PHP Web service development and API design error handling
Error handling in PHP web services development involves the use of try-catch blocks, error constants, and custom error handlers. In API design, best practices include using HTTP status codes, defining JSON error responses, providing friendly error messages, and using custom error handling middleware to handle errors consistently, for example: Using custom error handling middleware to catch exceptions and returns a JSON response including error code and message.
Error handling and API design in PHP Web service development
Introduction
In PHP web service development, error handling is crucial to ensure the stability and maintainability of the application. API design also involves handling and returning errors to improve the client experience. This article will explore error handling techniques in PHP and how to apply them to API design.
Error handling techniques in PHP
PHP provides the following error handling mechanisms:
- try-catch block: Explicitly catch and handle exceptions.
- Error constants: Access predefined error constants to check error types.
- set_error_handler(): Customize the error handler to handle all errors.
Error handling practical case
Consider the following PHP code:
try { // 执行代码可能会抛出异常 } catch (Exception $e) { // 在这里处理异常 echo $e->getMessage(); }
This code block uses a try-catch block to handle any errors that may occur during Exceptions thrown when executing code. If an exception occurs, it catches the exception information and prints it.
Best Practices in API Error Handling
- Use HTTP status codes: Return appropriate HTTP status for different error types code, such as 400 (BadRequest) or 500 (InternalServerError).
- Define JSON error response: Create a JSON response object, including the error code, message, and any relevant details.
- Provide friendly error messages: Error messages should not only be accurate, but also understandable and user-friendly.
- Use custom error handling middleware: For large applications, custom middleware can be created to handle incoming and outgoing requests/responses and handle errors consistently.
Practical Code Example
The following code snippet shows how to use custom error handling middleware to handle API errors:
<?php use Slim\Slim; use Slim\Middleware\ErrorMiddleware; $app = new Slim(); // 创建自定义的错误处理中间件 $errorMiddleware = function (Request $request, Response $response, $next) { try { // 调用下一个中间件 $response = $next($request, $response); } catch (Exception $e) { // 在这里处理错误 $response = $response->withStatus(500) ->withJson(array( 'error' => $e->getMessage() )); } return $response; }; // 将中间件添加到应用程序 $app->add($errorMiddleware);
In the above example, the error handling middleware will catch any exception thrown in the application and return a JSON response with the error code and message.
The above is the detailed content of PHP Web service development and API design error handling. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


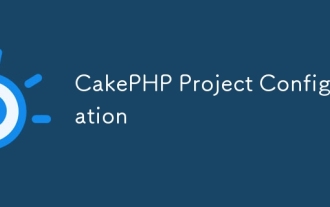
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
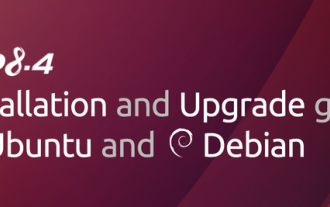
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
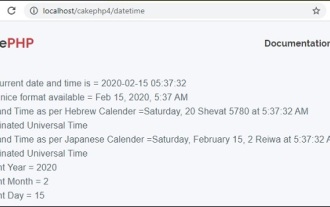
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
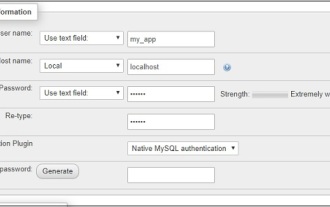
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
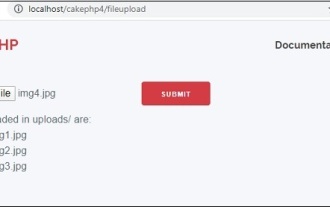
To work on file upload we are going to use the form helper. Here, is an example for file upload.
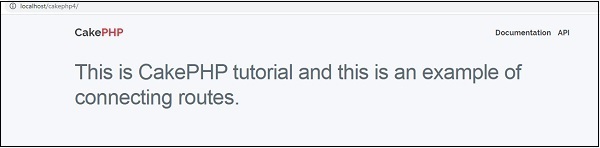
In this chapter, we are going to learn the following topics related to routing ?
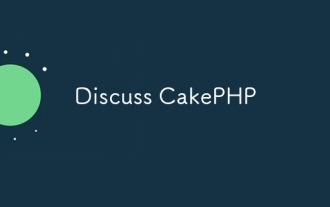
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
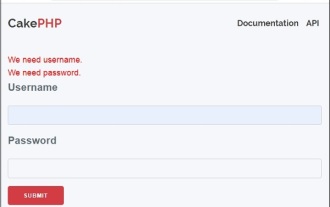
Validator can be created by adding the following two lines in the controller.
