Practice of PHP design patterns in large projects
The practice of design patterns is crucial in large-scale PHP projects. This article introduces several common patterns and their practical cases: Singleton pattern: ensures that only objects of a specific class are instantiated for managing global resources. Observer pattern: Allows objects to subscribe to events to receive notifications when events occur, enabling complex event processing. Factory method pattern: Provides a way to create objects without specifying a specific class, and objects can be created dynamically as needed. Strategy pattern: Allows dynamic changes to algorithms or behaviors without modifying client code, enabling interchangeable business rules or strategies.
Practice of PHP design patterns in large projects
In large software projects, design patterns are a vital tool , which helps developers create maintainable, extensible and reusable code. This article will introduce several commonly used design patterns and demonstrate their application in large-scale PHP projects through practical cases.
1. Singleton mode
The singleton mode ensures that only an object of a specific class is instantiated. This mode can be used to manage global resources such as database connections or caches.
Practical case: Database connection
<?php class DatabaseConnection { private static $instance = null; private function __construct() { /* ...数据库连接逻辑 ... */ } public static function getInstance() { if (self::$instance === null) { self::$instance = new self(); } return self::$instance; } }
2. Observer pattern
The observer pattern allows objects to subscribe to events so that Receive notifications when events occur. This pattern can be used to implement complex event handling systems.
Practical case: E-mail notification
<?php interface Observer { public function update(); } class EmailObserver implements Observer { public function update() { /* ...发送电子邮件通知 ... */ } } class Subject { private $observers = []; public function registerObserver(Observer $observer) { $this->observers[] = $observer; } public function notifyObservers() { foreach ($this->observers as $observer) { $observer->update(); } } }
3. Factory method pattern
The factory method pattern provides a created object way without specifying its concrete class. This pattern can be used to dynamically create objects based on need or configuration.
Practical case: Data source factory
<?php interface DataSourceInterface { public function connect(); public function fetch(); } class MySQLDataSource implements DataSourceInterface { // ...MySQL 数据源的实现 ... } class PostgreSQLDataSource implements DataSourceInterface { // ...PostgreSQL 数据源的实现 ... } class DataSourceFactory { public static function createDataSource($type) { switch ($type) { case 'mysql': return new MySQLDataSource(); case 'pgsql': return new PostgreSQLDataSource(); default: throw new Exception('Invalid data source type'); } } }
4. Strategy mode
The strategy mode allows dynamically changing algorithms or behaviors, No need to modify client code. This pattern can be used to implement interchangeable business rules or policies.
Practical case: Discount calculation
<?php interface DiscountStrategyInterface { public function calculateDiscount(float $amount); } class FixedDiscountStrategy implements DiscountStrategyInterface { private $discountAmount; public function __construct($discountAmount) { $this->discountAmount = $discountAmount; } public function calculateDiscount(float $amount) { return $amount - $this->discountAmount; } } class PercentageDiscountStrategy implements DiscountStrategyInterface { private $discountPercentage; public function __construct($discountPercentage) { $this->discountPercentage = $discountPercentage; } public function calculateDiscount(float $amount) { return $amount * (1 - $this->discountPercentage / 100); } }
The above is the detailed content of Practice of PHP design patterns in large projects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
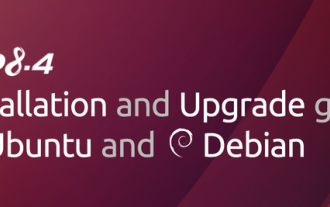
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
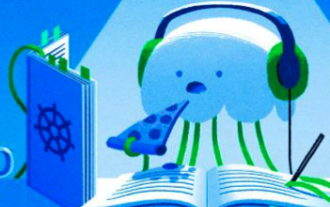
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c

One of the major changes introduced in MySQL 8.4 (the latest LTS release as of 2024) is that the "MySQL Native Password" plugin is no longer enabled by default. Further, MySQL 9.0 removes this plugin completely. This change affects PHP and other app
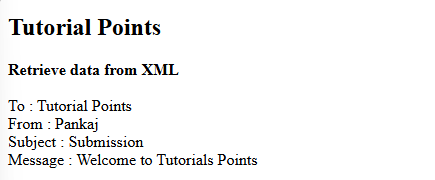
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
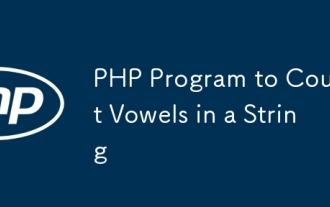
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
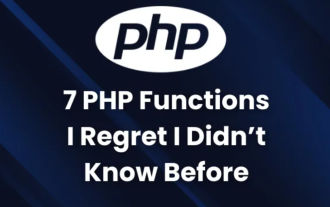
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
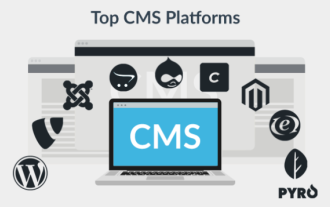
CMS stands for Content Management System. It is a software application or platform that enables users to create, manage, and modify digital content without requiring advanced technical knowledge. CMS allows users to easily create and organize content
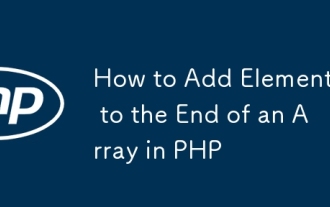
Arrays are linear data structures used to process data in programming. Sometimes when we are processing arrays we need to add new elements to the existing array. In this article, we will discuss several ways to add elements to the end of an array in PHP, with code examples, output, and time and space complexity analysis for each method. Here are the different ways to add elements to an array: Use square brackets [] In PHP, the way to add elements to the end of an array is to use square brackets []. This syntax only works in cases where we want to add only a single element. The following is the syntax: $array[] = value; Example
