Strategies for resolving type conflicts in PHP functions
Strategies to resolve type conflicts in PHP functions include: 1. Explicit type conversion; 2. Type annotations; 3. Default parameter values; 4. Union types. In practice, type annotations can be used to enforce parameter types, combined with explicit type conversions to validate input.
Strategy for resolving type conflicts in PHP functions
In PHP, the parameter and return value types of functions are optional declarations of. However, when a type is declared, PHP will perform type checking and raise an error if a conflict occurs.
Type conflict
Type conflict refers to the situation where the parameter type or return value type of a function does not match the actual variable type passed in. For example:
function sum(int $a, int $b): int {} sum('1', 2); // TypeError: Argument 1 passed to sum() must be of the type integer, string given
Resolution strategy
There are several ways to resolve type conflicts in PHP functions:
1. Explicit types Conversion
Explicit type conversion uses the settype()
function to force a variable to the desired type. However, this may produce unexpected or incorrect results. For example:
function divide(int $a, int $b): int {} $a = '10'; settype($a, 'integer'); divide($a, 2); // Result: 5 (should be float)
2. Type annotations
PHP 7 introduced type annotations, allowing you to declare parameter and return value types in function declarations. Type annotations are safer than explicit type conversions because they catch type conflicts at compile time.
function divide(int $a, int $b): float {} $a = '10'; divide($a, 2); // TypeError: Argument 1 passed to divide() must be of the type integer, string given
3. Default parameter values
Providing default values for function parameters can avoid type conflicts, because the default value will have the declared type. For example:
function divide(int $a = 0, int $b = 1): float {} $a = '10'; divide($a); // Result: 5.0 (float)
4. Union type
The Union type allows you to specify multiple acceptable parameter types. This is useful for working with data from different sources or formats. For example:
function process(int|string $value): void {} process(10); // int process('10'); // string
Practical case
The following is a practical case that demonstrates how to use type annotations and type conversions to resolve type conflicts in PHP functions:
function calculateArea(float $width, float $height): float { if (!is_numeric($width) || !is_numeric($height)) { throw new TypeError('Both width and height must be numeric'); } return $width * $height; } $width = '10'; $height = 5; try { $area = calculateArea($width, $height); echo "Area: $area"; } catch (TypeError $e) { echo $e->getMessage(); }
This script uses type annotations to enforce that the width
and height
parameters are floating point numbers. It also uses explicit type conversion to validate the input and throws an error if the input is not a number.
The above is the detailed content of Strategies for resolving type conflicts in PHP functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


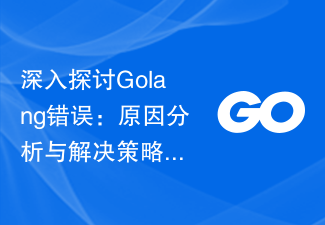
As a fast and efficient programming language, Golang language is favored by more and more developers. However, in daily development, we will inevitably encounter various errors. How to correctly analyze the causes of errors and find solution strategies is an important skill that every Golang developer needs to understand and master in depth. Error analysis type assertion failure In Golang, type assertion is a common operation, but sometimes if the types do not match, panic will occur. Here is a simple example: varxinter
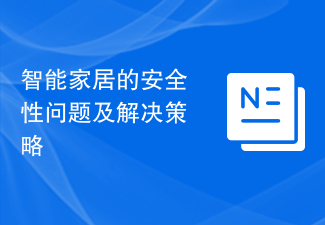
Nowadays, smart home applications are becoming more and more widespread. They not only bring convenience, but also bring some security challenges. The networked nature of smart home devices makes them susceptible to hackers, leading to problems such as leaking personal information and exposing family privacy. Therefore, we need to find some solutions to ensure that our smart home devices are secure. First, we need to choose reliable equipment manufacturers and suppliers. When purchasing smart home equipment, we should carefully consider which brands or manufacturers to choose to provide the equipment we need. we want

How to solve golang error: cannotrefertounexportedname'x'inpackage'y' During the development process using golang, we may encounter such an error: "cannotrefertounexportedname'x'inpackage'y'". This error is mainly because we are accessing unexported variable or function. in golang
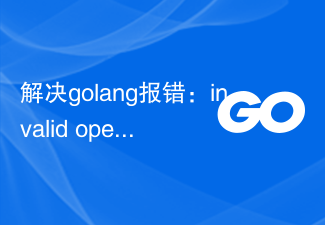
Solve golang error: invalidoperation: non-numerictype'x', solution strategy When programming with Golang, we sometimes encounter error messages similar to "invalidoperation: non-numerictype'x'". This error usually occurs when performing numerical operations on non-numeric types, such as adding strings to numbers or performing comparison operations. This article will introduce this question
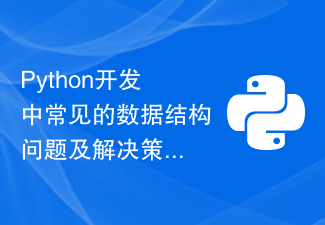
Common data structure problems and solution strategies in Python development In Python development, using effective data structures is crucial. Good data structures can improve the efficiency and performance of algorithms. However, sometimes you encounter some common problems when dealing with data structures. This article will introduce some common data structure problems, as well as solutions to these problems, and provide specific code examples. Linked list reversed linked list is a common linear data structure that can be used to store any type of data. When dealing with linked lists, it is often necessary to
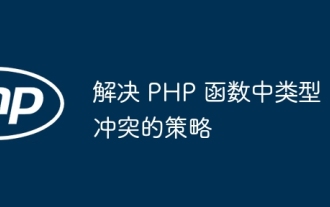
Strategies to resolve type conflicts in PHP functions include: 1. Explicit type conversion; 2. Type annotations; 3. Default parameter values; 4. Union types. In practice, type annotations can be used to enforce parameter types, combined with explicit type conversions to validate input.
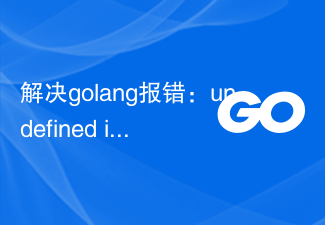
Solve golang error: undefinedinterfacemethod'x'oftypeT, solution strategy During the development process of using Golang, we often encounter some error messages. One of the common errors is "undefinedinterfacemethod'x'oftypeT". This error message indicates that we defined an interface type T and implemented the interface on a certain structure or type, but
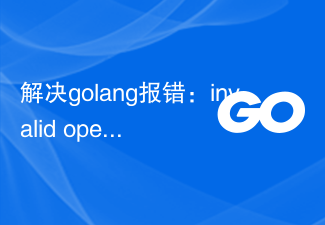
Solve golang error: invalidoperation: operator'x'notdefinedfor'y'(typeT), solution strategy When using Golang programming, you sometimes encounter such error message: "invalidoperation: operator'x'notdefinedfor'y'(typeT)" . This error message means that when using an operation
