


Introduction to Java Basics to Practical Applications: Object-Oriented Practical Design
Object-oriented programming (OOP) is a programming paradigm that introduces objects and classes into code to facilitate the development of large software systems. This article introduces the practical application of object-oriented design through a bank account system case: Define classes and objects: Divide system functions into classes and objects, for example, the BankAccount class represents a bank account. Create and use objects: Create a BankAccount object and operate it using methods such as deposit, withdrawal, and balance query. Modularity and maintainability: By separating data and operations into classes and objects, the scalability, reusability and ease of maintenance of the code are achieved.
Java Basics to Practical Application: Object-Oriented Practical Design
Object-oriented programming (OOP) is a programming paradigm. It introduces the concepts of objects and classes into code, making it easier to develop and maintain large software systems. This article will provide a practical case to help you understand the basics of object-oriented design.
Case: Design a bank account system
Consider a bank account system that contains the following main functions:
- Create an account
- Deposit
- Withdrawal
- Check balance
Design classes and objects
The first step is to define Classes and objects in the system. A bank account can be represented as a class, which contains account information and operation methods.
public class BankAccount { private int accountNumber; private double balance; // Constructor public BankAccount(int accountNumber, double balance) { this.accountNumber = accountNumber; this.balance = balance; } // Methods public void deposit(double amount) { balance += amount; } public void withdraw(double amount) { if (amount <= balance) { balance -= amount; } } public double getBalance() { return balance; } }
Creating and using objects
Next, we can create BankAccount
objects and perform operations with them.
// 创建一个账户对象 BankAccount account = new BankAccount(123456789, 1000.0); // 存入 500 元 account.deposit(500.0); // 取款 300 元 account.withdraw(300.0); // 查询余额 double balance = account.getBalance(); System.out.println("账户余额:" + balance);
Output results:
账户余额:1200.0
By using classes and objects to separate data and operations in the code, we create a modular and easy-to-maintain bank account system.
Conclusion
Object-oriented design is a powerful method for building complex systems in Java. By understanding the concepts of classes, objects, and methods, you can design code that is extensible, reusable, and easy to understand.
The above is the detailed content of Introduction to Java Basics to Practical Applications: Object-Oriented Practical Design. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
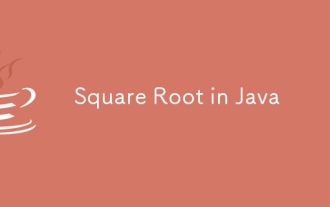
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
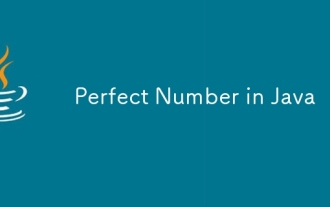
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
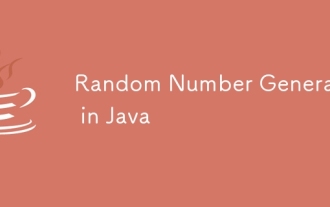
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
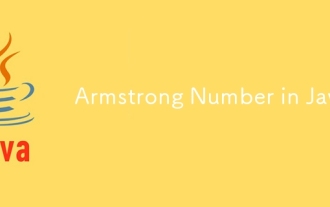
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
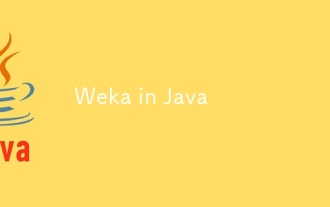
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
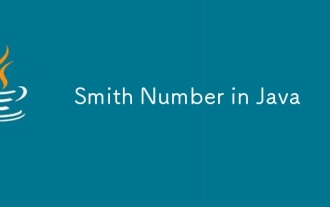
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
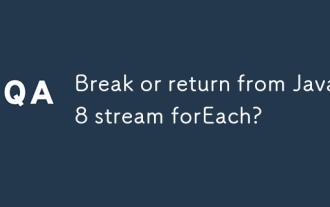
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
