


What are the concurrent programming frameworks and libraries in C++? What are their respective advantages and limitations?
C concurrent programming framework has the following options: lightweight threads (std::thread); thread-safe Boost concurrent containers and algorithms; OpenMP for shared memory multiprocessors; high-performance Thread Building Blocks (TBB); Cross-platform C concurrency interop library (cpp-Concur).
Concurrent Programming Frameworks and Libraries in C: Detailed Explanation and Comparison
Concurrent programming is essential for modern applications, allowing code to run on multiple threads or processes running simultaneously to improve performance and responsiveness. C provides a range of concurrent programming frameworks and libraries, each with its own unique advantages and limitations.
1. Thread (std::thread)
Thread is a lightweight concurrency mechanism provided in the C standard library. It allows you to execute code in a separate thread without using a higher level framework.
Advantages: Lightweight, easy to use, low overhead.
Limitations: Managing threads and synchronization operations is cumbersome and requires manual maintenance of thread life cycles and synchronization mechanisms.
2. Boost concurrent containers and algorithms
The Boost library provides a series of concurrent containers and algorithms, such as std::list, std::map and std:: Concurrent version of sort. These containers and algorithms use locking mechanisms to achieve thread safety, allowing multiple threads to access shared data structures simultaneously.
Advantages: Thread-safe and easy to use.
Limitations: May have additional overhead and may not be suitable for highly concurrent applications.
3. OpenMP
OpenMP is an API for shared memory multi-processor systems. It allows you to specify parallel regions in your code using pragma directives, and the compiler converts these regions into parallel code at compile time.
Advantages: Easy to use, suitable for computationally intensive applications, parallelism can be optimized by the compiler.
Limitations: Only available on compilers and platforms that support OpenMP, may be difficult to debug.
4. TBB (Thread Building Block)
TBB is a high-performance concurrency framework developed by Intel. It provides a set of primitives and abstractions designed to simplify parallel programming. TBB uses task decomposition, work-stealing scheduling, and cache locality optimization to achieve high performance.
Advantages: High performance, good scalability, and easy to use.
Limitations: Platform and compiler dependent, additional tuning may be required.
5. C Concurrency Interop Library (cpp-Concur)
cpp-Concur is a cross-platform concurrency framework developed by Microsoft. It provides a series of primitives for task scheduling, synchronization and communication, achieving cross-platform compatibility on different platforms and compilers.
Advantages: Cross-platform, flexible and easy to use.
Limitations: May have higher overhead than other frameworks, documentation may not be as comprehensive as other frameworks.
Practical case:
The following is a simple example of using Boost concurrent container:
#include <boost/thread/shared_mutex.hpp> #include <boost/thread.hpp> using namespace std; using namespace boost; shared_mutex mtx; unordered_map<int, string> shared_data; void writer_thread() { unique_lock<shared_mutex> lock(mtx); shared_data[1] = "foo"; } void reader_thread() { shared_lock<shared_mutex> lock(mtx); cout << shared_data[1] << endl; } int main() { boost::thread writer(writer_thread); boost::thread reader(reader_thread); writer.join(); reader.join(); return 0; }
In this example, we use shared_mutex
To protect shared data and allow concurrent read and write operations.
The above is the detailed content of What are the concurrent programming frameworks and libraries in C++? What are their respective advantages and limitations?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


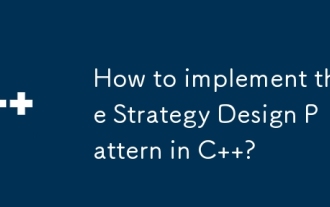
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
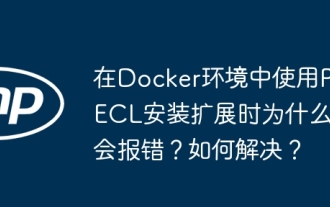
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
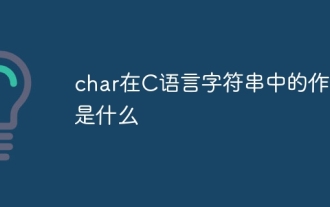
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
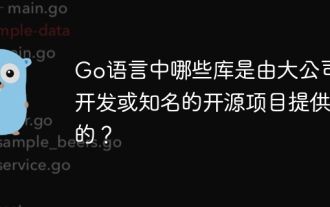
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
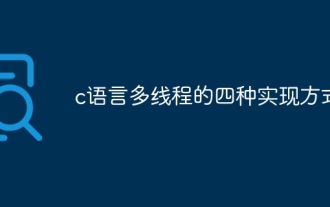
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
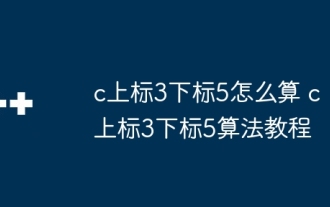
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
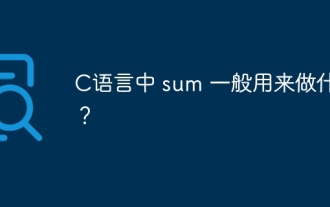
There is no function named "sum" in the C language standard library. "sum" is usually defined by programmers or provided in specific libraries, and its functionality depends on the specific implementation. Common scenarios are summing for arrays, and can also be used in other data structures, such as linked lists. In addition, "sum" is also used in fields such as image processing and statistical analysis. An excellent "sum" function should have good readability, robustness and efficiency.
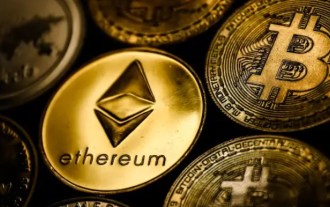
The Merge is a complex technological upgrade that transforms Ethereum's consensus mechanism from Proof of Work (PoW) to Proof of Stake (PoS). This involves multiple key aspects: first, the consensus layer is transformed into a PoS system managed by beacon chain, and the verifier needs to pledge Ether to participate in the consensus; second, the execution layer has been upgraded to be compatible with the PoS consensus layer to ensure transaction execution efficiency and accuracy; again, the new data processing and synchronization mechanism ensures the consistency of network nodes for the blockchain state; in addition, the difficulty bomb mechanism promotes the end of PoW mining; finally, smart contracts and decentralized applications have also undergone compatibility adjustments to ensure a smooth transition.
