Java Database Operations: Troubleshooting and Performance Tuning
Common problems in Java database operations include: database connection pool issues, SQLException exceptions, slow queries, deadlocks, and excessive connections. The solutions are: check the connection pool configuration, check exception messages, optimize queries and use indexes, reconstruct SQL statements involving deadlocks, and limit the number of open connections. Performance tuning tips include: batch operations, using cache, optimizing indexes, and correctly configuring connection pools.
Java Database Operations: Troubleshooting and Performance Tuning
Database operations are common tasks in Java applications. However, developers may encounter various issues when dealing with database connections, queries, and updates. This article discusses common problems and their solutions, and provides performance tuning tips to maximize the efficiency of database operations.
Common problems and solutions
1. Database connection pool problem
Problem: The application cannot establish a connection or connection to the database The pool is exhausted.
Solution:
- Ensure that the connection pool is properly configured, including the maximum number of connections and idle connection timeout.
- Use a connection pool management library, such as HikariCP or BoneCP, which can automatically create and destroy connections as needed.
2. SQLException
Problem: Database operation throws SQLException exception.
Workaround:
- Check the exception message carefully as it provides details about the source of the error.
- Use exception handling to handle common error scenarios, such as connection loss or constraint violations.
3. Slow query
Problem: The database query executes very slowly.
Solution:
- Use the explain/analyze statement to identify inefficient parts of the query execution plan.
- Create indexes to speed up searches on commonly used columns.
- Optimize query conditions carefully and avoid using OR and IN statements.
4. Deadlock
Problem: Multiple transactions hold locks on the same records at the same time, causing application deadlock.
Solution:
- Identify the SQL statements involved in deadlocks and restructure them to avoid simultaneous access to the same data.
- Implement transaction retry mechanism to automatically handle deadlock errors.
5. Over-connection
Problem: The application establishes too many connections to the database, thereby exhausting server resources.
Solution:
- Ensure that all database connections are properly closed after use.
- Use connection pooling to limit the number of connections open at the same time.
Performance tuning tips
1. Batch operations
Combining multiple database operations into one batch can significantly Improve performance.
// Batch insert using JDBC PreparedStatement try (PreparedStatement stmt = conn.prepareStatement("INSERT INTO employees (name, age) VALUES (?, ?)")) { stmt.setString(1, "John Doe"); stmt.setInt(2, 30); stmt.addBatch(); stmt.setString(1, "Jane Smith"); stmt.setInt(2, 25); stmt.addBatch(); int[] updateCounts = stmt.executeBatch(); } catch (SQLException e) { // Handle exceptions }
2. Use caching
Storing frequently queried data in the cache can reduce database access and improve performance.
import com.google.common.cache.CacheBuilder; import com.google.common.cache.CacheLoader; import com.google.common.cache.LoadingCache; // Cache employee objects by ID LoadingCache<Long, Employee> employeeCache = CacheBuilder.newBuilder() .maximumSize(1000) .build(new CacheLoader<Long, Employee>() { @Override public Employee load(Long id) { // Load employee from database return new Employee(id); } });
3. Index Optimization
Ensuring that the index is set up correctly is crucial for fast searches.
// Create index on employee name column try (Statement stmt = conn.createStatement()) { stmt.execute("CREATE INDEX idx_employee_name ON employees (name)"); }
4. Connection pool configuration
Properly configuring the connection pool can prevent excessive connections and connection leaks.
<!-- HikariCP configuration in application.properties --> spring.datasource.hikari.maximumPoolSize=10 spring.datasource.hikari.idleTimeout=600000
By employing these troubleshooting tips and performance tuning strategies, you can significantly optimize your Java database operations and improve your application's responsiveness and stability.
The above is the detailed content of Java Database Operations: Troubleshooting and Performance Tuning. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
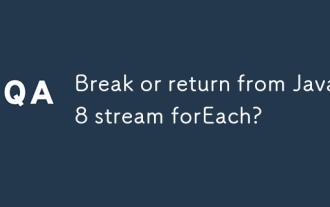
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
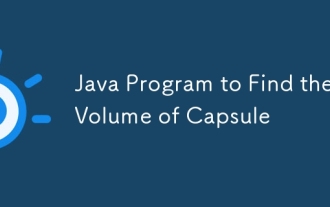
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
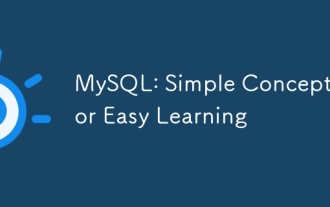
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
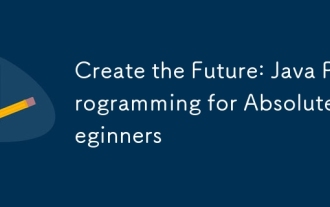
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
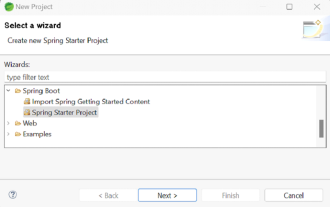
Spring Boot simplifies the creation of robust, scalable, and production-ready Java applications, revolutionizing Java development. Its "convention over configuration" approach, inherent to the Spring ecosystem, minimizes manual setup, allo
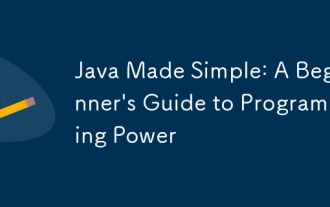
Java Made Simple: A Beginner's Guide to Programming Power Introduction Java is a powerful programming language used in everything from mobile applications to enterprise-level systems. For beginners, Java's syntax is simple and easy to understand, making it an ideal choice for learning programming. Basic Syntax Java uses a class-based object-oriented programming paradigm. Classes are templates that organize related data and behavior together. Here is a simple Java class example: publicclassPerson{privateStringname;privateintage;
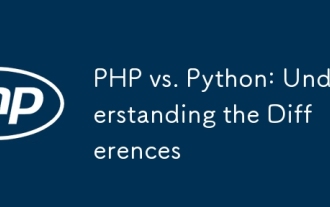
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
