


C++ function exceptions and multithreading: error handling in concurrent environments
Function exception handling in C is particularly important for multi-threaded environments to ensure thread safety and data integrity. The try-catch statement allows you to catch and handle specific types of exceptions when they occur to prevent program crashes or data corruption.
C Function exceptions and multi-threading: Error handling in concurrent environments
In a multi-threaded environment, it is crucial to handle function exceptions to ensure that the program stability and data integrity. This article will introduce the technology of function exception handling in C, and provide a practical case to illustrate how to handle exceptions in a concurrent environment.
Function exception handling basics
Function exception handling in C is mainly implemented through the try-catch
statement, whose syntax is as follows:
try { // 代码块 } catch (exception_type &e) { // 异常处理代码 }
A try
block contains code that may throw an exception, while a catch
block is used to catch and handle specific types of exceptions.
Exception handling in a concurrent environment
In a multi-threaded environment, exception handling becomes more complex because multiple threads may reference and modify shared data at the same time. Therefore, extra precautions need to be taken to ensure thread safety and data integrity.
Practical Case: Thread Pool
As a practical case, let us consider a thread pool that uses multiple threads to perform tasks. We can add exception handling to ensure that no data corruption occurs during task execution:
#include <thread> #include <vector> #include <future> using namespace std; // 任务函数 void task(int i) { // 可能会引发异常的代码 if (i < 0) { throw invalid_argument("负数参数"); } cout << "任务 " << i << " 已完成" << endl; } int main() { // 创建线程池 vector<thread> threads; vector<future<void>> futures; // 提交任务 for (int i = 0; i < 10; i++) { futures.push_back(async(task, i)); } // 获取任务结果 try { for (auto &future : futures) { future.get(); } } catch (exception &e) { cerr << "异常: " << e.what() << endl; } // 等待所有线程加入 for (auto &thread : threads) { thread.join(); } return 0; }
In this example, if the argument to the task
function is negative, it will throw an exception. We catch this exception in the main
function and print the error message in the console. This way, even if one task fails, the entire program does not crash and other tasks can continue to execute.
Conclusion
Handling function exceptions in a multi-threaded environment is critical to ensuring the robustness and stability of your application. By using the try-catch
statement and taking appropriate precautions, we can handle exceptions and prevent program crashes or data corruption.
The above is the detailed content of C++ function exceptions and multithreading: error handling in concurrent environments. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
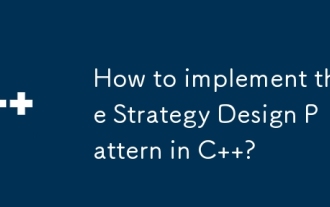
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
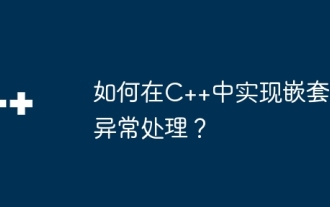
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
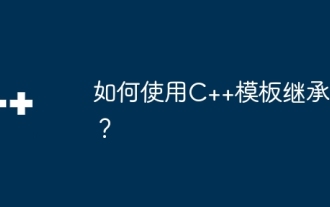
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
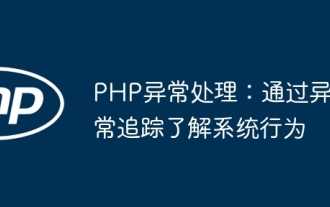
PHP exception handling: Understanding system behavior through exception tracking Exceptions are the mechanism used by PHP to handle errors, and exceptions are handled by exception handlers. The exception class Exception represents general exceptions, while the Throwable class represents all exceptions. Use the throw keyword to throw exceptions and use try...catch statements to define exception handlers. In practical cases, exception handling is used to capture and handle DivisionByZeroError that may be thrown by the calculate() function to ensure that the application can fail gracefully when an error occurs.
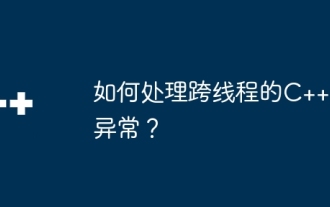
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
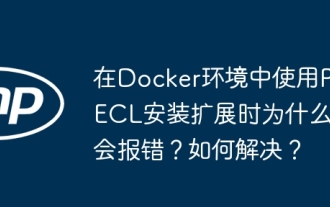
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
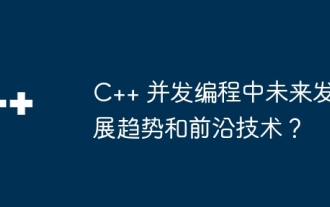
Future trends in C++ concurrent programming include distributed memory models, which allow memory to be shared on different machines; parallel algorithm libraries, which provide efficient parallel algorithms; and heterogeneous computing, which utilizes different types of processing units to improve performance. Specifically, C++20 introduces std::execution and std::experimental::distributed libraries to support distributed memory programming, C++23 is expected to include the std::parallel library to provide basic parallel algorithms, and C++AMP Libraries are available for heterogeneous computing. In actual combat, the parallelization case of matrix multiplication demonstrates the application of parallel programming.
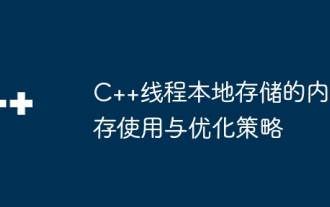
TLS provides each thread with a private copy of the data, stored in the thread stack space, and memory usage varies depending on the number of threads and the amount of data. Optimization strategies include dynamically allocating memory using thread-specific keys, using smart pointers to prevent leaks, and partitioning data to save space. For example, an application can dynamically allocate TLS storage to store error messages only for sessions with error messages.
