Common problems and solutions in PHP unit testing practice
FAQ in PHP unit testing: External dependency testing: Use a mocking framework (such as Mockery) to create fake dependencies and assert their interactions. Private member testing: Use reflection APIs such as ReflectionMethod to access private members or use test visibility modifiers such as @protected. Database interaction testing: Set up and verify database state using a database testing framework such as DbUnit. External API/Web Service Testing: Use an HTTP client library to simulate interactions, using a local or stub server in the test environment.
Frequently Asked Questions in PHP Unit Testing
Question 1: How to target code with external dependencies unit test?
Solution: Use a mocking framework, such as PHPUnit's Mockery or Prophecy, that allows you to create fake dependency objects and make assertions about their interactions.
use Prophecy\Prophet; class UserRepoTest extends \PHPUnit\Framework\TestCase { public function testFetchUser(): void { $prophet = new Prophet(); $cache = $prophet->prophesize(Cache::class); $userRepo = new UserRepo($cache->reveal()); $actualUser = $userRepo->fetchUser(1); $cache->get(1)->shouldHaveBeenCalled(); $this->assertEquals($expectedUser, $actualUser); } }
Question 2: How to test private methods or properties?
Solution: Use reflection APIs (such as ReflectionClass
and ReflectionMethod
) that allow you to access private members. However, it can make tests difficult to maintain.
Another solution is to use a test-specific visibility modifier, such as PHPUnit's @protected
.
class UserTest extends \PHPUnit\Framework\TestCase { public function testPasswordIsSet(): void { $user = new User(); $reflector = new ReflectionClass($user); $property = $reflector->getProperty('password'); $property->setAccessible(true); $property->setValue($user, 'secret'); $this->assertEquals('secret', $user->getPassword()); } }
Question 3: How to test database interaction?
Solution: Use a database testing framework, such as PHPUnit's DbUnit or Doctrine DBAL Assertions, that allow you to set and verify database state.
use PHPUnit\DbUnit\TestCase; class PostRepoTest extends TestCase { protected function getConnection(): Connection { return $this->createDefaultDBConnection(); } public function testCreatePost(): void { $dataset = $this->createXMLDataSet(__DIR__ . '/initial-dataset.xml'); $this->getDatabaseTester()->setDataSet($dataset); $this->getDatabaseTester()->onSetUp(); $post = new Post(['title' => 'My First Post']); $postRepo->persist($post); $postRepo->flush(); $this->assertTrue($this->getConnection()->getRowCount('posts') === 1); } }
Question 4: How to test code that relies on external APIs or web services?
Solution: Use an HTTP client library to simulate interactions with external services. In a test environment, you can use a local or stub server.
use GuzzleHttp\Client; class UserServiceTest extends \PHPUnit\Framework\TestCase { public function testFetchUser(): void { $httpClient = new Client(); $userService = new UserService($httpClient); $httpClient ->shouldReceive('get') ->with('/users/1') ->andReturn(new Response(200, [], json_encode(['id' => 1, 'name' => 'John Doe']))); $user = $userService->fetchUser(1); $this->assertInstanceOf(User::class, $user); $this->assertEquals(1, $user->getId()); } }
The above is the detailed content of Common problems and solutions in PHP unit testing practice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
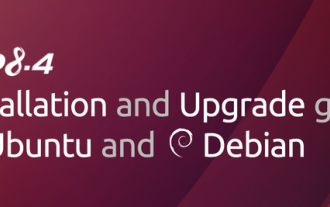
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
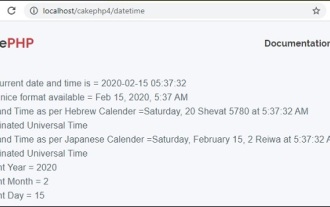
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
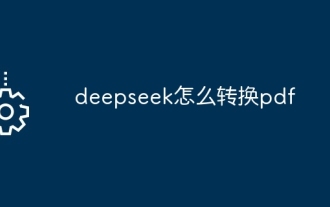
DeepSeek cannot convert files directly to PDF. Depending on the file type, you can use different methods: Common documents (Word, Excel, PowerPoint): Use Microsoft Office, LibreOffice and other software to export as PDF. Image: Save as PDF using image viewer or image processing software. Web pages: Use the browser's "Print into PDF" function or the dedicated web page to PDF tool. Uncommon formats: Find the right converter and convert it to PDF. It is crucial to choose the right tools and develop a plan based on the actual situation.
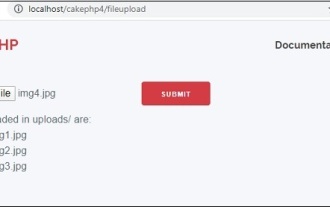
To work on file upload we are going to use the form helper. Here, is an example for file upload.
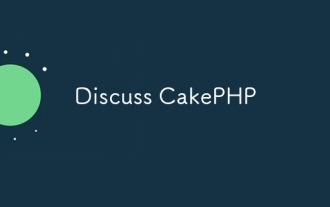
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
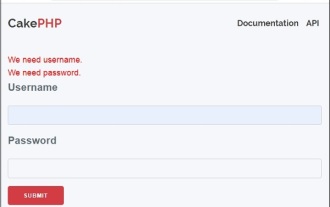
Validator can be created by adding the following two lines in the controller.
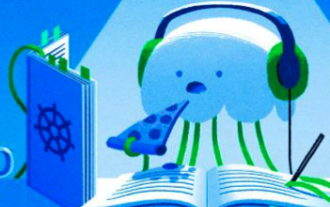
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
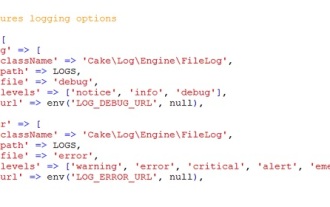
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
