How to use file input array in python
Using Python files to input arrays can use the numpy.loadtxt() function. The specific steps are as follows: Import the NumPy library and open the file. Use the loadtxt() function to read the file, specifying the data delimiter. For example, suppose there are comma-separated data 1,2,3,4,5,6,7,8,9 in my_data.txt, which can be read using the following code: import numpy as np with open('my_data.txt', 'r') as f: data = np.loadtxt(f, delimiter=',')
##How to use Python file input array
In Python, you can use thenumpy.loadtxt() function to read data from a file and convert it into an array.
Steps:
- Import NumPy library:
import numpy as np
- Open File:
with open('my_data.txt', 'r') as f: # 这里输入文件路径和读取模式('r')
- Use the loadtxt()
function to read the file:
data = np.loadtxt(f, delimiter=',')
- delimiter
The parameter specifies the delimiter of the data (default is space).
Example:
Suppose you have a text file namedmy_data.txt that contains the following data (separated by commas ):
<code>1,2,3 4,5,6 7,8,9</code>
import numpy as np with open('my_data.txt', 'r') as f: data = np.loadtxt(f, delimiter=',') print(data)
<code>[[1. 2. 3.] [4. 5. 6.] [7. 8. 9.]]</code>
Note:
- If the data contains a header row, you can skip it using the
- skiprows
parameter.
You can also use the - dtype
parameter to specify the type of data (default is float).
If you want to read the data in binary format, you can use the - np.load()
function.
The above is the detailed content of How to use file input array in python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


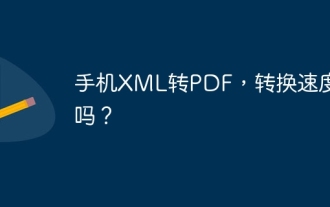
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
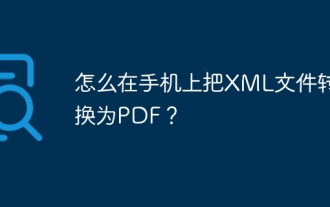
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
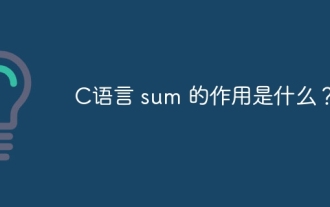
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
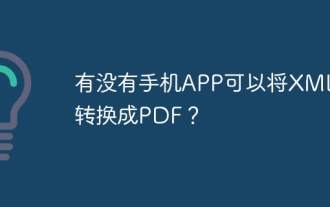
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
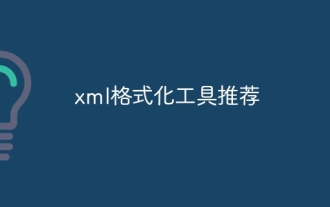
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
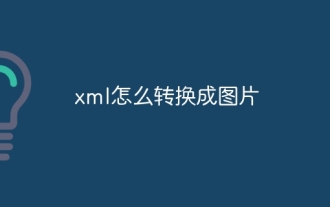
XML can be converted to images by using an XSLT converter or image library. XSLT Converter: Use an XSLT processor and stylesheet to convert XML to images. Image Library: Use libraries such as PIL or ImageMagick to create images from XML data, such as drawing shapes and text.
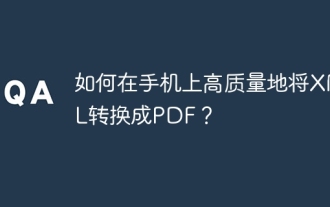
Convert XML to PDF with high quality on your mobile phone requires: parsing XML in the cloud and generating PDFs using a serverless computing platform. Choose efficient XML parser and PDF generation library. Handle errors correctly. Make full use of cloud computing power to avoid heavy tasks on your phone. Adjust complexity according to requirements, including processing complex XML structures, generating multi-page PDFs, and adding images. Print log information to help debug. Optimize performance, select efficient parsers and PDF libraries, and may use asynchronous programming or preprocessing XML data. Ensure good code quality and maintainability.
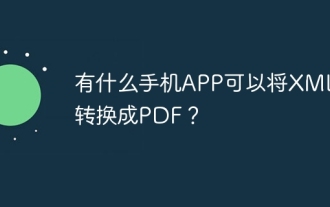
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
