How to get the current date data in js
There are two ways to get the current date data in JavaScript: use the Date object, for example: const now = new Date(); use the Date.now() method, for example: const timestamp = Date.now() ;
#How to get current date data in JavaScript
Getting current date data is a common task in JavaScript. Several methods can be used to achieve this:
Date
Objects
You can use Date
objects to represent specific dates and times. To get the current date, you can use the new Date()
constructor.
const now = new Date();
Date
The object provides a variety of methods to access date and time information, for example:
getDay()
: Get the day of the week Number (0-6, 0 represents Sunday)getDate()
: Get the date (1-31)getMonth()
: Get the month (0-11, where 0 represents January)getFullYear()
: Get the year
For example:
console.log(`当前星期:${now.getDay() + 1}`); // 输出:当前星期几 console.log(`当前日期:${now.getDate()}`); // 输出:当前日期 console.log(`当前月份:${now.getMonth() + 1}`); // 输出:当前月份 console.log(`当前年份:${now.getFullYear()}`); // 输出:当前年份
Date.now()
Another way to get the current date data is to use the Date.now()
method. This method returns the number of milliseconds since January 1, 1970 00:00:00 UTC.
const timestamp = Date.now();
This timestamp can be used to calculate relative times or compare with other timestamps.
Library
You can also use JavaScript libraries to obtain date data, for example:
- Moment.js: A tool for manipulating dates Popular library that provides a variety of convenient methods and formatting options.
- Date-fns: Another popular date library that provides some useful functions such as adding, subtracting and comparing dates.
The above is the detailed content of How to get the current date data in js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


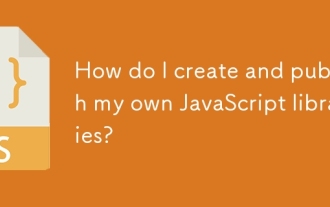
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
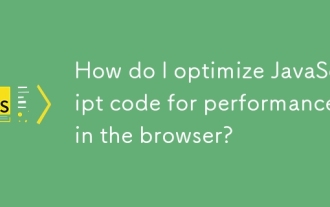
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
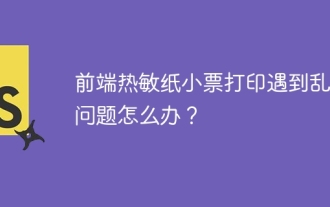
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
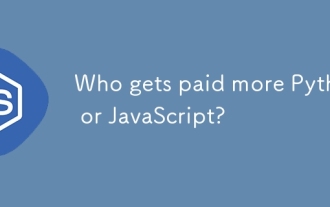
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
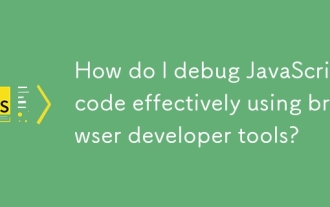
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
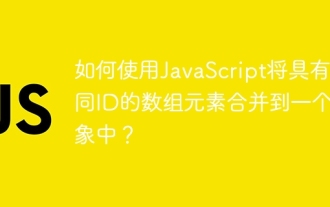
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
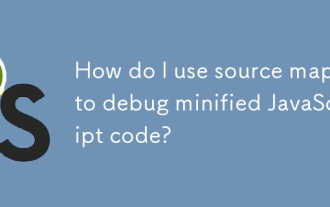
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
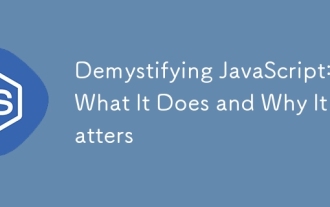
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
