The role of prototype in js
prototype is a property in JavaScript that points to a prototype object for shared properties and methods. Its main functions include: Inheritance: child objects inherit the prototype properties and methods of the parent object. Dynamically add properties: Dynamically add properties and methods by modifying the prototype object. Memory optimization: Multiple sub-objects share a prototype object to save memory. Code encapsulation: Encapsulate shared code into prototype objects to improve code clarity and maintainability.
The role of prototype in JavaScript
What is prototype?
Prototype is a special attribute in JavaScript that points to an object that contains properties and methods shared by other objects.
The functions of prototype
The main functions of prototype include:
- Inheritance:Child objects can inherit parent objects Properties and methods in the prototype to achieve code reuse.
- Dynamicly add properties: You can dynamically add properties and methods to sub-objects by modifying the prototype object.
- Memory optimization: Multiple sub-objects share a prototype object, which can save memory space.
- Code encapsulation: Encapsulate shared code into prototype objects to make the code clearer and easier to maintain.
Usage of prototype
You can use the following methods to access and modify the prototype object:
-
Get the prototype:
Object.getPrototypeOf(object)
-
Set prototype:
Object.setPrototypeOf(object, prototype)
Example description
The following code example demonstrates the use of prototype:
// 父对象 const Parent = { name: "Parent" }; // 子对象 const Child = { __proto__: Parent, // 设置子对象的原型指向父对象 age: 20 }; console.log(Child.name); // 输出: "Parent" console.log(Child.age); // 输出: 20 // 修改原型对象 Parent.hobby = "reading"; console.log(Child.hobby); // 输出: "reading"
In this example, the Child
object passes __proto__
Properties inherit the prototype of the Parent
object and share its name
property. At the same time, the Child
object can also dynamically access and modify the properties of the parent object, such as hobby
.
The above is the detailed content of The role of prototype in js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


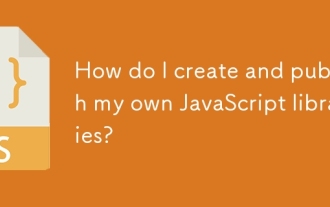
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
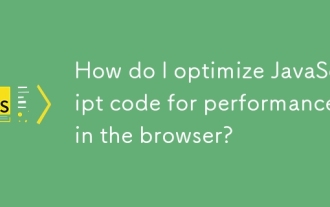
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
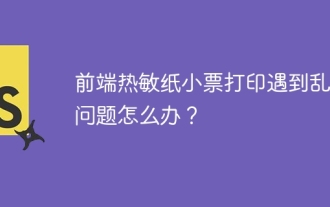
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
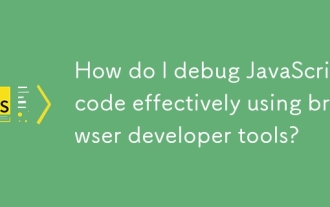
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
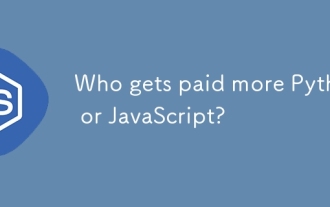
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
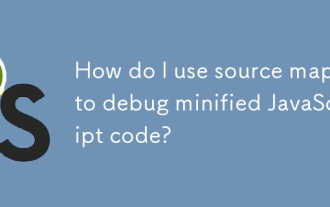
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
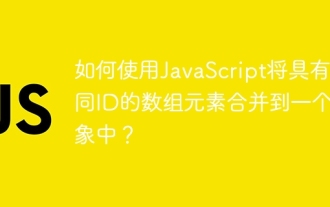
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
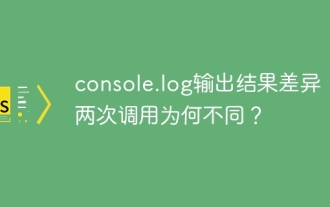
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
