


Confusion for Java Beginners: Challenges and Opportunities of Concurrent Programming
Concurrent programming presents challenges for Java beginners, such as data races and deadlocks. But it also brings opportunities to improve performance, taking advantage of multi-core processor power by executing tasks in parallel. In the actual case, multi-threaded file processing demonstrates the practical application of concurrency. A large number of files are processed in parallel through the thread pool, which greatly speeds up the processing. Concurrent programming presents both a challenge and an opportunity for Java developers to develop efficient and scalable applications.
Confusion for Java Beginners: Challenges and Opportunities of Concurrent Programming
Concurrent programming, that is, executing multiple tasks at the same time, for Java is both exciting and challenging for beginners. By adding real-life examples, this article will delve into the complexities of concurrent programming and reveal its career opportunities as a developer.
Challenge: Understanding Concurrency
Concurrent programming is very different from sequential programming, which can cause confusion for beginners. When multiple threads access shared resources at the same time, problems such as data competition and deadlock may occur. Understanding these challenges is critical to managing concurrency effectively.
Opportunity: Improving Performance
One of the main advantages of concurrent programming is that it can significantly improve performance. By executing tasks in parallel, programs can take advantage of the power of multi-core processors. This is especially effective when working with large amounts of data or resource-intensive tasks.
Practical Case: Multi-Threaded File Processing
To show concurrency in action, let us consider an example of multi-threaded file processing. Suppose we have 100 large files and need to perform complex operations on each file.
//顺序处理 for (File file : files) { // 处理文件 } //多线程处理 ExecutorService executor = Executors.newFixedThreadPool(10); for (File file : files) { executor.submit(() -> { // 处理文件 }); } executor.shutdown(); executor.awaitTermination(Long.MAX_VALUE, TimeUnit.SECONDS);
In sequential processing, files are processed sequentially. In multi-threaded processing, a thread pool is created and then one thread is submitted for each file. These threads run in parallel, significantly speeding up processing.
Conclusion
Concurrent programming is a fascinating field that presents challenges and opportunities for beginners. By understanding concurrency and leveraging its performance benefits, Java developers can develop efficient and scalable applications.
The above is the detailed content of Confusion for Java Beginners: Challenges and Opportunities of Concurrent Programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
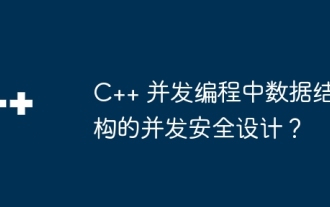
In C++ concurrent programming, the concurrency-safe design of data structures is crucial: Critical section: Use a mutex lock to create a code block that allows only one thread to execute at the same time. Read-write lock: allows multiple threads to read at the same time, but only one thread to write at the same time. Lock-free data structures: Use atomic operations to achieve concurrency safety without locks. Practical case: Thread-safe queue: Use critical sections to protect queue operations and achieve thread safety.
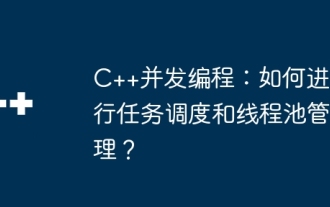
Task scheduling and thread pool management are the keys to improving efficiency and scalability in C++ concurrent programming. Task scheduling: Use std::thread to create new threads. Use the join() method to join the thread. Thread pool management: Create a ThreadPool object and specify the number of threads. Use the add_task() method to add tasks. Call the join() or stop() method to close the thread pool.
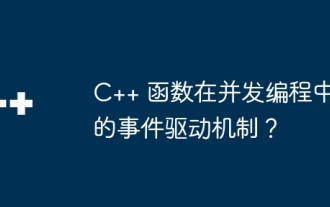
The event-driven mechanism in concurrent programming responds to external events by executing callback functions when events occur. In C++, the event-driven mechanism can be implemented with function pointers: function pointers can register callback functions to be executed when events occur. Lambda expressions can also implement event callbacks, allowing the creation of anonymous function objects. The actual case uses function pointers to implement GUI button click events, calling the callback function and printing messages when the event occurs.

To avoid thread starvation, you can use fair locks to ensure fair allocation of resources, or set thread priorities. To solve priority inversion, you can use priority inheritance, which temporarily increases the priority of the thread holding the resource; or use lock promotion, which increases the priority of the thread that needs the resource.
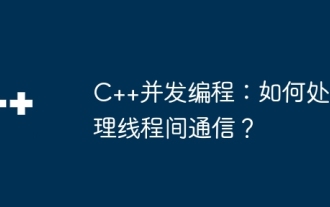
Methods for inter-thread communication in C++ include: shared memory, synchronization mechanisms (mutex locks, condition variables), pipes, and message queues. For example, use a mutex lock to protect a shared counter: declare a mutex lock (m) and a shared variable (counter); each thread updates the counter by locking (lock_guard); ensure that only one thread updates the counter at a time to prevent race conditions.
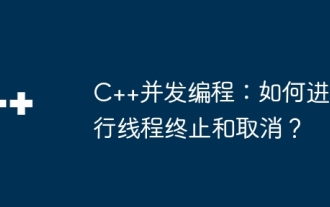
Thread termination and cancellation mechanisms in C++ include: Thread termination: std::thread::join() blocks the current thread until the target thread completes execution; std::thread::detach() detaches the target thread from thread management. Thread cancellation: std::thread::request_termination() requests the target thread to terminate execution; std::thread::get_id() obtains the target thread ID and can be used with std::terminate() to immediately terminate the target thread. In actual combat, request_termination() allows the thread to decide the timing of termination, and join() ensures that on the main line
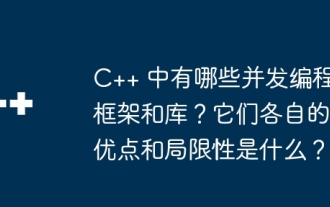
The C++ concurrent programming framework features the following options: lightweight threads (std::thread); thread-safe Boost concurrency containers and algorithms; OpenMP for shared memory multiprocessors; high-performance ThreadBuildingBlocks (TBB); cross-platform C++ concurrency interaction Operation library (cpp-Concur).
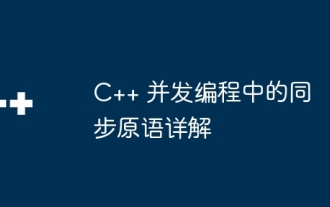
In C++ multi-threaded programming, the role of synchronization primitives is to ensure the correctness of multiple threads accessing shared resources. It includes: Mutex (Mutex): protects shared resources and prevents simultaneous access; Condition variable (ConditionVariable): thread Wait for specific conditions to be met before continuing execution; atomic operation: ensure that the operation is executed in an uninterruptible manner.
