


Open source projects and resource sharing of golang anonymous functions and closures
Anonymous functions and closures: Anonymous functions are nameless functions that are created on the fly to perform a specific task. Closures are variables that allow access to external variables within an anonymous function. In Go, they are declared using func() syntax. Anonymous functions and closures can be used to pass arguments, store in variables, or in practice for ordering slices and event handling.
Anonymous functions and closures in Go
Introduction
Anonymous functions are functions in Go that are not explicitly named and can be used as expressions, they are used to create one-time tasks or callbacks. A closure is an anonymous function that contains a reference to an external variable that persists even after the function returns.
Anonymous functions
Use the func()
syntax to declare anonymous functions:
func() { fmt.Println("这是一个匿名函数") }
Anonymous functions can be passed as parameters to other Functions can also be stored in variables:
func callAnon(anon func()) { anon() } var anonFunc = func() { fmt.Println("这是一个存储在变量中的匿名函数") }
Closures
Closures allow anonymous functions to access variables in the outer scope. These variables are called closure variables.
var x = 10 anon := func() { fmt.Println(x) // 访问闭包变量 } anon() // 输出:10
Practical case
- Sort slices: Use closure to sort slices by a certain field value:
type Employee struct { Name string Age int } func SortEmployeesByAge(employees []Employee) { sort.Slice(employees, func(i, j int) bool { return employees[i].Age < employees[j].Age }) }
- Event handler: Create the receiver function and specify the anonymous function as the callback:
type Button struct { onClick func() } func (b *Button) AddClickListener(f func()) { b.onClick = f }
Open source projects and resources
- [Go wiki: Anonymous functions and Closures](https://go.dev/blog/closures)
- [Practical Go Tutorial: Closures](https://go. dev/blog/closures)
- [Golang anonymous functions and closures](https://www.jianshu.com/p/8e78029d888a)
The above is the detailed content of Open source projects and resource sharing of golang anonymous functions and closures. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
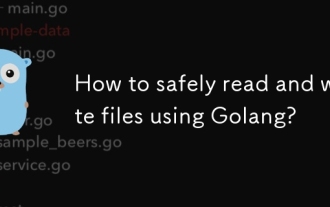
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
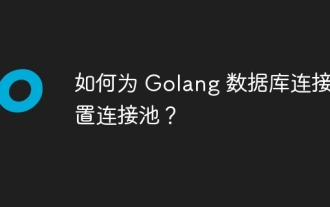
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
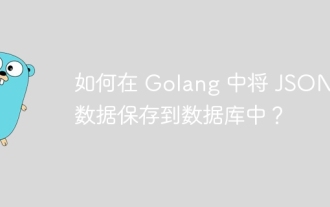
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
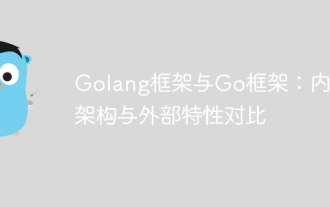
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
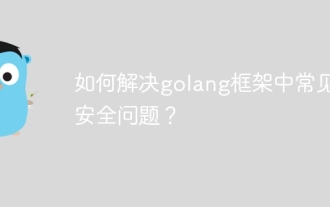
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
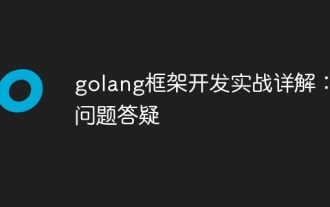
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
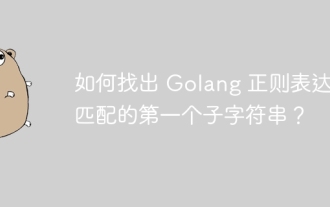
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
