How to express n raised to the nth power in c++
There are two ways to represent n to the power n in C: use the pow function, such as pow(5, 3) to represent 5 raised to the power 3, and the result is 125. Use operator overloading, such as Power(5) ^ 3 represents 5 raised to the third power, and the same result is 125.
In C, n raised to the nth power represents
C. Two methods are provided to express n raised to the nth power. :
1. pow function
#include <cmath> double pow(double x, int y);
x
:basey
:exponent
Example:
#include <iostream> #include <cmath> int main() { double base = 5; int exponent = 3; double result = pow(base, exponent); std::cout << base << " 的 " << exponent << " 次方为 " << result << std::endl; return 0; }
Run result:
<code>5 的 3 次方为 125</code>
2. Operator overloading
You can use operator<<
to overload operators and define your own operators to represent exponentiation operations.
Example:
#include <iostream> class Power { public: Power(double base) : base(base) {} double operator^(int exponent) { return pow(base, exponent); } private: double base; }; int main() { Power base(5); double result = base ^ 3; std::cout << 5 << " 的 " << 3 << " 次方为 " << result << std::endl; return 0; }
Run result:
<code>5 的 3 次方为 125</code>
The above is the detailed content of How to express n raised to the nth power in c++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
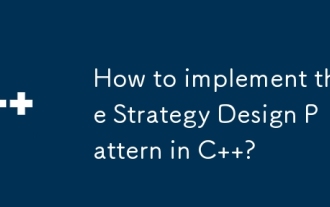
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
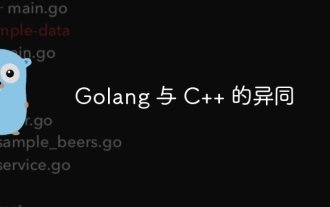
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
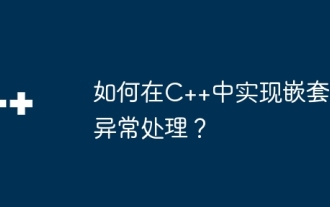
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
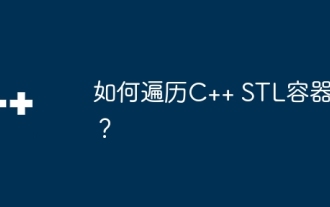
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
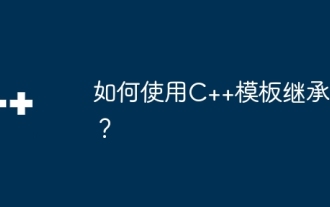
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
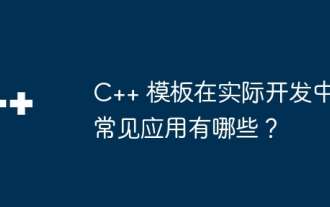
C++ templates are widely used in actual development, including container class templates, algorithm templates, generic function templates and metaprogramming templates. For example, a generic sorting algorithm can sort arrays of different types of data.
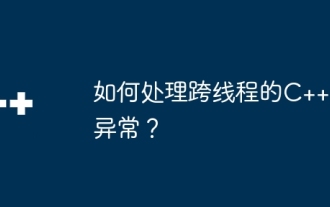
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
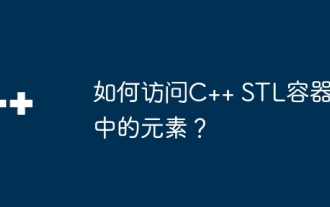
How to access elements in C++ STL container? There are several ways to do this: Traverse a container: Use an iterator Range-based for loop to access specific elements: Use an index (subscript operator []) Use a key (std::map or std::unordered_map)
