


Detailed explanation of golang function caching mechanism and best practices
The function caching mechanism in the Go language implements the storage and reuse of function results through sync.Pool, thus improving program performance. This mechanism has a significant effect on pure functions and frequently called functions. Best practices include choosing an appropriate cache size, using small objects, shortening object lifetimes, and caching only pure functions and frequently called functions.
Detailed explanation and best practices of Go language function caching mechanism
The function caching mechanism is an important performance optimization technology that can store frequently called functions The result is improved application performance. The Go language provides a built-in mechanism to support function caching. This article will introduce this mechanism in detail and provide best practices.
Principle and Mechanism
The function cache in the Go language is implemented through the sync.Pool
type. sync.Pool
is a collection of allocated objects that can be reused in a thread-safe environment. When a function is called, the Go language attempts to obtain the function result from sync.Pool
. If the result is cached, it is returned directly; otherwise, the function is executed and the result is cached for future use.
Usage examples
import ( "sync" ) var pool = &sync.Pool{ New: func() interface{} { return 0 }, } func GetCounter() *int { v := pool.Get().(*int) v++ return v } func main() { for i := 0; i < 1000000; i++ { counter := GetCounter() fmt.Println(*counter) pool.Put(counter) } }
Best practices
- Cache only pure functions: The function caching mechanism is only effective for pure functions, that is, it is not modified A function of its state or an external state.
- Cache only frequently called functions: It only makes sense to cache frequently called functions. For functions that are rarely called, the cost of caching is greater than the benefits.
-
Choose appropriate cache capacity: The capacity of
sync.Pool
will affect performance. A capacity that is too small will result in frequent allocation of objects, while a capacity that is too large will waste memory. Ideally, the capacity should be large enough to accommodate commonly used function results, yet small enough to avoid unnecessary memory overhead. - Use smaller objects: The smaller the cached objects, the better, because this will reduce the overhead of allocating and releasing objects.
-
Use a short life cycle: When an object is no longer needed, it should be put back into
sync.Pool
as soon as possible to release its resources.
The above is the detailed content of Detailed explanation of golang function caching mechanism and best practices. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
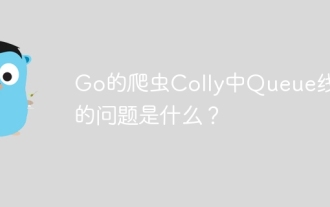
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
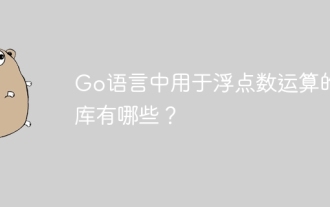
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
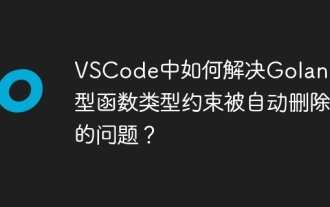
Automatic deletion of Golang generic function type constraints in VSCode Users may encounter a strange problem when writing Golang code using VSCode. when...
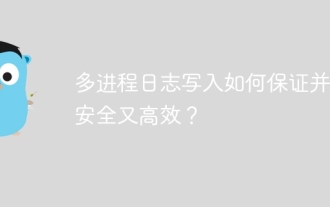
Efficiently handle concurrency security issues in multi-process log writing. Multiple processes write the same log file at the same time. How to ensure concurrency is safe and efficient? This is a...
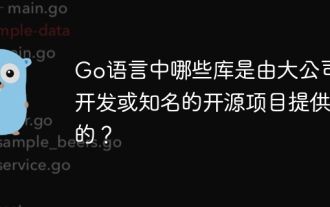
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
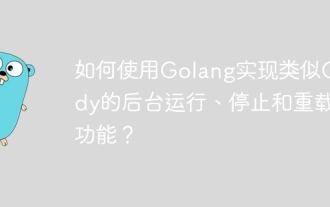
How to implement background running, stopping and reloading functions in Golang? During the programming process, we often need to implement background operation and stop...
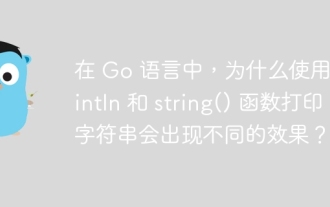
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
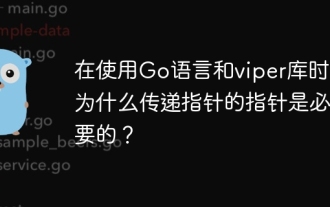
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
