What are the commonly used JSON parsing tools in Java function libraries?
The most commonly used JSON parsing library in Java: Jackson: fast and feature-rich, supporting annotations, data binding and multiple formats. Gson: easy to use and fast, providing convenient API, strong type support and automatic deserialization. Other popular libraries: JSON-B: JAXB-like library for converting Java objects to JSON and vice versa. Smile: A very fast binary JSON format parsing library. JsonPath: Library for querying and transforming JSON data. Factors such as performance, functionality, ease of use, etc. should be considered when selecting a library.
Common JSON parsing libraries in Java
JSON (JavaScript Object Notation) is a popular data exchange format used for transmission in web applications and store data. In Java, there are many popular JSON parsing libraries available. This article will introduce some of the most commonly used libraries and their characteristics.
Jackson
Jackson is probably the most widely used JSON parsing library in Java. It is fast and feature-rich, providing the following features:
- Annotation support: Serialization and deserialization behavior can be configured using annotations.
- Data Binding: Can bind JSON data to Java objects and vice versa.
- Support of various formats: Supports JSON, YAML, XML, CSV and other formats.
Practical case:
import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.core.JsonProcessingException; public class JacksonExample { public static void main(String[] args) throws JsonProcessingException { // 创建一个 ObjectMapper 实例 ObjectMapper mapper = new ObjectMapper(); // 将 Java 对象序列化为 JSON String json = mapper.writeValueAsString(new Person("John", "Doe")); // 将 JSON 反序列化为 Java 对象 Person person = mapper.readValue(json, Person.class); // 打印反序列化后的对象 System.out.println(person); } } class Person { private String firstName; private String lastName; // ... 省略 getter 和 setter 方法 }
Gson
Gson is another popular JSON parsing library known for its speed and ease of use. famous. It provides the following features:
- Convenient API: Use a simple API to parse and generate JSON data.
- Strong type support: JSON data can be mapped to strongly typed objects.
- Automated deserialization: Can automatically generate Java objects based on class names and data structures.
Practical case:
import com.google.gson.Gson; import com.google.gson.GsonBuilder; public class GsonExample { public static void main(String[] args) { // 创建一个 GsonBuilder 实例 GsonBuilder gsonBuilder = new GsonBuilder(); // 启用自动反序列化 gsonBuilder.enableComplexMapKeySerialization(); // 创建一个 Gson 实例 Gson gson = gsonBuilder.create(); // 将 Java 对象序列化为 JSON String json = gson.toJson(new Person("John", "Doe")); // 将 JSON 反序列化为 Java 对象 Person person = gson.fromJson(json, Person.class); // 打印反序列化后的对象 System.out.println(person); } }
Other libraries
In addition to Jackson and Gson, there are several other popular Java JSON parsing Libraries, including:
- JSON-B (JAXB Binding): A library similar to the JAXB specification for converting Java objects to JSON and vice versa.
- Smile: A binary JSON format parsing library that is very fast.
- JsonPath: A library for querying and transforming JSON data.
Factors to consider when choosing a JSON parsing library:
- Performance: Choose a speed specific to your application Fast library.
- Features: Consider the features you need, such as data binding, annotation support, or custom parsing.
- Ease of use: Choose a library with good documentation and a supporting community.
The above is the detailed content of What are the commonly used JSON parsing tools in Java function libraries?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


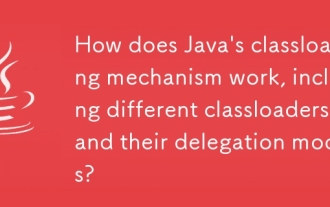
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
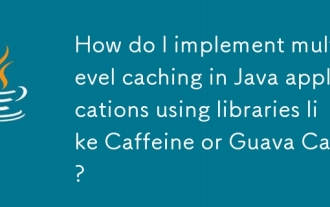
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
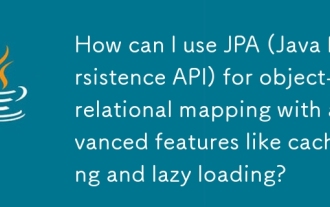
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
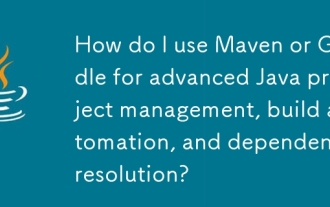
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
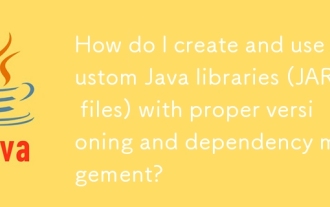
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
