


C++ Concurrent Programming: How to avoid thread starvation and priority inversion?
To avoid thread starvation, you can use fair locks to ensure fair allocation of resources, or set thread priorities. To solve priority inversion, you can use priority inheritance, which temporarily increases the priority of the thread holding the resource; or use lock promotion, which increases the priority of the thread that needs the resource.
C Concurrent programming: Avoid thread starvation and priority inversion
In concurrent programming, thread starvation and priority inversion are common challenges that can lead to deadlocks and uncertainty. This article explores these issues and provides solutions, illustrated with practical examples.
Thread starvation
Thread starvation occurs when a thread cannot obtain the required resources (such as locks and memory) for a long time. This may be caused by other threads accessing the resource first.
Solution strategy:
- Use fair lock: Fair lock ensures that all threads obtain resources fairly and prevents a single thread from holding resources for a long time .
- Set thread priority: Allocate more execution opportunities to high-priority threads to ensure that they are not blocked by low-priority threads.
Priority Inversion
Priority inversion occurs when a low-priority thread holds resources required by a high-priority thread. This may cause high-priority threads to be unable to execute, thereby delaying task completion.
Solution strategy:
- Use priority inheritance: When a thread holds a resource, its priority will be temporarily increased , to prevent low-priority threads from grabbing resources.
- Use lock promotion: When a thread needs to access a resource held by a high-priority thread, it will temporarily increase its priority to quickly obtain the resource.
Practical case
Consider the following scenario:
// Thread 1 (low priority) void thread1() { std::mutex m; m.lock(); // Critical section m.unlock(); } // Thread 2 (high priority) void thread2() { std::mutex m; m.lock(); // Critical section m.unlock(); }
Assume that thread2 runs with a higher priority than thread1. If thread1 acquires the lock first and enters the critical section, thread2 may be blocked. When thread1 releases the lock, thread2 may still be unable to acquire the lock because thread1 has a lower priority and will seize the lock again. This causes thread2 to starve.
To solve this problem, priority inheritance can be used:
void set_thread_priority(Thread thread, int priority); void thread1() { std::mutex m; m.lock(); // Critical section // Boost thread priority while holding lock set_thread_priority(std::this_thread::get_id(), 2); m.unlock(); }
Conclusion
By understanding thread starvation and priority inversion and applying appropriate Workaround strategies that can significantly improve the performance and reliability of concurrent code.
The above is the detailed content of C++ Concurrent Programming: How to avoid thread starvation and priority inversion?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
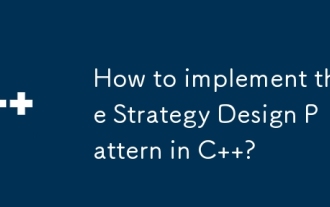
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
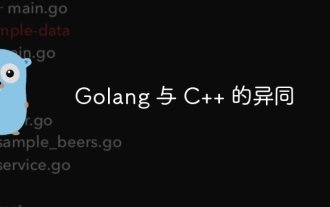
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
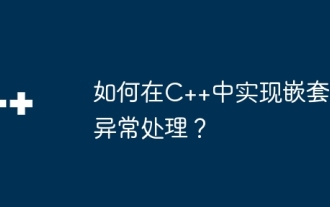
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
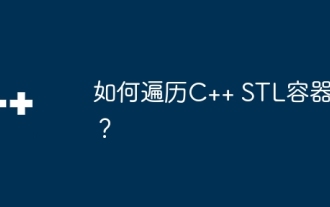
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
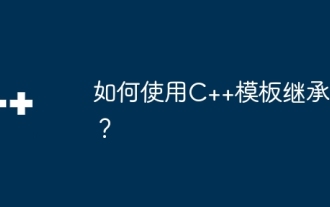
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
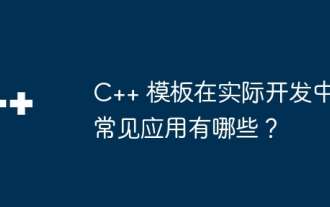
C++ templates are widely used in actual development, including container class templates, algorithm templates, generic function templates and metaprogramming templates. For example, a generic sorting algorithm can sort arrays of different types of data.
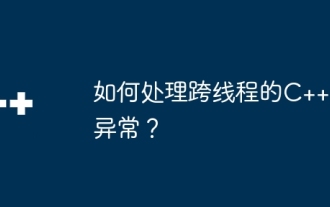
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
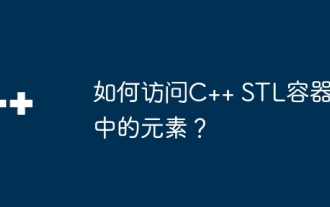
How to access elements in C++ STL container? There are several ways to do this: Traverse a container: Use an iterator Range-based for loop to access specific elements: Use an index (subscript operator []) Use a key (std::map or std::unordered_map)
