Golang reflection limitations and alternatives
Question: What are the limitations of Go language reflection? Answer: High performance overhead makes it difficult to use non-inlineable alternatives: code generation (performance optimization) code introspection (replacement of reflection operation)
Limitations of Go language reflection Properties and Alternatives
Reflection is a powerful tool in the Go language, which allows you to introspect and modify program code at runtime. However, reflection also has some limitations, the most common limitations are listed below:
- High performance overhead: Reflection operations will involve a large number of runtime checks and memory allocation, There may be a significant impact on performance.
- Difficulty to use: The reflection API is complex and difficult to use, especially when designing type-safe code.
- Not inlineable: Reflection calls generally cannot be inlined, which may affect the size and performance of the final executable code.
Alternatives
Code generation
Code generation is the dynamic generation of source code as needed while the program is running a technology. This allows you to shift the overhead of reflection operations to compile time, thus improving performance. Code generation in Go can be achieved by using the go generate
build tag.
Code Introspection
Code introspection is a technique for obtaining program state and metadata through code rather than reflection. This can be achieved by using built-in functions such as reflect.TypeOf()
and reflect.ValueOf()
:
func TypeOfField(t reflect.Type, fieldname string) reflect.StructField { for i := 0; i < t.NumField(); i++ { field := t.Field(i) if field.Name == fieldname { return field } } panic("field not found") }
Practical example:
Here is a practical example that demonstrates the limitations of reflection and uses code introspection as an alternative:
package main import ( "fmt" "reflect" ) // 结构体 type Person struct { Name string Age int } func main() { // 创建结构体实例 person := Person{Name: "John", Age: 30} // 使用反射获取字段信息 t := reflect.TypeOf(person) // 获取结构体类型 field, ok := t.FieldByName("Name") // 根据字段名获取字段信息 if !ok { panic("field not found") } // 使用内省获取字段值 nameField := t.Field(0) // 根据字段索引获取字段信息 name := reflect.ValueOf(person).Field(0).Interface().(string) // 输出结果 fmt.Printf("Reflection: Field name: %s, Field value: %s\n", field.Name, name) }
Output using code introspection:
Reflection: Field name: Name, Field value: John
The above is the detailed content of Golang reflection limitations and alternatives. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
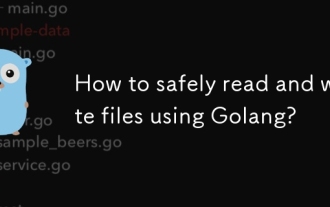
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
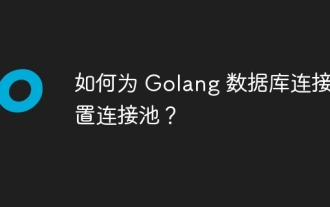
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
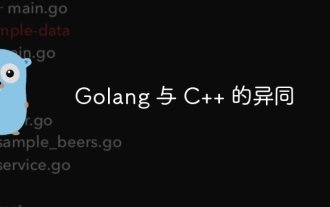
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
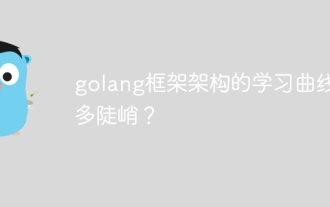
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
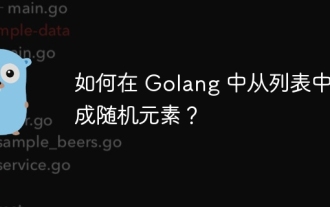
How to generate random elements of a list in Golang: use rand.Intn(len(list)) to generate a random integer within the length range of the list; use the integer as an index to get the corresponding element from the list.
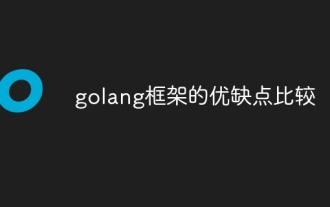
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
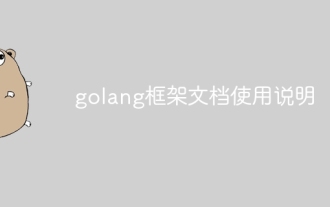
How to use Go framework documentation? Determine the document type: official website, GitHub repository, third-party resource. Understand the documentation structure: getting started, in-depth tutorials, reference manuals. Locate the information as needed: Use the organizational structure or the search function. Understand terms and concepts: Read carefully and understand new terms and concepts. Practical case: Use Beego to create a simple web server. Other Go framework documentation: Gin, Echo, Buffalo, Fiber.
