


Detailed explanation of C++ function recursion: recursive traversal of tree structures
Recursive functions can be used to traverse a tree structure. The basic principle is that the function continuously calls itself and passes in different parameter values until the basic situation terminates the recursion. In practical cases, the recursive function used to traverse a binary tree follows the following process: if the current node is empty, return; recursively traverse the left subtree; output the value of the current node; recursively traverse the right subtree. The complexity of the algorithm depends on the structure of the tree, for a complete binary tree the number of recursive calls is 2n. Note that you should ensure that the base case terminates the recursive process and use recursion with caution to avoid stack overflows.
Detailed explanation of C function recursion: recursive traversal of tree structure
Preface
Recursion is an important algorithm design technique in computer science that solves problems by constantly calling itself. In C, functional recursion can provide concise and elegant solutions, especially when dealing with tree structures.
Basic principles of recursion
Function recursion follows the following basic principles:
- The function calls itself, passing in different parameter values.
- In recursive calls, the problem is decomposed into smaller sub-problems.
- The recursive process terminates when the size of the subproblem is reduced to the base case.
Practical case: Recursive traversal of a tree structure
Consider a binary tree data structure in which each node contains a value and two pointers to child nodes. . We're going to write a recursive function that traverses the tree and prints the node's value.
struct Node { int value; Node* left; Node* right; }; void printTree(Node* root) { if (root == nullptr) { return; // 基本情况:空树 } printTree(root->left); // 递归左子树 cout << root->value << " "; // 输出根节点的值 printTree(root->right); // 递归右子树 }
Algorithm process
- If the current node is empty, return (basic case).
- Recursively traverse the left subtree.
- Output the value of the current node.
- Recursively traverse the right subtree.
Complexity Analysis
The complexity of the recursive function depends on the structure of the tree. For a complete binary tree with n nodes, the number of recursive calls is 2n. For an unbalanced tree, the recursion depth may be much greater than the height of the tree.
Notes
- Avoid infinite loops in recursion and ensure that the basic situation can terminate the recursive process.
- Large-scale recursive calls may cause stack overflow, so recursion needs to be used with caution.
- For very large tree structures, consider using non-recursive algorithms (such as depth-first search or breadth-first search).
The above is the detailed content of Detailed explanation of C++ function recursion: recursive traversal of tree structures. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


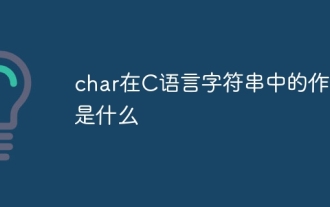
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
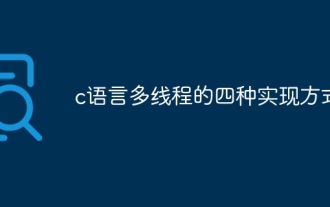
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
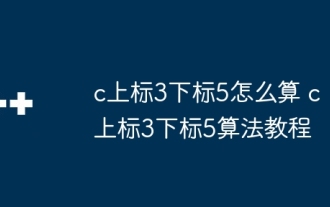
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
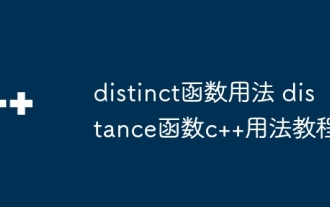
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
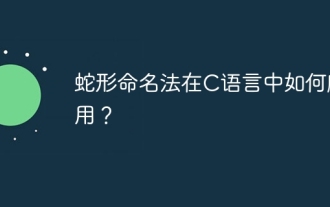
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
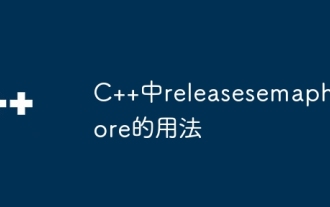
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
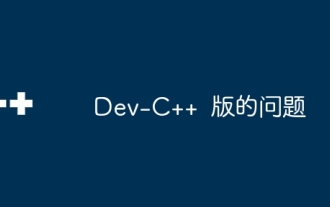
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
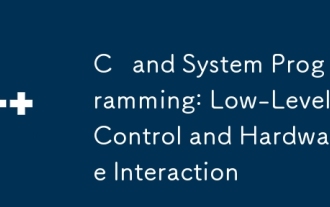
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
