Confusion for Java Beginners: A Deep Study of JVM Memory Management
Answer: JVM memory management uses a mark-and-clear algorithm to recycle objects that are no longer used, and is divided into heap, stack, method area, local method stack and program counter. Detailed description: JVM memory is divided into heap, stack, method area, local method stack and program counter. The mark-sweep GC algorithm marks reachable objects and frees memory for unmarked objects. Practical cases show that GC can release objects that are no longer used and reduce memory usage. Best practices include minimizing object creation, avoiding large object allocations, using weak and soft references, manually triggering GC, monitoring memory usage, and tuning JVM parameters.
Java Beginner’s Confusion: A Deep Study of JVM Memory Management
Introduction
Java Virtual Machine (JVM) memory management is a complex concept, but it is crucial to understanding the performance of Java programs. This article will delve into JVM memory management and illustrate it through practical cases.
JVM memory structure
JVM memory is divided into multiple areas, each area has a specific purpose:
- Heap: Storage dynamically allocated objects
- Stack:Storage local variables and object references used when calling methods
- Method area :Storage class metadata and code
- Native method stack:Storage implementation of native method
- Program counter:Track the current process Methods of the executing thread
Garbage Collection
The JVM will automatically perform garbage collection (GC) to recycle objects that are no longer used to release memory. The main algorithm of GC is the mark-sweep algorithm:
- Mark: Mark all reachable objects (can be accessed through the root object)
- Clear: Release the memory of unmarked objects
Memory management practical case
The following code creates an array containing 1000 integer arrays, and then passes GC manually triggers recycling:
import java.util.ArrayList; import java.util.Arrays; public class MemoryManagementExample { public static void main(String[] args) { // 创建包含 1000 个数组的 ArrayList ArrayList<int[]> list = new ArrayList<>(); for (int i = 0; i < 1000; i++) { list.add(new int[1000]); } // 强制触发 GC System.gc(); // 打印 GC 后内存使用情况 Runtime runtime = Runtime.getRuntime(); System.out.println("内存使用情况:"); System.out.println("已使用内存:" + runtime.totalMemory() - runtime.freeMemory()); System.out.println("可用内存:" + runtime.freeMemory()); } }
Output
内存使用情况: 已使用内存:803968 可用内存:0
In this example, the GC successfully freed the integers in the array that are no longer used, resulting in a significant reduction in memory usage.
Best Practices
The following are some best practices for JVM memory management:
- Minimize object creation
- Avoid large object allocation
- Consider using weak references and soft references
- Manually trigger GC regularly
- Monitor memory usage and adjust JVM parameters as needed
Conclusion
Understanding JVM memory management is the foundation of Java programming. By mastering the mark-sweep GC algorithm and best practices, you can optimize the performance and memory utilization of your Java programs.
The above is the detailed content of Confusion for Java Beginners: A Deep Study of JVM Memory Management. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
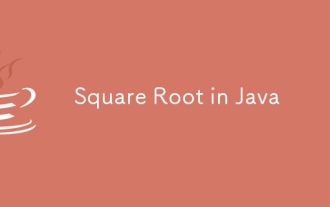
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
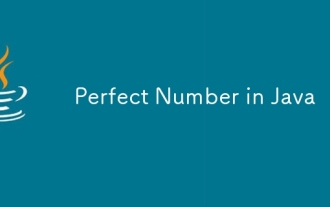
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
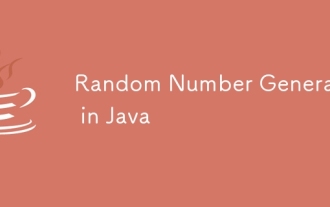
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
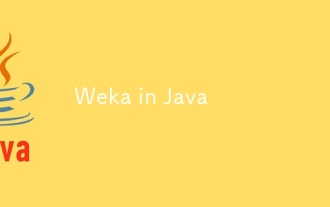
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
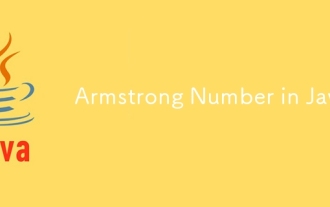
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
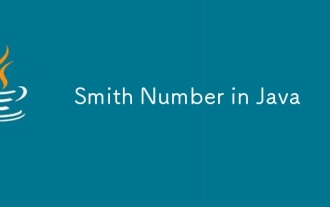
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
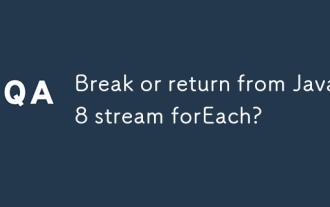
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
