with Go generics
Using Go generics for unit testing can create universal test functions suitable for multiple types, improving the reusability, maintainability and readability of test code. Specific advantages include: Reusability: Generic test functions are applicable to multiple types, reducing duplication of code. Maintainability: Centrally manage generic test functions to keep the code clean. Readability: Generic syntax improves code readability and understandability.
Using Go Generics for Unit Testing
Go 1.18 introduces the generics feature, allowing developers to create more general and more Flexible code. The same applies to unit testing, which simplifies the reusability and maintainability of your test code.
Generic test functions
Using generics, we can create test functions that apply to a wide range of collections of types. For example, we can define a generic assertLess
function for any type that implements the comparable
interface:
func assertLess[T comparable](t *testing.T, got, want T) { if got >= want { t.Errorf("got %v, want less than %v", got, want) } }
Practical case
Here is an example using assertLess
to test the math/big.Int
type:
package big import ( "math/big" "testing" ) func TestIntLess(t *testing.T) { tests := []struct { got, want *big.Int }{ {got: big.NewInt(1), want: big.NewInt(2)}, {got: big.NewInt(5), want: big.NewInt(3)}, } for _, tt := range tests { assertLess(t, tt.got, tt.want) } }
Advantages
Using generics for unit testing has the following advantages:
- Reusability:Generic test functions can be applied to multiple types, reducing the risk of duplicating code.
- Maintainability: Centralized management of generic test functions makes it easier to keep the test code clean.
- Readability: Generic syntax improves the readability and understandability of test code.
Conclusion
Go generics provide powerful capabilities for unit testing, allowing us to write more versatile and reusable test code. By combining generics with clear, concise syntax, we can improve the quality and maintainability of our test code.
The above is the detailed content of Unit testing with Go generics. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
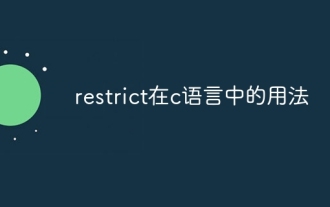
The restrict keyword is used to inform the compiler that a variable can only be accessed by a pointer, preventing undefined behavior, optimizing code and improving readability: Preventing undefined behavior when multiple pointers point to the same variable. To optimize code, the compiler uses the restrict keyword to optimize variable access. Improves code readability by indicating that variables can only be accessed by a pointer.
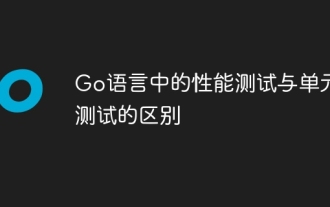
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.
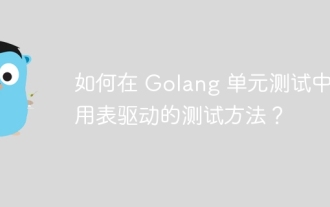
Table-driven testing simplifies test case writing in Go unit testing by defining inputs and expected outputs through tables. The syntax includes: 1. Define a slice containing the test case structure; 2. Loop through the slice and compare the results with the expected output. In the actual case, a table-driven test was performed on the function of converting string to uppercase, and gotest was used to run the test and the passing result was printed.
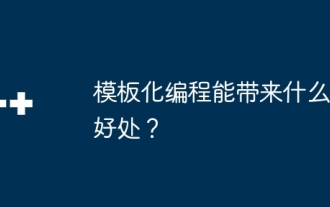
Templated programming improves code quality because it: Enhances readability: Encapsulates repetitive code, making it easier to understand. Improved maintainability: Just change the template to accommodate data type changes. Optimization efficiency: The compiler generates optimized code for specific data types. Promote code reuse: Create common algorithms and data structures that can be reused.
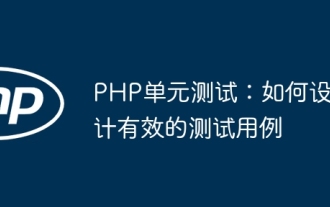
It is crucial to design effective unit test cases, adhering to the following principles: atomic, concise, repeatable and unambiguous. The steps include: determining the code to be tested, identifying test scenarios, creating assertions, and writing test methods. The practical case demonstrates the creation of test cases for the max() function, emphasizing the importance of specific test scenarios and assertions. By following these principles and steps, you can improve code quality and stability.
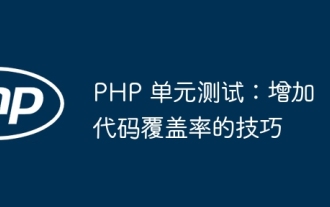
How to improve code coverage in PHP unit testing: Use PHPUnit's --coverage-html option to generate a coverage report. Use the setAccessible method to override private methods and properties. Use assertions to override Boolean conditions. Gain additional code coverage insights with code review tools.
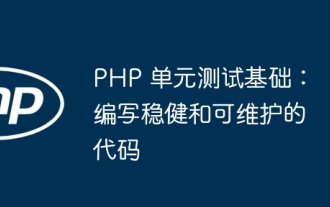
PHPUnit is a popular PHP unit testing framework that can be used to write robust and maintainable test cases. It contains the following steps: installing PHPUnit and creating the tests directory to store test files. Create a test class that inherits PHPUnit\Framework\TestCase. Define test methods starting with "test" to describe the functionality to be tested. Use assertions to verify that expected results are consistent with actual results. Run vendor/bin/phpunit to run tests from the project root directory.
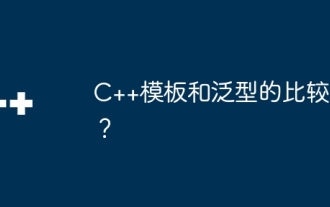
The difference between templates and generics in C++: Templates: defined at compile time, clearly typed, high efficiency, and small code size. Generics: runtime typing, abstract interface, provides flexibility, low efficiency.
