


What are the common patterns for implementing distributed systems with Golang?
When building a distributed system, it is crucial to follow common patterns: Distributed Consistency: The Raft consensus algorithm is used to ensure node consistency. Load Balancing: Hash rings evenly distribute requests to groups of servers. Message Queuing: Apache Kafka for reliable and scalable event streaming. Distributed lock: Redis distributed lock enables exclusive access across nodes. Distributed transactions: Two-phase commit coordinates multi-participant atomic transaction processing. Distributed cache: Memcached can store high-performance key-value data.
Use Golang to implement common patterns of distributed systems
When building distributed systems, understand and apply common patterns to It's important. Using Golang, we can easily implement these patterns by leveraging its concurrency and parallelism features.
1. Distributed consistency
- Raft consensus algorithm: Ensures that nodes in the cluster reach consensus, even if there is a network partition.
- Example: Using etcd storage system configuration
import ( "github.com/etcd-io/etcd/clientv3" ) func main() { client, err := clientv3.New(clientv3.Config{ Endpoints: []string{"localhost:2379"}, }) if err != nil { // Handle error } defer client.Close() }
2. Load balancing
- Hash ring: Evenly distribute requests among server groups.
- Example: Using consul service discovery and load balancing
import ( "github.com/hashicorp/consul/api" ) func main() { client, err := api.NewClient(api.DefaultConfig()) if err != nil { // Handle error } // ... Register and discover services using the client }
3. Message queue
- Apache Kafka: Distributed messaging platform for reliable and scalable event streaming.
- Example: Use sarama client library to connect to Kafka cluster
import ( "github.com/Shopify/sarama" ) func main() { config := sarama.NewConfig() client, err := sarama.NewClient([]string{"localhost:9092"}, config) if err != nil { // Handle error } defer client.Close() // ... Produce and consume messages using the client }
4. Distributed lock
- Redis distributed lock: Use the atomicity feature of Redis to achieve exclusive access across nodes.
- Example: Use the redisgo library to acquire and release distributed locks
import ( "github.com/go-redis/redis/v8" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", }) defer client.Close() // ... Acquire and release lock using the client }
5. Distributed transactions
- Two-Phase Commit (2PC): Coordinates multiple participants for atomic transaction processing.
- Example: Use go-tx library to implement 2PC
import ( "github.com/guregu/go-tx" ) func main() { db := tx.New(tx.Config{ Driver: "postgres", }) db.AutoCommit = false // ... Execute the two-phase commit }
6. Distributed cache
- Memcached: Distributed memory cache, used to store high-performance key-value data.
- Example: Use the go-memcached library to connect to the Memcached server
import ( "github.com/bradfitz/gomemcache/memcache" ) func main() { client := memcache.New("localhost:11211") // ... Set and get cache values using the client }
The above is the detailed content of What are the common patterns for implementing distributed systems with Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


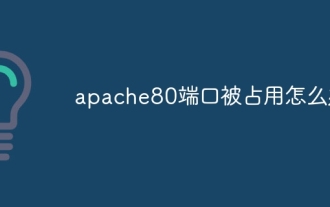
When the Apache 80 port is occupied, the solution is as follows: find out the process that occupies the port and close it. Check the firewall settings to make sure Apache is not blocked. If the above method does not work, please reconfigure Apache to use a different port. Restart the Apache service.
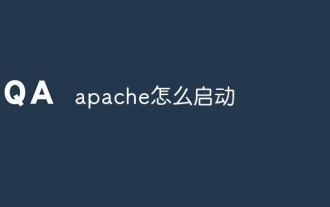
The steps to start Apache are as follows: Install Apache (command: sudo apt-get install apache2 or download it from the official website) Start Apache (Linux: sudo systemctl start apache2; Windows: Right-click the "Apache2.4" service and select "Start") Check whether it has been started (Linux: sudo systemctl status apache2; Windows: Check the status of the "Apache2.4" service in the service manager) Enable boot automatically (optional, Linux: sudo systemctl
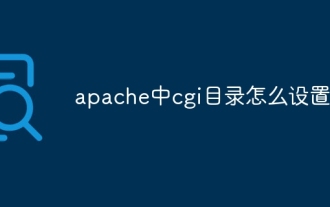
To set up a CGI directory in Apache, you need to perform the following steps: Create a CGI directory such as "cgi-bin", and grant Apache write permissions. Add the "ScriptAlias" directive block in the Apache configuration file to map the CGI directory to the "/cgi-bin" URL. Restart Apache.
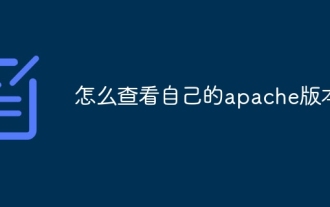
There are 3 ways to view the version on the Apache server: via the command line (apachectl -v or apache2ctl -v), check the server status page (http://<server IP or domain name>/server-status), or view the Apache configuration file (ServerVersion: Apache/<version number>).
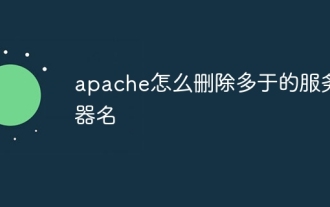
To delete an extra ServerName directive from Apache, you can take the following steps: Identify and delete the extra ServerName directive. Restart Apache to make the changes take effect. Check the configuration file to verify changes. Test the server to make sure the problem is resolved.
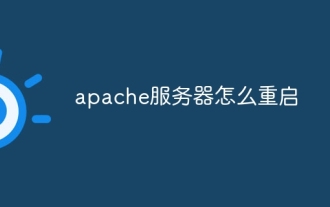
To restart the Apache server, follow these steps: Linux/macOS: Run sudo systemctl restart apache2. Windows: Run net stop Apache2.4 and then net start Apache2.4. Run netstat -a | findstr 80 to check the server status.
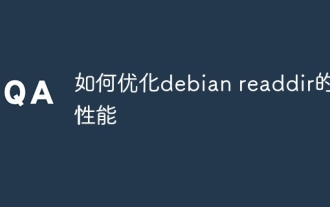
In Debian systems, readdir system calls are used to read directory contents. If its performance is not good, try the following optimization strategy: Simplify the number of directory files: Split large directories into multiple small directories as much as possible, reducing the number of items processed per readdir call. Enable directory content caching: build a cache mechanism, update the cache regularly or when directory content changes, and reduce frequent calls to readdir. Memory caches (such as Memcached or Redis) or local caches (such as files or databases) can be considered. Adopt efficient data structure: If you implement directory traversal by yourself, select more efficient data structures (such as hash tables instead of linear search) to store and access directory information
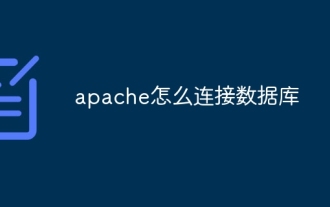
Apache connects to a database requires the following steps: Install the database driver. Configure the web.xml file to create a connection pool. Create a JDBC data source and specify the connection settings. Use the JDBC API to access the database from Java code, including getting connections, creating statements, binding parameters, executing queries or updates, and processing results.
