


How to use barriers to achieve thread synchronization in Java concurrent programming?
A barrier is a synchronization tool used to make a group of threads wait for all threads to reach a specified point before continuing execution. Barriers can be created using java.util.concurrent.CyclicBarrier, and each thread joins the barrier and waits for other threads by calling the await() method. When all threads have reached the barrier, they continue execution. Barriers can be used to ensure that no subsequent operations are performed until all threads have completed their calculations.
Using barriers to achieve thread synchronization in Java concurrent programming
The barrier is a synchronization tool in concurrent programming that allows a The group thread waits for all threads to reach a certain point before continuing execution. This is useful in certain scenarios, such as performing subsequent operations after all threads have completed initialization.
Create Barrier
We can use the java.util.concurrent.CyclicBarrier
class to create a barrier. The constructor of this class receives an integer parameter representing the number of threads in the barrier.
CyclicBarrier barrier = new CyclicBarrier(4);
Using Barrier
To use a barrier, each thread must call the await()
method. This method will block the thread until all threads in the barrier have called the await()
method. When all threads have reached the barrier, they continue execution.
for (int i = 0; i < 4; i++) { new Thread(() -> { try { barrier.await(); } catch (InterruptedException | BrokenBarrierException e) { e.printStackTrace(); } }).start(); }
Practical Case
Suppose we have a group of threads that need to generate a report that can only be summarized when all threads have completed their calculations. We can use a barrier to ensure that aggregation does not start until all threads have completed their calculations.
cyclicBarrier.await(); // 汇总结果并生成报告
Notes
- Barriers are a synchronization tool that can slow down a program and should be considered carefully when used.
- The barrier does not support interruption, so when a thread is blocked in the barrier, other threads cannot interrupt it.
- The barrier supports timeout. If a thread does not reach the barrier within the specified time, the barrier will throw a
BrokenBarrierException
exception and all other threads will continue to execute.
The above is the detailed content of How to use barriers to achieve thread synchronization in Java concurrent programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
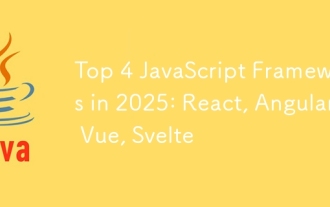
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
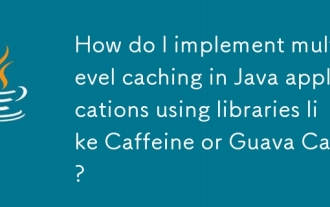
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
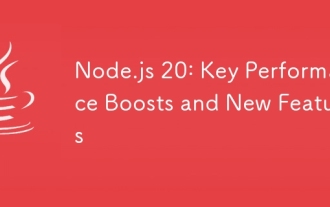
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
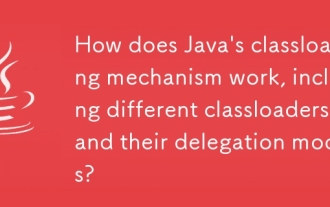
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
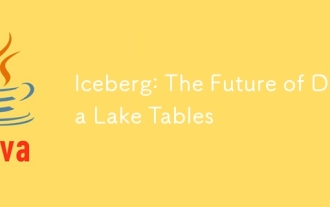
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
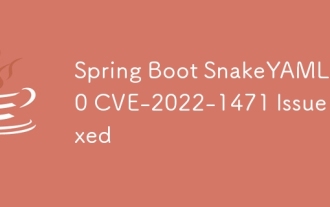
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
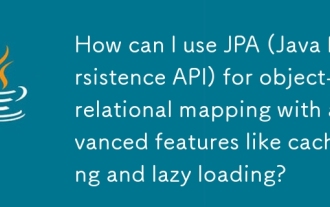
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
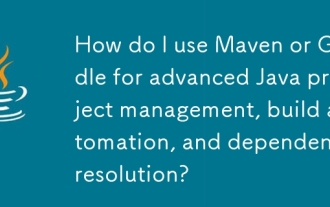
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
